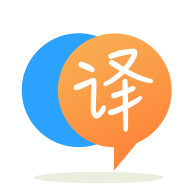
[英]Can i use the bfs technique for finding the shortest path in an undirected weighted graph? Instead of dijkstra's algo?
[英]How to implement Dijkstra Algorithm for finding shortest path from 1 node to all other in an undirected graph in C and print the distances as well
有一個無向圖。 您需要將所有邊權重存儲在一個二維數組cost[][]中,並計算從源節點0到所有其他節點的最短距離。 假設最多有 100 個節點。 如果兩個節點之間沒有邊,我們將它們的權重設置為一個很大的數,MAX_DIS=999999,表示這兩個節點沒有直接連接。
在本練習中,您需要完成以下兩項任務。
初始化成本數組 最初,我們使用數組 cost[100][100] 來存儲邊成本。 我們輸入總節點數 n 和邊數 m,並以 <x,y,w> 格式輸入所有邊,其中 w 是邊 (x,y) 的權重。 如果兩個節點之間沒有邊,我們設置成本 MAX_DIS。
計算最短距離。 使用成本數組,我們需要計算節點 0 和所有其他節點之間的最短距離。 另外,我們首先需要初始化距離數組distance[100]。 然后在每個循環中,我們首先找到最小距離 distance[w],如果節點 v 與 w 相鄰,則更新其他距離 distance[v]。
//下面是我的這個挑戰的代碼,但它不適用於所有測試用例。 它對某些人來說很好,但我不知道問題出在哪里。 我希望這是一個需要解決的很好的挑戰,這就是我在這里發布它的原因。 你們能幫我調試一下這段代碼嗎...
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_NODES 100
#define MAX_DIS 999999
int cost[MAX_NODES][MAX_NODES];
int distance[MAX_NODES];
void initial(int m, int n);
void Dijkstra(int n);
void initial(int m, int n)
{
/*
let user input all edges and their weights and initialize cost[][].
note that if no edge between (x,y), set cost[x][y]=MAX_DIS
and cost[a][b]=cost[b][a] for the undirected graph.
Fill in your code here...
*/
int i,j;
for(i=0;i<n;i++){
for(j=0;j<n;j++){
cost[i][j] = MAX_DIS;
}
}
cost[0][0] = 0;
int weight,x,y;
for(i=0; i < m; i++){
scanf("%d %d %d", &x,&y,&weight);
cost[x][y] = weight;
cost[y][x] = weight;
}
}
void Dijsktra(int n)
{
/*
Fill in your code here...
calculate the distance from node 0 to all other nodes.
*/
int i;
int S[n];
S[0] = 1;
int all_visited = 0;
for(i=1;i<n;i++){
S[i] = -1;
}
for(i=0;i<n;i++){
distance[i] = cost[0][i];
}
while(all_visited != 1){
int temp = MAX_DIS;
int pos = -1;
for(i=1;i<n;i++){
if(S[i] == -1 && cost[0][i] <= temp){
temp = cost[0][i];
pos = i;
}
}
S[pos] = 1;
for(i=0;i<n;i++){
if(S[i] == -1)
break;
}
if(i==n)
all_visited = 1;
for(i=1; i<n; i++){
distance[i] = (int)fmin(distance[i], distance[pos] + cost[pos][i]);
}
}
}
int main()
{
int m,n;
printf("Input the number of nodes:\n");
scanf("%d",&n);
printf("Input the number of edges:\n");
scanf("%d",&m);
printf("Input these edges:\n");
initial(m,n);
Dijsktra(n);
for(int i=0;i<n;i++)
printf("%d ",distance[i]);
return 0;
}
這是我的代碼失敗的測試用例 -
輸入節點數:8 輸入邊數:10 輸入這些邊:0 1 2, 1 2 9, 2 3 4, 3 5 7, 2 4 8, 5 6 10, 6 7 8, 7 5 1, 7 3 4, 0 4 10
預期 output - 0 2 11 15 10 20 27 19
我的 output - 0 2 11 15 10 999999 999999 999999
在此循環中使用break
語句。
for(i=1;i<n;i++){
if(S[i] == -1 && cost[0][i] <= temp){
temp = cost[0][i];
pos = i;
break; //here
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.