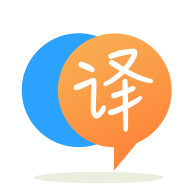
[英]Pandas: populating column values with date and time string based on conditions
[英]Create column based on date and time conditions
我有一個包含超過 10,000 條記錄的交通機構的每日智能卡交易數據集。 我正在嘗試創建一個名為transfer
的列,用於指示交易是否為轉移。 我創建了一個模擬數據集:
mock_df = pd.DataFrame({
'cardNumber': ['100', '100', '100', '200', '300', '300', '300'],
'type': ['DAILY_CAP_REACHED', 'DAILY_CAP_REACHED', 'DAILY_CAP_REACHED', 'DAILY_CAP_REACHED',
'DAILY_CAP_REACHED', 'DAILY_CAP_REACHED', 'DAILY_CAP_REACHED'],
'date_only': ['2021/05/01', '2021/05/01', '2021/05/01', '2021/05/01', '2021/05/04', '2021/05/04', '2021/05/04'],
'time_only': ['17:15', '17:45', '18:15', '12:15', '13:15', '17:45', '18:15'],
'fare': [250, 0, 0, 250, 250, 0, 0]
})
產生下表。
cardNumber type date_only time_only fare
0 100 DAILY_CAP_REACHED 2021/05/01 17:15 250
1 100 DAILY_CAP_REACHED 2021/05/01 17:45 0
2 100 DAILY_CAP_REACHED 2021/05/01 18:15 0
3 200 DAILY_CAP_REACHED 2021/05/01 12:15 250
4 300 DAILY_CAP_REACHED 2021/05/04 13:15 250
5 300 DAILY_CAP_REACHED 2021/05/04 17:45 0
6 300 DAILY_CAP_REACHED 2021/05/04 18:15 0
每天,乘客有 90 分鍾的時間從他們的第一筆交易中進行轉賬。 因此,如果自第一次交易以來已經過去了 90 分鍾以上,則不應將其記錄為轉賬。 我希望有一個如下所示的數據框:
cardNumber type date_only time_only fare transfer
0 100 DAILY_CAP_REACHED 2021/05/01 17:15 250 N
1 100 DAILY_CAP_REACHED 2021/05/01 17:45 0 Y
2 100 DAILY_CAP_REACHED 2021/05/01 18:15 0 Y
3 200 DAILY_CAP_REACHED 2021/05/01 12:15 250 N
4 300 DAILY_CAP_REACHED 2021/05/04 13:15 250 N
5 300 DAILY_CAP_REACHED 2021/05/04 17:45 0 N
6 300 DAILY_CAP_REACHED 2021/05/04 18:15 0 Y
您可以使用:
mock_df['datetime'] = pd.to_datetime(mock_df['date_only'] + ' ' + mock_df['time_only'])
mock_df['transfer'] = np.where(mock_df.groupby('cardNumber')['datetime'].diff() <= pd.Timedelta(minutes=90), 'Y', 'N')
結果:
print(mock_df)
cardNumber type date_only time_only fare datetime transfer
0 100 DAILY_CAP_REACHED 2021/05/01 17:15 250 2021-05-01 17:15:00 N
1 100 DAILY_CAP_REACHED 2021/05/01 17:45 0 2021-05-01 17:45:00 Y
2 100 DAILY_CAP_REACHED 2021/05/01 18:15 0 2021-05-01 18:15:00 Y
3 200 DAILY_CAP_REACHED 2021/05/01 12:15 250 2021-05-01 12:15:00 N
4 300 DAILY_CAP_REACHED 2021/05/04 13:15 250 2021-05-04 13:15:00 N
5 300 DAILY_CAP_REACHED 2021/05/04 17:45 0 2021-05-04 17:45:00 N
6 300 DAILY_CAP_REACHED 2021/05/04 18:15 0 2021-05-04 18:15:00 Y
我正在閱讀的關鍵部分是這個要求:
“如果自他們的第一筆交易以來已經過去了 90 分鍾以上,則不應將其記錄為轉移。”
因此,僅與前一次相比是不夠的,因為 3 筆交易中的每筆交易之間可能有不到 90 分鍾,但第一筆和最后一筆之間的時間可能超過 90 分鍾,從而使其成為非轉移。
這種方法會奏效,盡管可能有辦法讓它更漂亮一點:
mock_df['datetime'] = pd.to_datetime(mock_df['date_only'] + ' ' + mock_df['time_only'])
for grp_idx,grp in mock_df.groupby('cardNumber'):
for i,(idx,dt) in enumerate(grp['datetime'].iteritems()):
if i==0:
dt_comp = dt
mock_df.at[idx,'transfer'] = 'N'
else:
if dt-dt_comp < pd.Timedelta(minutes=90):
mock_df.at[idx,'transfer'] = 'Y'
else:
mock_df.at[idx,'transfer'] = 'N'
dt_comp = dt
編輯:添加結果
cardNumber type date_only time_only fare \
0 100 DAILY_CAP_REACHED 2021/05/01 17:15 250
1 100 DAILY_CAP_REACHED 2021/05/01 17:45 0
2 100 DAILY_CAP_REACHED 2021/05/01 18:15 0
3 200 DAILY_CAP_REACHED 2021/05/01 12:15 250
4 300 DAILY_CAP_REACHED 2021/05/04 13:15 250
5 300 DAILY_CAP_REACHED 2021/05/04 17:45 0
6 300 DAILY_CAP_REACHED 2021/05/04 18:15 0
datetime transfer
0 2021-05-01 17:15:00 N
1 2021-05-01 17:45:00 Y
2 2021-05-01 18:15:00 Y
3 2021-05-01 12:15:00 N
4 2021-05-04 13:15:00 N
5 2021-05-04 17:45:00 N
6 2021-05-04 18:15:00 Y
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.