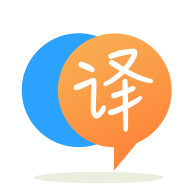
[英]How would I make my Python Morse code translator distinguish between singular dots and dashes and a sequence of them?
[英]How do I make my code differentiate between words and singular characters? (Python)
(Python)我的任務是創建一個程序,收集一個 input() 並將其放入字典中。 對於文本中的每個單詞,它都會計算它之前出現的次數。 我的代碼:
text = input()
words = {}
for word in text:
if word not in words:
words[word] = 0
print(words[word])
elif word in words:
words[word] = words[word] + 1
print(words[word])
示例輸入可以是:
one two one two three two four three
正確的 output 應該是:
0
0
1
1
0
2
0
1
然而,我的代碼計算每個字符的出現次數,而不是每個單詞的出現次數,這使得 output 太長了。 我如何讓它區分單詞和字符?
那是因為text
是一個字符串,遍歷字符串就是遍歷字符。 您可以for word in text.split()
,這會將字符串拆分為一個列表。 默認情況下,它會根據空格進行拆分,因此會在此處將其拆分為一個單詞列表。
鑒於您的示例輸入,您需要在空格上拆分text
才能獲取單詞。 通常,將任意文本拆分為單詞/標記的問題很重要; 有很多專門為此而構建的自然語言處理庫。
此外,對於計數,內置 collections 模塊中的Counter
class 非常有用。
from collections import Counter
text = input()
word_counts = Counter(w for w in text.split())
print(word_counts.most_common())
Output
[('two', 3), ('one', 2), ('three', 2), ('four', 1)]
您正在尋找 function 拆分自字符串類型: https://docs.python.org/3/library/stdtypes.html?highlight=str%20split#str.split
用它來創建一個單詞數組:
splitted_text = text.split()
完整示例如下所示:
text = 'this is an example and this is nice'
splitted_text = text.split()
words = {}
for word in splitted_text:
if word not in words:
words[word] = 0
elif word in words:
words[word] = words[word] + 1
print(words)
這將是 output:
{'this': 1, 'is': 1, 'an': 0, 'example': 0, 'and': 0, 'nice': 0}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.