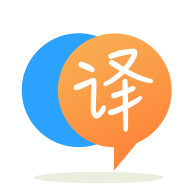
[英]How would I make my Python Morse code translator distinguish between singular dots and dashes and a sequence of them?
[英]How do I make my code differentiate between words and singular characters? (Python)
(Python)我的任务是创建一个程序,收集一个 input() 并将其放入字典中。 对于文本中的每个单词,它都会计算它之前出现的次数。 我的代码:
text = input()
words = {}
for word in text:
if word not in words:
words[word] = 0
print(words[word])
elif word in words:
words[word] = words[word] + 1
print(words[word])
示例输入可以是:
one two one two three two four three
正确的 output 应该是:
0
0
1
1
0
2
0
1
然而,我的代码计算每个字符的出现次数,而不是每个单词的出现次数,这使得 output 太长了。 我如何让它区分单词和字符?
那是因为text
是一个字符串,遍历字符串就是遍历字符。 您可以for word in text.split()
,这会将字符串拆分为一个列表。 默认情况下,它会根据空格进行拆分,因此会在此处将其拆分为一个单词列表。
鉴于您的示例输入,您需要在空格上拆分text
才能获取单词。 通常,将任意文本拆分为单词/标记的问题很重要; 有很多专门为此而构建的自然语言处理库。
此外,对于计数,内置 collections 模块中的Counter
class 非常有用。
from collections import Counter
text = input()
word_counts = Counter(w for w in text.split())
print(word_counts.most_common())
Output
[('two', 3), ('one', 2), ('three', 2), ('four', 1)]
您正在寻找 function 拆分自字符串类型: https://docs.python.org/3/library/stdtypes.html?highlight=str%20split#str.split
用它来创建一个单词数组:
splitted_text = text.split()
完整示例如下所示:
text = 'this is an example and this is nice'
splitted_text = text.split()
words = {}
for word in splitted_text:
if word not in words:
words[word] = 0
elif word in words:
words[word] = words[word] + 1
print(words)
这将是 output:
{'this': 1, 'is': 1, 'an': 0, 'example': 0, 'and': 0, 'nice': 0}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.