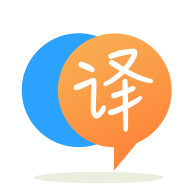
[英]This model has not yet been built. Build the model first by calling `build()` or by calling the model on a batch of data
[英]RNN Model Error: "ValueError: This model has not yet been built."
我正在使用 Google Colab 上的本教程構建基於字符的 LSTM-RNN 文本生成器: https ://colab.research.google.com/github/tensorflow/text/blob/master/docs/tutorials/text_generation.ipynb# scrollTo=d4tSNwymzf-q 。
雖然他們的代碼在我的 Google Colab 帳戶上使用他們的莎士比亞數據集運行和編譯,但當我輸入自己的數據集時它不起作用。 這個錯誤不斷出現:
"ValueError: This model has not yet been built.
他們使用的數據集是來自 Tensorflow ( https://storage.googleapis.com/download.tensorflow.org/data/shakespeare.txt ) 的莎士比亞文本。 另一方面,我的數據集是短字符的形式。 這是我的數據集的前五行(我正在嘗試生成肽序列):
acssspskhcg
acknffwktftsc
阿吉爾克
阿吉爾金卡拉阿拉基勒
aplepeypgdnatpeqmaqyaaelrryinmltrpry
貪婪
我想這可能是問題的一部分。
這是我到目前為止的代碼:
import tensorflow as tf
from tensorflow.keras.layers.experimental import preprocessing
import numpy as np
import os
import time
# Read, then decode for py2 compat.
text = open("/content/generatorinput.txt", 'rb').read().decode(encoding='utf-8')
# length of text is the number of characters in it
print(f'Length of text: {len(text)} characters')
# The unique characters in the file
vocab = sorted(set(text))
print(f'{len(vocab)} unique characters')
example_texts = ['acdefgh', 'tvy']
chars = tf.strings.unicode_split(example_texts, input_enco
chars
ids_from_chars = preprocessing.StringLookup(
vocabulary=list(vocab), mask_token=None)
ids = ids_from_chars(chars)
ids
chars_from_ids = tf.keras.layers.experimental.preprocessing.StringLookup(
vocabulary=ids_from_chars.get_vocabulary(), invert=True, mask_token=None)
chars = chars_from_ids(ids)
chars
tf.strings.reduce_join(chars, axis=-1).numpy()
def text_from_ids(ids):
return tf.strings.reduce_join(chars_from_ids(ids), axis=-1)
all_ids = ids_from_chars(tf.strings.unicode_split(text, 'UTF-8'))
all_ids
ids_dataset = tf.data.Dataset.from_tensor_slices(all_ids)
for ids in ids_dataset.take(10):
print(chars_from_ids(ids).numpy().decode('utf-8'))
seq_length = 100
examples_per_epoch = len(text)//(seq_length+1)
sequences = ids_dataset.batch(seq_length+1, drop_remainder=True)
for seq in sequences.take(1):
print(chars_from_ids(seq))
def split_input_target(sequence):
input_text = sequence[:-1]
target_text = sequence[1:]
return input_text, target_text
dataset = sequences.map(split_input_target)
for input_example, target_example in dataset.take(1):
print("Input :", text_from_ids(input_example).numpy())
print("Target:", text_from_ids(target_example).numpy())
# Batch size
BATCH_SIZE = 64
# Buffer size to shuffle the dataset
# (TF data is designed to work with possibly infinite sequences,
# so it doesn't attempt to shuffle the entire sequence in memory. Instead,
# it maintains a buffer in which it shuffles elements).
BUFFER_SIZE = 100
dataset = (
dataset
.shuffle(BUFFER_SIZE)
.batch(BATCH_SIZE, drop_remainder=True)
.prefetch(tf.data.experimental.AUTOTUNE))
dataset
# Length of the vocabulary in chars
vocab_size = len(vocab)
# The embedding dimension
embedding_dim = 256
# Number of RNN units
rnn_units = 1024
class MyModel(tf.keras.Model):
def __init__(self, vocab_size, embedding_dim, rnn_units):
super().__init__(self)
self.embedding = tf.keras.layers.Embedding(vocab_size, embedding_dim)
self.gru = tf.keras.layers.GRU(rnn_units,
return_sequences=True,
return_state=True)
self.dense = tf.keras.layers.Dense(vocab_size)
def call(self, inputs, states=None, return_state=False, training=False):
x = inputs
x = self.embedding(x, training=training)
if states is None:
states = self.gru.get_initial_state(x)
x, states = self.gru(x, initial_state=states, training=training)
x = self.dense(x, training=training)
if return_state:
return x, states
else:
return x
model = MyModel(
# Be sure the vocabulary size matches the `StringLookup` layers.
vocab_size=len(ids_from_chars.get_vocabulary()),
embedding_dim=embedding_dim,
rnn_units=rnn_units)
for input_example_batch, target_example_batch in dataset.take(1):
example_batch_predictions = model(input_example_batch)
print(example_batch_predictions.shape, "# (batch_size, sequence_length, vocab_size)")
model.summary() # <-- This is where the code stops working
我已經嘗試過:重新啟動我的運行時,更改我的緩沖區大小並定義輸入形狀。
當我定義輸入形狀並繼續執行代碼時,我得到以下信息:
sampled_indices = tf.random.categorical(example_batch_predictions[0], num_samples=1)
sampled_indices = tf.squeeze(sampled_indices, axis=-1).numpy()
ERROR: example_batch_predictions is not defined
無論哪種方式,我都會收到錯誤消息。 我該如何解決這個問題? 任何建議都深表感謝。
如果您嘗試將一些數據傳遞給您的模型,因為您正在嘗試使用此行: example_batch_predictions = model(input_example_batch)
(在您的 for 循環中),您的模型摘要將起作用,但請注意在您的循環中沒有打印任何內容。 問題是您正在使用example_texts
,其中包含兩個字符串,並且您仍在使用 64 的batch_size
和 100 的sequence_length
。如果您將batch_size
更改為 2 並將sequence_length
更改為 5,您應該會看到如下輸出:
Length of text: 100 characters
20 unique characters
a
c
s
s
s
p
s
k
h
c
tf.Tensor([b'a' b'c' b's'], shape=(3,), dtype=string)
Input : b'ac'
Target: b'cs'
(1, 2, 21) # (batch_size, sequence_length, vocab_size)
Model: "my_model_13"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
embedding_13 (Embedding) multiple 5376
_________________________________________________________________
gru_13 (GRU) multiple 3938304
_________________________________________________________________
dense_13 (Dense) multiple 21525
=================================================================
Total params: 3,965,205
Trainable params: 3,965,205
Non-trainable params: 0
_________________________________________________________________
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.