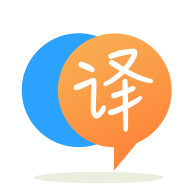
[英]Filter dataframe with dictionary values while assigning dictionary keys to matching rows?
[英]How to filter pandas dataframe rows based on dictionary keys and values?
我在 Python 中有一個數據框和一個字典,如下所示,我需要根據字典過濾數據框。 如您所見,字典的鍵和值是數據框的兩列。 我想要一個數據框的子集,其中包含字典的鍵和值以及其他列。
df:
顧客ID | 類別 | 類型 | 送貨 |
---|---|---|---|
40275 | 書 | 買 | 真的 |
40275 | 軟件 | 賣 | 錯誤的 |
40275 | 電子游戲 | 賣 | 錯誤的 |
40275 | 手機 | 賣 | 錯誤的 |
39900 | CD/DVD | 賣 | 真的 |
39900 | 書 | 買 | 真的 |
39900 | 軟件 | 賣 | 真的 |
35886 | 手機 | 賣 | 錯誤的 |
35886 | 電子游戲 | 買 | 錯誤的 |
35886 | CD/DVD | 賣 | 錯誤的 |
35886 | 軟件 | 賣 | 錯誤的 |
40350 | 軟件 | 賣 | 真的 |
28129 | 軟件 | 買 | 錯誤的 |
字典是:
d = {
40275: ['Book','Software'],
39900: ['Book'],
35886: ['Software'],
40350: ['Software'],
28129: ['Software']
}
我需要以下數據框:
顧客ID | 類別 | 類型 | 送貨 |
---|---|---|---|
40275 | 書 | 買 | 真的 |
40275 | 軟件 | 賣 | 錯誤的 |
39900 | 書 | 買 | 真的 |
35886 | 軟件 | 賣 | 錯誤的 |
40350 | 軟件 | 賣 | 真的 |
28129 | 軟件 | 買 | 錯誤的 |
我們可以set_index
到Customer_ID
和Category
列,然后從字典d
構建元組列表並reindex
DataFrame 以僅包含與元組列表匹配的行,然后reset_index
恢復列:
new_df = df.set_index(['Customer_ID', 'Category']).reindex(
[(k, v) for k, lst in d.items() for v in lst]
).reset_index()
new_df
:
Customer_ID Category Type Delivery
0 40275 Book Buy True
1 40275 Software Sell False
2 39900 Book Buy True
3 35886 Software Sell False
4 40350 Software Sell True
5 28129 Software Buy False
*請注意,這只適用於 MultiIndex 是唯一的(如所示示例)。 如果字典不代表 DataFrame 的 MultiIndex 的子集(這可能是也可能不是所需的行為),它也會添加行。
設置:
import pandas as pd
d = {
40275: ['Book', 'Software'],
39900: ['Book'],
35886: ['Software'],
40350: ['Software'],
28129: ['Software']
}
df = pd.DataFrame({
'Customer_ID': [40275, 40275, 40275, 40275, 39900, 39900, 39900, 35886,
35886, 35886, 35886, 40350, 28129],
'Category': ['Book', 'Software', 'Video Game', 'Cell Phone', 'CD/DVD',
'Book', 'Software', 'Cell Phone', 'Video Game', 'CD/DVD',
'Software', 'Software', 'Software'],
'Type': ['Buy', 'Sell', 'Sell', 'Sell', 'Sell', 'Buy', 'Sell', 'Sell',
'Buy', 'Sell', 'Sell', 'Sell', 'Buy'],
'Delivery': [True, False, False, False, True, True, True, False, False,
False, False, True, False]
})
您可以將df.merge
與df.append
df.merge
使用:
In [444]: df1 = pd.DataFrame.from_dict(d, orient='index', columns=['Cat1', 'Cat2']).reset_index()
In [449]: res = df.merge(df1[['index', 'Cat1']], left_on=['Customer_ID', 'Category'], right_on=['index', 'Cat1']).drop(['index', 'Cat1'], 1)
In [462]: res = res.append(df.merge(df1[['index', 'Cat2']], left_on=['Customer_ID', 'Category'], right_on=['index', 'Cat2']).drop(['index', 'Cat2'], 1)).sort_values('Customer_ID', ascending=False)
In [463]: res
Out[463]:
Customer_ID Category Type Delivery
3 40350 Software Sell True
0 40275 Book Buy True
0 40275 Software Sell False
1 39900 Book Buy True
2 35886 Software Sell False
4 28129 Software Buy False
展平字典並創建一個新的數據幀,然后將df
與新的數據幀進行內部合並
df.merge(pd.DataFrame([{'Customer_ID': k, 'Category': i}
for k, v in d.items() for i in v]))
Customer_ID Category Type Delivery
0 40275 Book Buy True
1 40275 Software Sell False
2 39900 Book Buy True
3 35886 Software Sell False
4 40350 Software Sell True
5 28129 Software Buy False
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.