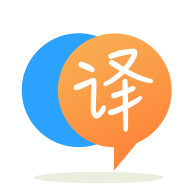
[英]How do I generate a Random.Range value once, and then use it in void Update to move? Unity, C#
[英]How to generate only one random value from a range c#
從一個范圍內只生成一個隨機值/int我遇到了一個問題,當我從一個范圍內生成一個隨機值/int 時,它會生成 10,而我只希望它生成一個。 我已經嘗試將生成隨機 int 的代碼放在 if 語句中,其中需要一個變量為 false 才能運行它,然后在 if 語句中將變量更改為 true,但這也不起作用。
random = Random.Range(0, 9);
上面的代碼行是我用來生成隨機整數的。 我還嘗試了另一種方法,我將在下面展示。
int buttonValue = Random.Range(minButtons, maxButtons);
//these are the variables being used in the code above
public int maxButtons = 9;
public int minButtons = 1;
int buttonValue;
作為參考,這是我的整個腳本。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ButtonSystem : MonoBehaviour
{
// buttons
GameObject button1;
GameObject button2;
GameObject button3;
GameObject button4;
GameObject button5;
GameObject button6;
GameObject button7;
GameObject button8;
GameObject button9;
// button fronts
GameObject buttonfront1;
GameObject buttonfront2;
GameObject buttonfront3;
GameObject buttonfront4;
GameObject buttonfront5;
GameObject buttonfront6;
GameObject buttonfront7;
GameObject buttonfront8;
GameObject buttonfront9;
public Material greenLight;
public Material redLight;
public int maxButtons = 9;
public int minButtons = 1;
bool numGenerated;
bool isGenerated;
int[] buttons = new int[9] {1, 2, 3, 4, 5, 6, 7, 8, 9};
int random;
public void Start()
{
// defining buttons
button1 = GameObject.Find("PARENT_button1");
button2 = GameObject.Find("PARENT_button2");
button3 = GameObject.Find("PARENT_button3");
button4 = GameObject.Find("PARENT_button4");
button5 = GameObject.Find("PARENT_button5");
button6 = GameObject.Find("PARENT_button6");
button7 = GameObject.Find("PARENT_button7");
button8 = GameObject.Find("PARENT_button8");
button9 = GameObject.Find("PARENT_button9");
// defining front buttons
buttonfront1 = GameObject.Find("buttonfront_1");
buttonfront2 = GameObject.Find("buttonfront_2");
buttonfront3 = GameObject.Find("buttonfront_3");
buttonfront4 = GameObject.Find("buttonfront_4");
buttonfront5 = GameObject.Find("buttonfront_5");
buttonfront6 = GameObject.Find("buttonfront_6");
buttonfront7 = GameObject.Find("buttonfront_7");
buttonfront8 = GameObject.Find("buttonfront_8");
buttonfront9 = GameObject.Find("buttonfront_9");
isGenerated = false;
numGenerated = false;
generate();
StartCoroutine(waitbeforeStart());
}
IEnumerator waitbeforeStart()
{
yield return new WaitForSeconds(1);
StartCoroutine(ButtonAlgo());
}
void generate()
{
if (isGenerated == false)
{
random = Random.Range(0, 9);
Debug.Log(random);
isGenerated = true;
}
}
IEnumerator ButtonAlgo()
{
yield return new WaitForSeconds(1);
switch (random)
{
case 1:
button1.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront1.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 2:
button2.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront2.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 3:
button3.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront3.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 4:
button4.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront4.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 5:
button5.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront5.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 6:
button6.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront6.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 7:
button7.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront7.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 8:
button8.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront8.GetComponent<MeshRenderer>().material = greenLight;
yield break;
case 9:
button9.GetComponentInChildren<Light>().color = new Color32(23, 255, 0, 255);
buttonfront9.GetComponent<MeshRenderer>().material = greenLight;
yield break;
}
}
}
您錯過的是:要選擇一個隨機索引,您應該從0
開始。
所以將你的minButtonIndex
從1
更改為0
。
注意:使用 switch 而不是 if else 語句,如:
switch(finalNum)
{
case 1:
// run your code when final was equal to 1.
break;
// you can add as many case as you want to.
}
看:
您在使用它們之后設置minButtons
和maxButtons
,因此您應該在獲取隨機數之前設置它們。
看起來像這樣:
public int maxButtons = 9;
public int minButtons = 0;
int buttonValue = Random.Range(minButtons, maxButtons);
啊,我找到了,我希望
創建一個 Void 以生成一個值,但它僅在 bool 不為 true 時才生成一個 // isGenerated = false 默認情況下
void generate(){
if(isGenerated == true){
// generate one
isGenerated = true;
}
}
當您保護最終結果時,您可以將 isGenerated 設置為 false
這就是它最后的樣子
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ButtonSystem : MonoBehaviour
{
// buttons
GameObject button1;
GameObject button2;
GameObject button3;
GameObject button4;
GameObject button5;
GameObject button6;
GameObject button7;
GameObject button8;
GameObject button9;
// button fronts
GameObject buttonfront1;
GameObject buttonfront2;
GameObject buttonfront3;
GameObject buttonfront4;
GameObject buttonfront5;
GameObject buttonfront6;
GameObject buttonfront7;
GameObject buttonfront8;
GameObject buttonfront9;
public Material greenLight;
public Material redLight;
private color green = new Color32(23, 255, 0, 255);
public int maxButtons = 9;
public int minButtons = 1;
int buttonValue;
int finalValue;
bool waitready;
bool isGenerated; // <--here is a change
bool numLock;
bool numGenerated;
bool finalCalled;
int[] buttons = new int[9] {1, 2, 3, 4, 5, 6, 7, 8, 9};
public void Start()
{
// defining buttons
button1 = GameObject.Find("PARENT_button1");
button2 = GameObject.Find("PARENT_button2");
button3 = GameObject.Find("PARENT_button3");
button4 = GameObject.Find("PARENT_button4");
button5 = GameObject.Find("PARENT_button5");
button6 = GameObject.Find("PARENT_button6");
button7 = GameObject.Find("PARENT_button7");
button8 = GameObject.Find("PARENT_button8");
button9 = GameObject.Find("PARENT_button9");
// defining front buttons
buttonfront1 = GameObject.Find("buttonfront_1");
buttonfront2 = GameObject.Find("buttonfront_2");
buttonfront3 = GameObject.Find("buttonfront_3");
buttonfront4 = GameObject.Find("buttonfront_4");
buttonfront5 = GameObject.Find("buttonfront_5");
buttonfront6 = GameObject.Find("buttonfront_6");
buttonfront7 = GameObject.Find("buttonfront_7");
buttonfront8 = GameObject.Find("buttonfront_8");
buttonfront9 = GameObject.Find("buttonfront_9");
isGenerated = false; // <-- here is a change
waitready = false;
numLock = false;
numGenerated = false;
finalCalled = false;
StartCoroutine(waitbeforeStart());
}
void generate(){
if(isGenerated == true){
int finalValue = buttons[Random.Range(0, buttons.Length)];
finalCalled = true;
numLock = true;
isGenerated = true;
}
}
IEnumerator waitbeforeStart()
{
yield return new WaitForSeconds(1);
waitready = true;
StartCoroutine(ButtonAlgo(waitready, numGenerated, numLock, buttons, finalCalled));
}
IEnumerator ButtonAlgo(bool waitready, bool numGenerated, bool numLock, int[] buttons, bool finalCalled)
{
// buttonAlgostart
if (waitready && !numGenerated && !numLock)
{
numGenerated = true;
numLock = false;
finalCalled = false;
int fV;
//int buttonValue = Random.Range(minButtons, maxButtons);
if (!finalCalled && !numLock)
{
generate();
yield return new WaitForSeconds(1);
fV = finalValue;
yield return new WaitForSeconds(3);
Debug.Log(fV); // ? why ?
switch (finalValue){
case 1:
waitready = false;
button2.GetComponentInChildren<Light>().color = green;
buttonfront2.GetComponent<MeshRenderer>().material = greenLight;
break;
case 2:
waitready = false;
button2.GetComponentInChildren<Light>().color = green;
buttonfront2.GetComponent<MeshRenderer>().material = greenLight;
break;
case 3:
waitready = false;
button3.GetComponentInChildren<Light>().color = green;
buttonfront3.GetComponent<MeshRenderer>().material = greenLight;
break;
case 4:
waitready = false;
button4.GetComponentInChildren<Light>().color = green;
buttonfront4.GetComponent<MeshRenderer>().material = greenLight;
break;
case 5:
waitready = false;
button5.GetComponentInChildren<Light>().color = green;
buttonfront5.GetComponent<MeshRenderer>().material = greenLight;
break;
case 6:
waitready = false;
button6.GetComponentInChildren<Light>().color = green;
buttonfront6.GetComponent<MeshRenderer>().material = greenLight;
break;
case 7:
waitready = false;
button7.GetComponentInChildren<Light>().color = green;
buttonfront7.GetComponent<MeshRenderer>().material = greenLight;
break;
case 8:
waitready = false;
button8.GetComponentInChildren<Light>().color = green;
buttonfront8.GetComponent<MeshRenderer>().material = greenLight;
break;
case 9:
waitready = false;
button9.GetComponentInChildren<Light>().color = green;
buttonfront9.GetComponent<MeshRenderer>().material = greenLight;
break;
}
isGenerated = false;
}
}
}
}
我認為增加yield break;
在每個語句中都可以解決您的問題。
我不明白你的邏輯,你更新方法內部的臨時變量而不是更新你在 class 中定義的變量?
public class ButtonSystem : MonoBehaviour
{
:
:
public int maxButtons = 9;
public int minButtons = 1;
int buttonValue;
int finalValue;
bool waitready;
bool numLock;
bool numGenerated;
bool finalCalled;
int[] buttons = new int[9] {1, 2, 3, 4, 5, 6, 7, 8, 9};
IEnumerator waitbeforeStart()
{
yield return new WaitForSeconds(1);
waitready = true;
StartCoroutine(ButtonAlgo());
}
IEnumerator ButtonAlgo()
找到答案了,所以我找到了答案,而且我最初擁有的腳本是正確的。 在我的項目中,我有 9 個按鈕,腳本應用於每個按鈕,這意味着它生成了 9 個不同的數字,這讓我很困惑,我剛剛發現了這一點,感謝大家的幫助:)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.