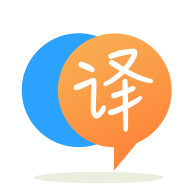
[英]Why on some targets the player is not looking at the target when using Physics.Raycast and ik?
[英]When using ik to look at target if the target is rotating around the player when the target is coming from behind the player is not looking at it why?
using UnityEngine;
using System;
using System.Collections;
using System.Collections.Generic;
[RequireComponent(typeof(Animator))]
public class TestingIK : MonoBehaviour
{
public List<Transform> lookObjects = new List<Transform>();
public float weightDamping = 1.5f;
private Animator animator;
private Transform lastPrimaryTarget;
private float lerpEndDistance = 0.1f;
private float finalLookWeight = 0;
private bool transitionToNextTarget = false;
void Start()
{
animator = GetComponent<Animator>();
}
// Callback for calculating IK
void OnAnimatorIK()
{
if (lookObjects != null)
{
Transform primaryTarget = null;
float closestLookWeight = 0;
// Here we find the target which is closest (by angle) to the players view line
foreach (Transform target in lookObjects)
{
Vector3 lookAt = target.position - transform.position;
lookAt.y = 0f;
float dotProduct = Vector3.Dot(new Vector3(transform.forward.x, 0f, transform.forward.z).normalized, lookAt.normalized);
float lookWeight = Mathf.Clamp(dotProduct, 0f, 1f);
if (lookWeight > closestLookWeight)
{
closestLookWeight = lookWeight;
primaryTarget = target;
}
}
if (primaryTarget != null)
{
if ((lastPrimaryTarget != null) && (lastPrimaryTarget != primaryTarget) && (finalLookWeight > 0f))
{
// Here we start a new transition because the player looks already to a target but
// we have found another target the player should look at
transitionToNextTarget = true;
}
}
// The player is in a neutral look position but has found a new target
if ((primaryTarget != null) && !transitionToNextTarget)
{
lastPrimaryTarget = primaryTarget;
finalLookWeight = Mathf.Lerp(finalLookWeight, closestLookWeight, Time.deltaTime * weightDamping);
float bodyWeight = finalLookWeight * .75f;
animator.SetLookAtWeight(finalLookWeight, bodyWeight, 1f);
animator.SetLookAtPosition(primaryTarget.position);
}
// Let the player smoothly look away from the last target to the neutral look position
if ((primaryTarget == null && lastPrimaryTarget != null) || transitionToNextTarget)
{
finalLookWeight = Mathf.Lerp(finalLookWeight, 0f, Time.deltaTime * weightDamping);
float bodyWeight = finalLookWeight * .75f;
animator.SetLookAtWeight(finalLookWeight, bodyWeight, 1f);
animator.SetLookAtPosition(lastPrimaryTarget.position);
if (finalLookWeight < lerpEndDistance)
{
transitionToNextTarget = false;
finalLookWeight = 0f;
lastPrimaryTarget = null;
}
}
}
}
}
我有一個圍繞玩家轉圈的立方體。
當立方體從左側移到玩家身后時,玩家會稍微向后看,然后將頭返回,但是當立方體從右側返回時,玩家根本沒有看它,他開始只有當立方體幾乎就在他面前時才看立方體。
當立方體從右側從后面回到前面時,右側不活動。
更新 :
我發現如果我改變這條線
float dotProduct = Vector3.Dot(new Vector3(transform.forward.x, 0f, transform.forward.z).normalized, lookAt.normalized);
在 x 和 z 的前向前加上減號:
float dotProduct = Vector3.Dot(new Vector3(-transform.forward.x, 0f, -transform.forward.z).normalized, lookAt.normalized);
現在,當對象從右側從后面進來但現在左側不工作時,它會正常工作。
所以它要么是從前到后的左側,沒有減號,要么是從后到前的右側,有減號。
我該如何解決它,以便它可以在前面/后面平等地工作?
Vector3.Dot
當目標在玩家身后時會返回負數,不幸的是你將結果鉗制為 [0, 1] 並且最小權重為 0,這就是后面目標被忽略的原因。
要修復它,請直接使用點積值並使用足夠小的初始權重。
float closestLookWeight = float.MinValue;
float lookWeight = dotProduct;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.