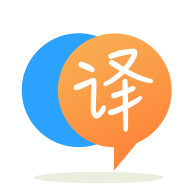
[英]Can I retrieve the confidence of the transcription from Azure Cognitive Services?
[英]Can I retrieve the confidence of the recognized from Azure Cognitive Services?
我正在以 C 尖銳的 window 形式對文本應用程序進行語音。 它工作正常並在視覺工作室中運行,但是
我正在使用此代碼來識別使用 Microsoft Azure 認知服務。 一旦整個事情都被識別出來,我可以在 c 尖銳 window 表格中獲得信心分數嗎?
我該如何解決這個問題?
我的代碼:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Microsoft.CognitiveServices.Speech;
using Microsoft.CognitiveServices.Speech.Audio;
using System.IO;
using System.Threading;
namespace WindowsFormsApp2
{
public partial class Form1 : Form
{
private bool isRecognizing = false;
private SpeechRecognizer recognizer;
public Form1()
{
InitializeComponent();
}
private void initRecognizer()
{
SpeechConfig config = SpeechConfig.FromSubscription("key", "region");
if (Properties.Settings.Default.Punctuation)
{
config.SetServiceProperty("punctuation", "explicit", ServicePropertyChannel.UriQueryParameter);
}
//AudioConfig audioConfig = AudioConfig.FromMicrophoneInput();
recognizer = new SpeechRecognizer(config/*, audioConfig*/);
recognizer.Recognized += SpeechRecognizer_Recognized;
}
private void Form1_Load(object sender, EventArgs e)
{
initRecognizer();
}
private void SpeechRecognizer_Recognized(object sender, SpeechRecognitionEventArgs e)
{
if (e.Result.Reason == ResultReason.RecognizedSpeech)
{
if (e.Result.Text.ToLower().Equals("new line") || e.Result.Text.ToLower().Equals("newline"))
{
SendKeys.SendWait(Environment.NewLine);
}
else
{
SendKeys.SendWait(e.Result.Text);
}
}
}
private void Startstop()
{
if (isRecognizing)
{
recognizer.StopContinuousRecognitionAsync();
picture_btn.Image = Properties.Resources.green;
startToolStripMenuItem.Text = "Start";
pictureBox1.Enabled = true;
isRecognizing = false;
timer1.Stop();
timer1.Enabled = false;
}
else
{
picture_btn.Image = Properties.Resources.red;
startToolStripMenuItem.Text = "Stop";
pictureBox1.Enabled = false;
recognizer.StartContinuousRecognitionAsync();
isRecognizing = true;
timer1.Interval = 600;
timer1.Start();
timer1.Enabled = true;
}
}
private void pictureBox1_Click(object sender, EventArgs e)
{
Startstop();
}
private void Form1_Move(object sender, EventArgs e)
{
if (this.WindowState == FormWindowState.Normal)
{
ShowInTaskbar = true;
notifyIcon1.Visible = true;
this.Hide();
notifyIcon1.ShowBalloonTip(1000);
}
}
private void Form1_MouseDoubleClick(object sender, MouseEventArgs e)
{
ShowInTaskbar = true;
notifyIcon1.Visible = false;
WindowState = FormWindowState.Normal;
this.WindowState = FormWindowState.Normal;
notifyIcon1.Visible = false;
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
Application.Exit();
}
void SettingFormClosed(object sender, FormClosedEventArgs e)
{
initRecognizer();
}
private void startToolStripMenuItem_Click(object sender, EventArgs e)
{
Startstop();
}
private void timer1_Tick(object sender, EventArgs e)
{
if (picture_btn.Tag.Equals("red"))
{
picture_btn.Image = Properties.Resources.grey;
picture_btn.Tag = "grey";
}
else
{
picture_btn.Image = Properties.Resources.red;
picture_btn.Tag = "red";
}
}
private void pictureBox1_Click_1(object sender, EventArgs e)
{
var myForm = new Form2();
myForm.FormClosed += SettingFormClosed;
myForm.Show();
}
private void notifyIcon1_MouseDoubleClick(object sender, MouseEventArgs e)
{
this.Show();
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (e.CloseReason == CloseReason.UserClosing)
{
notifyIcon1.Visible = true;
this.Hide();
e.Cancel = true;
}
}
}
}
在深入研究服務之前,您應該始終通讀服務文檔。 該文檔將涵蓋重要的配置方面,並應詳細說明限制。
Azure - 認知服務 - 語音服務 - 語音轉文本文檔
這是語音到文本服務的所有標准資源的登錄頁面,通讀所有這些以了解該服務的工作原理並獲得常見場景的代碼示例。
- 獲取語音識別結果
這是特定於SpeechRecognizer
事件和Results
的指南- 確認的偏移量和持續時間
本節說明如何獲得詳細結果。
您需要配置SpeechRecognizer
實例以返回詳細的 output:
SpeechConfig config = SpeechConfig.FromSubscription("key", "region");
// Detailed output will include confidence factor
config.OutputFormat = OutputFormat.Detailed;
if (Properties.Settings.Default.Punctuation)
{
config.SetServiceProperty("punctuation", "explicit", ServicePropertyChannel.UriQueryParameter);
}
Best()
擴展方法訪問詳細信息這在本節中記錄: 識別偏移量和持續時間但是一般的想法是我們調用e.Result.Best()
擴展方法來檢索有關結果的詳細信息。
確保您具有以下 using 語句以使
Best()
方法可用:using Microsoft.CognitiveServices.Speech;
private void SpeechRecognizer_Recognized(object sender, SpeechRecognitionEventArgs e)
{
if (e.Result.Reason == ResultReason.RecognizedSpeech)
{
if (e.Result.Text.ToLower().Equals("new line") || e.Result.Text.ToLower().Equals("newline"))
SendKeys.SendWait(Environment.NewLine);
else
SendKeys.SendWait(e.Result.Text);
// Get the detailed results
var detailedResults = e.Result.Best();
if (detailedResults != null && detailedResults.Any())
{
// The first item in detailedResults corresponds to the recognized text.
// This is not necessarily the item with the highest confidence number.
var bestResults = detailedResults?.ToList()[0];
Console.WriteLine(String.Format("\tConfidence: {0}\n\tText: {1}\n\tLexicalForm: {2}\n\tNormalizedForm: {3}\n\tMaskedNormalizedForm: {4}",
bestResults.Confidence,
bestResults.Text,
bestResults.LexicalForm,
bestResults.NormalizedForm,
bestResults.MaskedNormalizedForm));
}
}
例如,您可以從服務中獲取此級別的信息,我還啟用了speechConfig.RequestWordLevelTimestamps()
以獲取單詞級別的時間戳:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.