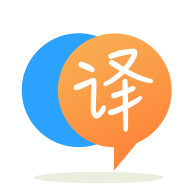
[英]Print Value of pi. How many terms of this series do you have to use before you first get 3.14? 3.141? 3.1415? 3.14159?
[英]In C language how to calculate the term number of infinite series where we get pi=3.14, pi=3.141,pi=3.1415 and finally pi=3.14159?
問題是:從無限級數中計算出 π 的值。 打印一張表格,顯示用該級數的一項、兩項、三項等近似的 π 值。 在您第一次獲得 3.14 之前,您必須使用這個系列的多少項? 3.141? 3.1415? 3.14159?
int n = 2;
double sum,
pi = 4,
den;
printf("Calculating the value of pi.\n");
while (pi != 3.140000) {
if (n % 2 == 0) {
den = (2 * n) - 1;
sum = (4.0 / den);
pi = pi - sum;
}
else {
den = (2 * n) - 1;
sum = (4.0 / den);
pi = pi + sum;
}
pi = (round(pi * 100)) / 100;
printf("pi=%lf\n", pi);
if (pi == 3.140000) {
break;
}
n = n + 1;
}
printf("The number of terms to get pi=3.14 is %d.\n", n - 2);
此代碼有效,並表明我們將在第 30 學期獲得 3.14,但是當我重復此代碼以獲取 pi=3.141、pi=3.1415 和 pi=3.14159 的學期編號時,代碼不起作用並且在執行時僅顯示黑色屏幕上有語句,計算 pi 的值。 這不是我想要的確切 output,但有點像。 實際上我希望表格打印到我得到 3.14,然后我應該得到顯示術語編號的語句,我得到 3.14,表格從我離開的地方開始,一旦我得到 3.141,語句顯示術語編號在那里我得到 3.141 版畫等等。 請在這方面幫助我。(使用 C 語言)(我必須在不使用 prec、trunc 或其他一些功能的情況下執行此操作,因為我不允許這樣做)
編輯:我這樣做了:
int
main()
{
int n = 2,
x = 2,
y = 2,
z = 2;
double sum,
pi = 4;
printf("Calculating the value of pi.\n");
while (pi != 3.140000) {
if (n % 2 == 0) {
sum = (4.0 / ((2 * n) - 1));
pi = pi - sum;
}
else {
sum = (4.0 / ((2 * n) - 1));
pi = pi + sum;
}
pi = (round(pi * 100)) / 100;
if (pi == 3.140000) {
break;
}
n = n + 1;
}
while (pi != 3.141000) {
if (n % 2 == 0) {
sum = (4.0 / ((2 * n) - 1));
pi = pi - sum;
}
else {
sum = (4.0 / ((2 * n) - 1));
pi = pi + sum;
}
pi = (round(pi * 100)) / 100;
if (pi == 3.141000) {
break;
}
n = n + 1;
}
while (pi != 3.141500) {
if (n % 2 == 0) {
sum = (4.0 / ((2 * n) - 1));
pi = pi - sum;
}
else {
sum = (4.0 / ((2 * n) - 1));
pi = pi + sum;
}
pi = (round(pi * 100)) / 100;
if (pi == 3.141500) {
break;
}
n = n + 1;
}
while (pi != 3.141590) {
if (n % 2 == 0) {
sum = (4.0 / ((2 * n) - 1));
pi = pi - sum;
}
else {
sum = (4.0 / ((2 * n) - 1));
pi = pi + sum;
}
pi = (round(pi * 100)) / 100;
if (pi == 3.141590) {
break;
}
n = n + 1;
}
printf("The number of terms to get pi=3.14 is %d.\n", n - 2);
printf("The number of terms to get pi=3.141 is %d.\n", n - 2);
printf("The number of terms to get pi=3.1415 is %d.\n", n - 2);
printf("The number of terms to get pi=3.14159 is %d.\n", n - 2);
}
但屏幕顯示沒有 output。
while ( pi.= 3.140000 )
要求您已經知道答案。 如果你這樣做了,你為什么要計算pi
呢?
你應該使用的是像
double precision = 0.01;
double pi = 4;
while ( 1 ) {
double previous_pi = 4;
# Refine the value of `pi` here...
if ( fabs( previous_pi - pi ) < precision / 2 )
break;
}
這給了我們你問題的答案。 只需改變0.01
即可獲得不同的精度。
當您正在尋找諸如“3.14100”之類的值時,明顯永無止境的過程的原因是您需要為更多的小數位提高舍入精度。 在您的代碼中,此語句使您感到困惑。
pi=(round(pi*100))/100;
此語句決不允許代碼以大於兩位小數的精度查看值。
為了使您的代碼更加健壯,以下是您的代碼的一個調整版本,其中包含一些 #define 語句來標識要查詢的值以及所需小數點右側的精度。
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define check 3.14100
#define precision 1000
int main()
{
int n=2;
double sum,pi=4.000000,den;
printf("Calculating the value of pi.\n");
while(pi != check)
{
if(n%2==0)
{
den=(2*n)-1;
sum=(4.0/den);
pi=pi-sum;
}
else
{
den=(2*n)-1;
sum=(4.0/den);
pi=pi+sum;
}
pi=(round(pi*precision))/precision; /* Provide enough precision */
printf("pi=%lf\n",pi);
if(pi == check)
{
break;
}
n=n+1;
}
printf("The number of terms to get pi= %f is %d.\n", check, n-2);
return 0;
}
主要內容是要使用的精度乘數/除數。
當使用值“3.14100”進行測試時,以下是終端上的最后幾行 output。
pi=3.142000
pi=3.137000
pi=3.141000
The number of terms to get pi= 3.141000 is 443.
我使用固定的 #define 語句,但您可以對測試值執行一些位置檢查,以提出一種更靈活的方法來確定所需的精度值。
試試看它是否符合您項目的精神。
與其限制為pi.= 3.14xxx
,不如對值使用公差。
fabs(pi - pi_Appoximate) >= 0.5 / limit
只需對 OP 的代碼進行一些更改,就可以迭代而不是復制代碼,增加 10 的冪。
int main(void) {
printf("Calculating the value of pi.\n");
char *pi_string = "3.1415926535897932384626433832795";
for (unsigned i = 4; i<17; i++) {
char buf[i + 1];
buf[0] = '\0';
double pi_Appoximate = atof(strncat(buf, pi_string, i));
double limit = pow(10, i - 1);
unsigned n = 2;
double sum;
double pi = 4;
printf("i:%2u limit:%e ~pi:%.*g", i, limit, i, pi_Appoximate);
fflush(stdout);
while (fabs(pi - pi_Appoximate) >= 0.5 / limit) {
if (n % 2 == 0) {
sum = (4.0 / ((2 * n) - 1));
pi = pi - sum;
} else {
sum = (4.0 / ((2 * n) - 1));
pi = pi + sum;
}
pi = (round(pi * limit)) / limit;
n = n + 1;
}
printf(" pi:%.*g n:%u\n", i, pi, n);
}
puts(pi_string);
}
Output
Calculating the value of pi.
i: 4 limit:1.000000e+03 ~pi:3.14 pi:3.14 n:802
i: 5 limit:1.000000e+04 ~pi:3.141 pi:3.141 n:1145
i: 6 limit:1.000000e+05 ~pi:3.1415 pi:3.1415 n:36366
i: 7 limit:1.000000e+06 ~pi:3.14159 pi:3.14159 n:72729
i: 8 limit:1.000000e+07 ~pi:3.141592 pi:3.141592 n:1379312
i: 9 limit:1.000000e+08 ~pi:3.1415926 pi:3.1415926 n:2649009
i:10 limit:1.000000e+09 ~pi:3.14159265 pi:3.14159265 n:7476638
i:11 limit:1.000000e+10 ~pi:3.141592653 pi:3.141592653 n:40363271
i:12 limit:1.000000e+11 ~pi:3.1415926535 pi:3.1415926535 n:58402689
i:13 limit:1.000000e+12 ~pi:3.14159265358 pi:3.14159265358 n:62874301
i:14 limit:1.000000e+13 ~pi:3.141592653589 pi:3.141592653589 n:68508285
i:15 limit:1.000000e+14 ~pi:3.1415926535897 pi:3.1415926535897 n:53634827
i:16 limit:1.000000e+15 ~pi:3.14159265358979 pi:3.14159265358979 n:59359529
3.1415926535897932384626433832795
由於double
數學精度約為 15-17 位十進制數字,因此幾乎不需要 go。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.