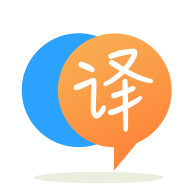
[英]How do I add a JsonIgnore attribute to all properties with a specific return type in a class using Roslyn?
[英]How do I find all properties of type DateTime in an class?
我需要調整一堆對象的日期時間。
我想循環遍歷類的屬性,如果類型是dateTime相應調整。
是否有任何形式的“描述類型”我可以使用?
你可以使用反射 。
您的方案可能看起來像這樣:
static void Main(string[] args)
{
var list = new List<Mammal>();
list.Add(new Person { Name = "Filip", DOB = DateTime.Now });
list.Add(new Person { Name = "Peter", DOB = DateTime.Now });
list.Add(new Person { Name = "Goran", DOB = DateTime.Now });
list.Add(new Person { Name = "Markus", DOB = DateTime.Now });
list.Add(new Dog { Name = "Sparky", Breed = "Unknown" });
list.Add(new Dog { Name = "Little Kid", Breed = "Unknown" });
list.Add(new Dog { Name = "Zorro", Breed = "Unknown" });
foreach (var item in list)
Console.WriteLine(item.Speek());
list = ReCalculateDOB(list);
foreach (var item in list)
Console.WriteLine(item.Speek());
}
你想在哪里重新計算所有哺乳動物的生日。 以上的實現看起來像這樣:
internal interface Mammal
{
string Speek();
}
internal class Person : Mammal
{
public string Name { get; set; }
public DateTime DOB { get; set; }
public string Speek()
{
return "My DOB is: " + DOB.ToString() ;
}
}
internal class Dog : Mammal
{
public string Name { get; set; }
public string Breed { get; set; }
public string Speek()
{
return "Woff!";
}
}
所以基本上你需要做的是使用Relfection,它是一種機制,用於檢查類型並獲取類型屬性以及運行時類似的其他內容。 下面是一個關於如何為每個獲得DOB的哺乳動物的上述DOB添加10天的示例。
static List<Mammal> ReCalculateDOB(List<Mammal> list)
{
foreach (var item in list)
{
var properties = item.GetType().GetProperties();
foreach (var property in properties)
{
if (property.PropertyType == typeof(DateTime))
property.SetValue(item, ((DateTime)property.GetValue(item, null)).AddDays(10), null);
}
}
return list;
}
請記住,使用反射可能很慢,而且通常很慢。
但是,上面會打印出這個:
My DOB is: 2010-03-22 09:18:12
My DOB is: 2010-03-22 09:18:12
My DOB is: 2010-03-22 09:18:12
My DOB is: 2010-03-22 09:18:12
Woff!
Woff!
Woff!
My DOB is: 2010-04-01 09:18:12
My DOB is: 2010-04-01 09:18:12
My DOB is: 2010-04-01 09:18:12
My DOB is: 2010-04-01 09:18:12
Woff!
Woff!
Woff!
它被稱為反射。
var t = this;
var props = t.GetType().GetProperties();
foreach (var prop in props)
{
if (prop.PropertyType == typeof(DateTime))
{
//do stuff like prop.SetValue(t, DateTime.Now, null);
}
}
class HasDateTimes
{
public DateTime Foo { get; set; }
public string NotWanted { get; set; }
public DateTime Bar { get { return DateTime.MinValue; } }
}
static void Main(string[] args)
{
foreach (var propertyInfo in
from p in typeof(HasDateTimes).GetProperties()
where Equals(p.PropertyType, typeof(DateTime)) select p)
{
Console.WriteLine(propertyInfo.Name);
}
}
查找反思,但基本上你這樣做
obj.GetType()。GetProperties(.. Instance | ..Public),你得到了一個定義屬性的列表..檢查屬性的值類型,並將其與typeof(DateTime)進行比較。
對於非常快速的答案和指向該問題的指示,如果您有PowerShell可用(Vista / Windows 7,Windows 2008已安裝它),您可以啟動控制台和DateTime例如
Get-Date | Get-Member
這將列出DateTime實例的成員。 您還可以查看靜態成員:
Get-Date | Get-Member -Static
如果您擔心反射對性能的影響,您可能會對Fasterflect感興趣, 這是一個庫,可以更輕松,更快速地查詢和訪問成員。
對於instace,可以使用Fasterflect重寫MaxGuernseyIII的代碼,如下所示:
var query = from property in typeof(HasDateTimes).Properties()
where property.Type() == typeof(DateTime)
select p;
Array.ForEach( query.ToArray(), p => Console.WriteLine( p.Name ) );
Fasterflect使用輕量級代碼生成來更快地訪問(如果您直接緩存和調用生成的委托,則需要2-5倍,或者接近原生速度)。 查詢成員通常更容易,更方便,但不是更快。 請注意,這些數字不包括JIT編譯生成的代碼的重要初始開銷,因此性能增益僅在重復訪問時才可見。
免責聲明:我是該項目的貢獻者。
嘿這個問題有點老了但是你可以用這個:
在類中(選擇所有值) - 設置值將在沒有強制轉換的情況下運行,僅用於選擇:
(from property in this.GetType().GetProperties()
where property.PropertyType == typeof(DateTime)
select property.GetValue(this)).Cast<DateTime>()
課外將是:
var instance = new MyClass();
var times = (from property in instance.GetType().GetProperties()
where property.PropertyType == typeof(DateTime)
select property.GetValue(instance)).Cast<DateTime>()
為了獲得最大日期時間值,我運行它
var lastChange = (from property in this.GetType().GetProperties()
where property.PropertyType == typeof(DateTime)
select property.GetValue(this)).Cast<DateTime>().Max();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.