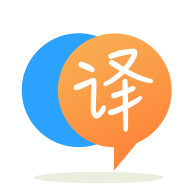
[英]Implementing ordering for List of objects in C# and methods for changing the index of the object
[英]C# Changing Objects within a List
我在使用找到的索引更改列表中對象的成員時遇到了一些問題。
所以這是我目前正在使用的方法:
static void addToInventory(ref List<baseItem> myArray, baseItem item, float maxAmount, ref float currentAmount)
{
if (currentAmount + item.getWeight() <= maxAmount)
{
Console.WriteLine("item.Quantity = {0}", item.Quantity);
if (myArray.Contains(item))
{
Console.WriteLine("Item ({0}) already exists", item.Name);
int id = myArray.IndexOf(item);
myArray[id].Quantity += item.Quantity;//Change occurs in this line, item.Quantity becomes the same as myArray[id].Quantity
}
else
{
Console.WriteLine("Adding new item ({0})", item.Name);
myArray.Add(item);
}
currentAmount += item.getWeight();
}
else
{
Console.WriteLine("Inventory full");
}
myArray.Sort();
}
此方法采用幾個參數,包括清單/清單。 我檢查該項目是否適合,如果適合,我查看列表中是否還有另一個同名項目,找到索引,並添加更多項目。 但是,突然增加的項目數量變得與列表中的項目數量相同。 由於某些原因,這也會更改列表外的商品數量。 因此,與其像這樣累加數量:1、2、3、4,他們不這樣累加累加:1、2、4、8。我如何做到這一點,以使增加的項目數量不變?
我剛剛開始學習如何使用列表,因此,如果我缺少任何內容,請隨時批評。 提前致謝。
標記:感謝您的快速回復! 不好意思的命名(myArray); 它曾經是一個ArrayList。 currentAmount和maxAmount分別指庫存中的當前重量和庫存可以容納的最大重量。 另外,我不想在數量上加1。 我希望它添加所傳遞物品的數量。感謝您的提示。 我可能會考慮使用Dictionary來代替。
這里發生的是myArray[id]
和item
引用相同的對象 。 List.Contains
按引用而不是按值進行比較。
所以看起來您只想做
if (myArray.Contains(item))
{
item.Quantity++;
}
表示列表中還有該項目。
但是,以這種方式使用列表從根本上來說是不正確的方法。 您應該使用Dictionary或Set來實現O(1)查找。
如果您選擇字典路線,則會遇到以下情況:
// a Set might be better -- I don't know what you're using this for
var myDict = new Dictionary<string, BaseItem>();
// ...
if (currentAmount + item.Weight <= maxAmount)
{
if (myDict.ContainsKey(item.Name))
{
Console.WriteLine("Item already exists");
item.Quantity++;
}
else
{
Console.WriteLine("Adding new item");
myDict[item.Name] = item;
}
currentAmount += item.Weight;
}
else
{
Console.WriteLine("Full");
}
其他一些注意事項:
您最好使用字典,在字典中使用項目的名稱來索引單個項目。
從這種意義上講,您不必去搜索Item(並保持與示例相似),而只需
/* Remove ref on dictionary, you're not setting myArray to something else */
static void addToInventory(Dictionary<string, baseItem> myArray, baseItem item, float maxAmount, ref float currentAmount)
{
if (currentAmount + item.getWeight() <= maxAmount)
{
Console.WriteLine("item.Quantity = {0}", item.Quantity);
if (myArray[item.Name] == null)
Console.WriteLine("Adding new item ({0})", item.Name);
else
Console.WriteLine("Item ({0}) already exists", item.Name);
myArray[item.Name] = myArray[item.Name] ?? item;
myArray[item.Name].Quantity += item.Quantity;
currentAmount += item.getWeight();
}
else
{
Console.WriteLine("Inventory full");
}
}
我也很想創建自己的Inventory類(擁有自己的Dictionary字段),以便您可以進行方法鏈接,即:
Inventory i = new Inventory(maxAmount);
float currentItemCount = i.add(item).add(item).add(item).getQuantity(item);
您可以通過使add方法返回“ this”(即,輸入Inventory而不是void)來實現此目的。
getQuantity只是“返回myArray [item.name] .Quantity”,當然,您不應在庫存類“ myArray”中調用Dictionary變量。
這些行引用列表中的同一項目
int id = myArray.IndexOf(item);
myArray[id].Quantity += item.Quantity;//Change occurs in this line, item.Quantity becomes the same as myArray[id].Quantity
因為item
已經在列表中,所以當您說IndexOf
時,您將獲得另一個變量,該變量引用同一對象。 似乎您可能在程序的早期邏輯中存在錯誤,因為item
始終具有相同數量的myArray[id].Quantity
。
從您的代碼片段中,您似乎想創建一個新項目並確定該項目類型是否已在列表中。 如果是,則將其數量添加到該類型的現有項目中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.