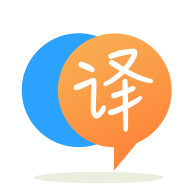
[英]Is there any shorter way of getting an intersection and union of two strings?
[英]Intersection and union of two strings
我必須消除字符串1中出現的字符串2,並找到兩個字符串的交集。
這是我嘗試過的:
#include "stdafx.h"
#include "stdio.h"
#include "conio.h"
#include "string.h"
class operation
{
public:
char string1[100];
char string2[50];
operation(){};
operation(char a[100], char b[50]);
operation operator+(operation);
operation operator-(operation);
operation operator*(operation);
};
operation::operation(char a[100], char b[50])
{
strcpy(string1, a);
strcpy(string2, b);
}
operation operation::operator +(operation param)
{
operation temp;
strcpy(param.string1, temp.string1);
strcpy(param.string2, temp.string2);
strcat(temp.string1, temp.string2);
return (temp);
}
operation operation::operator -(operation param)
{
operation temp;
strcpy(param.string1, temp.string1);
strcpy(param.string2, temp.string2) ;
for (int i = 0; i<strlen(temp.string2); i++)
{
temp.string1.erase(i, 1);
}
return (temp);
}
operation operation::operator *(operation param)
{
operation temp;
strcpy(param.string1, temp.string1);
strcpy(param.string2, temp.string2);
char result[50];
for(int i = 0; i<strlen(temp.string2); i++)
{
if( temp.string1.find( temp.string2[i] ) != string::npos )
result = result + temp.string2[i];
}
return (temp);
}
我收到了編譯器錯誤,我也不確定我在嘗試是否正確。
錯誤如下:
C2228: left of .erase must have class/struct/union
C2228: left of .find must have class/struct/union
令人高興的是,在C ++中,設置差異 , 交集和聯合算法已經在標准庫中實現。 這些可以應用於任何容器類的字符串。
這是一個演示(您可以使用簡單的char
數組執行此操作,但為了清晰起見,我使用std::string
):
#include <string>
#include <algorithm>
#include <iostream>
int main()
{
std::string string1 = "kanu";
std::string string2 = "charu";
std::string string_difference, string_intersection, string_union;
std::sort(string1.begin(), string1.end());
std::sort(string2.begin(), string2.end());
std::set_difference(string1.begin(), string1.end(), string2.begin(), string2.end(), std::back_inserter(string_difference));
std::cout << "In string1 but not string2: " << string_difference << std::endl;
std::set_intersection(string1.begin(), string1.end(), string2.begin(), string2.end(), std::back_inserter(string_intersection));
std::cout << "string1 intersect string2: " << string_intersection << std::endl;
std::set_union(string1.begin(), string1.end(), string2.begin(), string2.end(), std::back_inserter(string_union));
std::cout << "string1 union string2: " << string_union << std::endl;
}
如何在operation
類中實現它作為練習。
如果是strcpy( string1...
編譯那么string1
是一個char*
而不是一個std::string
。你似乎在混合std::string
C和C ++功能。選擇一個並堅持下去(我說std::string
自你在做C ++)
這是C ++(不是C:C沒有運算符重載)
在我們幫助識別編譯錯誤之前,您需要向我們展示您的類定義。
如果你沒有“operator”的類定義,那就單獨解釋了錯誤:)
如果您使用的是C ++,則應該使用標准C ++“string”(而不是C“char []”數組)。 使用“string”也會影響您的實現代碼。
問:這不是家庭作業,不是嗎? 如果是這樣,請在標簽中添加“作業”。
提前完成.. PSM
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.