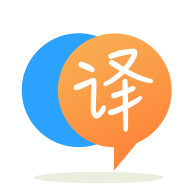
[英]How to display decimal value to 2 decimal places in datatable to display in datagrid
[英]How do I display a decimal value to 2 decimal places?
當前使用.ToString()
顯示小數的值時,精確到小數點后 15 位,並且由於我用它來表示美元和美分,所以我只希望 output 為小數點后 2 位。
我是否為此使用.ToString()
的變體?
decimalVar.ToString("#.##"); // returns ".5" when decimalVar == 0.5m
或者
decimalVar.ToString("0.##"); // returns "0.5" when decimalVar == 0.5m
或者
decimalVar.ToString("0.00"); // returns "0.50" when decimalVar == 0.5m
我知道這是一個老問題,但我很驚訝地發現似乎沒有人發布答案;
這就是我會使用的:
decimal.Round(yourValue, 2, MidpointRounding.AwayFromZero);
decimalVar.ToString("F");
這會:
23.456
→ 23.46
23
→ 23.00
; 12.5
→ 12.50
顯示貨幣的理想選擇。
查看關於ToString("F")的文檔(感謝 Jon Schneider)。
如果你只需要這個來顯示使用 string.Format
String.Format("{0:0.00}", 123.4567m); // "123.46"
http://www.csharp-examples.net/string-format-double/
“m”是十進制后綴。 關於十進制后綴:
給定十進制 d=12.345; 表達式d.ToString("C")或String.Format("{0:C}", d)產生$12.35 - 請注意,使用了當前文化的貨幣設置,包括符號。
請注意, “C”使用當前文化中的位數。 您始終可以覆蓋默認值以使用C{Precision specifier}
強制必要的精度,例如String.Format("{0:C2}", 5.123d)
。
如果您希望它使用逗號和小數點(但沒有貨幣符號)格式化,例如 3,456,789.12 ...
decimalVar.ToString("n2");
Decimal
有一個非常重要的特征,但並不明顯:
Decimal
根據它的來源“知道”它有多少個小數位
以下情況可能出乎意料:
Decimal.Parse("25").ToString() => "25"
Decimal.Parse("25.").ToString() => "25"
Decimal.Parse("25.0").ToString() => "25.0"
Decimal.Parse("25.0000").ToString() => "25.0000"
25m.ToString() => "25"
25.000m.ToString() => "25.000"
對於上述所有示例,對Double
執行相同的操作將導致小數點為零( "25"
)。
如果你想要小數點到 2 位小數,很有可能是因為它是貨幣,在這種情況下,這在 95% 的情況下可能沒問題:
Decimal.Parse("25.0").ToString("c") => "$25.00"
或者在 XAML 中,您將使用{Binding Price, StringFormat=c}
在將 XML 發送到亞馬遜的網絡服務時,我遇到了一個需要小數作為小數的情況。 該服務抱怨是因為 Decimal 值(最初來自 SQL Server)作為25.1200
被發送25.1200
拒絕( 25.12
是預期的格式)。
我需要做的就是Decimal.Round(...)
2 個小數位的Decimal.Round(...)
來解決問題,而不管值的來源如何。
// generated code by XSD.exe
StandardPrice = new OverrideCurrencyAmount()
{
TypedValue = Decimal.Round(product.StandardPrice, 2),
currency = "USD"
}
TypedValue
是Decimal
類型,所以我不能只做ToString("N2")
並且需要將它四舍五入並將其保留為decimal
。
這是一個顯示不同格式的小 Linqpad 程序:
void Main()
{
FormatDecimal(2345.94742M);
FormatDecimal(43M);
FormatDecimal(0M);
FormatDecimal(0.007M);
}
public void FormatDecimal(decimal val)
{
Console.WriteLine("ToString: {0}", val);
Console.WriteLine("c: {0:c}", val);
Console.WriteLine("0.00: {0:0.00}", val);
Console.WriteLine("0.##: {0:0.##}", val);
Console.WriteLine("===================");
}
結果如下:
ToString: 2345.94742
c: $2,345.95
0.00: 2345.95
0.##: 2345.95
===================
ToString: 43
c: $43.00
0.00: 43.00
0.##: 43
===================
ToString: 0
c: $0.00
0.00: 0.00
0.##: 0
===================
ToString: 0.007
c: $0.01
0.00: 0.01
0.##: 0.01
===================
如果值為 0,您很少會想要一個空字符串。
decimal test = 5.00;
test.ToString("0.00"); //"5.00"
decimal? test2 = 5.05;
test2.ToString("0.00"); //"5.05"
decimal? test3 = 0;
test3.ToString("0.00"); //"0.00"
評分最高的答案不正確,浪費了(大多數)人的 10 分鍾時間。
Mike M. 的答案在 .NET 上對我來說是完美的,但在撰寫本文時,.NET Core 沒有decimal.Round
方法。
在 .NET Core 中,我不得不使用:
decimal roundedValue = Math.Round(rawNumber, 2, MidpointRounding.AwayFromZero);
一個hacky方法,包括轉換為字符串,是:
public string FormatTo2Dp(decimal myNumber)
{
// Use schoolboy rounding, not bankers.
myNumber = Math.Round(myNumber, 2, MidpointRounding.AwayFromZero);
return string.Format("{0:0.00}", myNumber);
}
這些都沒有完全滿足我的需要,強制2 dp並四舍五入為0.005 -> 0.01
強制 2 dp 需要將精度提高 2 dp 以確保我們至少有 2 dp
然后四舍五入以確保我們沒有超過 2 dp
Math.Round(exactResult * 1.00m, 2, MidpointRounding.AwayFromZero)
6.665m.ToString() -> "6.67"
6.6m.ToString() -> "6.60"
評分最高的答案描述了一種格式化十進制值的字符串表示形式的方法,並且它有效。
但是,如果您確實想將保存的精度更改為實際值,則需要編寫如下內容:
public static class PrecisionHelper
{
public static decimal TwoDecimalPlaces(this decimal value)
{
// These first lines eliminate all digits past two places.
var timesHundred = (int) (value * 100);
var removeZeroes = timesHundred / 100m;
// In this implementation, I don't want to alter the underlying
// value. As such, if it needs greater precision to stay unaltered,
// I return it.
if (removeZeroes != value)
return value;
// Addition and subtraction can reliably change precision.
// For two decimal values A and B, (A + B) will have at least as
// many digits past the decimal point as A or B.
return removeZeroes + 0.01m - 0.01m;
}
}
一個示例單元測試:
[Test]
public void PrecisionExampleUnitTest()
{
decimal a = 500m;
decimal b = 99.99m;
decimal c = 123.4m;
decimal d = 10101.1000000m;
decimal e = 908.7650m
Assert.That(a.TwoDecimalPlaces().ToString(CultureInfo.InvariantCulture),
Is.EqualTo("500.00"));
Assert.That(b.TwoDecimalPlaces().ToString(CultureInfo.InvariantCulture),
Is.EqualTo("99.99"));
Assert.That(c.TwoDecimalPlaces().ToString(CultureInfo.InvariantCulture),
Is.EqualTo("123.40"));
Assert.That(d.TwoDecimalPlaces().ToString(CultureInfo.InvariantCulture),
Is.EqualTo("10101.10"));
// In this particular implementation, values that can't be expressed in
// two decimal places are unaltered, so this remains as-is.
Assert.That(e.TwoDecimalPlaces().ToString(CultureInfo.InvariantCulture),
Is.EqualTo("908.7650"));
}
您可以使用 system.globalization 將數字格式化為任何所需的格式。
例如:
system.globalization.cultureinfo ci = new system.globalization.cultureinfo("en-ca");
如果你有一個decimal d = 1.2300000
並且你需要把它修整到 2 個小數位,那么它可以像這樣打印d.Tostring("F2",ci);
其中 F2 是格式化為 2 個小數位的字符串,而 ci 是語言環境或文化信息。
有關更多信息,請查看此鏈接
http://msdn.microsoft.com/en-us/library/dwhawy9k.aspx
https://msdn.microsoft.com/en-us/library/dwhawy9k%28v=vs.110%29.aspx
此鏈接詳細說明了您可以如何處理問題以及如果您想了解更多信息可以做什么。 為簡單起見,您要做的是
double whateverYouWantToChange = whateverYouWantToChange.ToString("F2");
如果你想要一個貨幣,你可以通過輸入“C2”而不是“F2”來讓它更容易
最適用的解決方案是
decimalVar.ToString("#.##");
Double Amount = 0;
string amount;
amount=string.Format("{0:F2}", Decimal.Parse(Amount.ToString()));
如果您只需要保留 2 個小數位(即截掉所有其余的小數位):
decimal val = 3.14789m;
decimal result = Math.Floor(val * 100) / 100; // result = 3.14
如果您只需要保留 3 個小數位:
decimal val = 3.14789m;
decimal result = Math.Floor(val * 1000) / 1000; // result = 3.147
var arr = new List<int>() { -4, 3, -9, 0, 4, 1 };
decimal result1 = arr.Where(p => p > 0).Count();
var responseResult1 = result1 / arr.Count();
decimal result2 = arr.Where(p => p < 0).Count();
var responseResult2 = result2 / arr.Count();
decimal result3 = arr.Where(p => p == 0).Count();
var responseResult3 = result3 / arr.Count();
Console.WriteLine(String.Format("{0:#,0.000}", responseResult1));
Console.WriteLine(String.Format("{0:#,0.0000}", responseResult2));
Console.WriteLine(String.Format("{0:#,0.00000}", responseResult3));
你可以放任意多個 0。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.