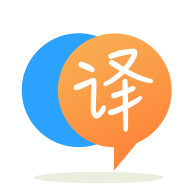
[英]How do I write a function that returns a list of dictionaries in Python?
[英]How do I write a function that returns another function?
在 Python 中,我想編寫一個返回另一個函數的函數make_cylinder_volume(r)
。 返回的函數應該可以使用參數h
調用,並返回高度為h
和半徑為r
的圓柱體的體積。
我知道如何從 Python 中的函數返回值,但如何返回另一個函數?
試試這個,使用 Python:
import math
def make_cylinder_volume_func(r):
def volume(h):
return math.pi * r * r * h
return volume
像這樣使用它,例如radius=10
和height=5
:
volume_radius_10 = make_cylinder_volume_func(10)
volume_radius_10(5)
=> 1570.7963267948967
請注意,返回一個函數是在函數內部定義一個新函數並在最后返回它的簡單問題——注意為每個函數傳遞適當的參數。 僅供參考,從另一個函數返回一個函數的技術稱為柯里化。
使用 lambda,也稱為匿名函數,您可以將make_cylinder_volume_func
中的volume
函數抽象為一行。 與 Óscar López 的回答沒有什么不同,使用 lambda 的解決方案在某種意義上仍然“更實用”。
這是使用 lambda 表達式編寫接受的答案的方法:
import math
def make_cylinder_volume_fun(r):
return lambda h: math.pi * r * r * h
然后像調用任何其他咖喱函數一樣調用:
volume_radius_1 = make_cylinder_volume_fun(1)
volume_radius_1(1)
=> 3.141592653589793
只是想指出你可以用 pymonad 做到這一點
import pymonad
@pymonad.curry
def add(a, b):
return a + b
add5 = add(5)
add5(4)
9
我知道我來晚了,但我想你可能會發現這個解決方案很有趣。
from math import pi
from functools import partial
def cylinder_volume(r, h):
return pi * r * r * h
make_cylinder_with_radius_2 = partial(cylinder_volume, 2)
make_cylinder_with_height_3 = partial(cylinder_volume, h=3)
print(cylinder_volume(2, 3)) # 37.6991118431
print(make_cylinder_with_radius_2(3)) # 37.6991118431
print(make_cylinder_with_height_3(2)) # 37.6991118431
這是有關partial
工作原理的文檔。
這是一個巨大的例子,它涵蓋了一個函數中的許多單個多參數情況
def maths(var='NA'):
if var.lower() == 'add':
def add(*args):
return "Sum is : "+str(sum(args))
return add
elif var.lower() == 'substract':
def substract(a,b):
if a>b:
return "Difference is : "+str(a-b)
else:
return "Difference is : "+str(b-a)
return substract
elif var.lower() == 'multiply':
def multiply(*args):
temp = 1
for x in args:
temp = temp*x
return "multiplication is : "+str(temp)
return multiply
elif var.lower() == 'divide':
def divide(a,b):
return "Division is : "+str(a/b)
return divide
else:
print("Please choose one of given operations: 'add','substract','multiply','divide'")
這里首先調用需要運算的maths函數,然后使用返回的函數進行實際計算
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.