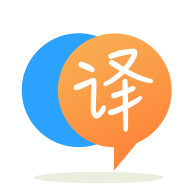
[英]Sorting a List<KeyValuePair<int, string>> Uppercase and Lowercase
[英]c# Sorting a List<KeyValuePair<int, string>>
在C#中,我想按List<KeyValuePair<int, string>>
中每個字符串的長度對List<KeyValuePair<int, string>>
進行排序。 在Psuedo-Java中,這將是一個匿名的,看起來像:
Collections.Sort(someList, new Comparator<KeyValuePair<int, string>>( {
public int compare(KeyValuePair<int, string> s1, KeyValuePair<int, string> s2)
{
return (s1.Value.Length > s2.Value.Length) ? 1 : 0; //specify my sorting criteria here
}
});
C#中的等價物是使用lambda表達式和Sort
方法:
someList.Sort((x, y) => x.Value.Length.CompareTo(y.Value.Length));
您還可以使用OrderBy
擴展方法。 它的代碼略少,但它增加了更多的開銷,因為它創建了列表的副本而不是將其排序到位:
someList = someList.OrderBy(x => x.Value.Length).ToList();
您可以使用linq調用OrderBy
list.OrderBy(o => o.Value.Length);
有關@Guffa指出要查找Linq和延遲執行的更多信息,基本上它只會在需要時執行。 因此,要立即從此行返回列表,您需要添加.ToList()
,這將使表達式返回列表。
你可以用這個
using System;
using System.Collections.Generic;
class Program
{
static int Compare1(KeyValuePair<string, int> a, KeyValuePair<string, int> b)
{
return a.Key.CompareTo(b.Key);
}
static int Compare2(KeyValuePair<string, int> a, KeyValuePair<string, int> b)
{
return a.Value.CompareTo(b.Value);
}
static void Main()
{
var list = new List<KeyValuePair<string, int>>();
list.Add(new KeyValuePair<string, int>("Perl", 7));
list.Add(new KeyValuePair<string, int>("Net", 9));
list.Add(new KeyValuePair<string, int>("Dot", 8));
// Use Compare1 as comparison delegate.
list.Sort(Compare1);
foreach (var pair in list)
{
Console.WriteLine(pair);
}
Console.WriteLine();
// Use Compare2 as comparison delegate.
list.Sort(Compare2);
foreach (var pair in list)
{
Console.WriteLine(pair);
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.