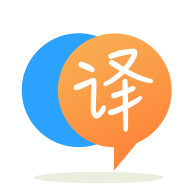
[英]scipy.optimize.minimize, travelling salesman with integer programming
[英]Travelling Salesman in scipy
如何在python中解决旅行商问题? 我没有找到任何库,应该有一种方法使用scipy函数进行优化或其他库。
我的hacky-extremelly-lazy-pythonic强制解决方案是:
tsp_solution = min( (sum( Dist[i] for i in izip(per, per[1:])), n, per) for n, per in enumerate(i for i in permutations(xrange(Dist.shape[0]), Dist.shape[0])) )[2]
其中Dist(numpy.array)是距离矩阵。 如果Dist太大,这将需要永远。
建议?
scipy.optimize
函数的构造不允许直接适应旅行商问题(TSP)。 对于一个简单的解决方案,我推荐2-opt算法,这是一种广为接受的算法,用于解决TSP并且相对简单易用。 这是我的算法实现:
import numpy as np
# Calculate the euclidian distance in n-space of the route r traversing cities c, ending at the path start.
path_distance = lambda r,c: np.sum([np.linalg.norm(c[r[p]]-c[r[p-1]]) for p in range(len(r))])
# Reverse the order of all elements from element i to element k in array r.
two_opt_swap = lambda r,i,k: np.concatenate((r[0:i],r[k:-len(r)+i-1:-1],r[k+1:len(r)]))
def two_opt(cities,improvement_threshold): # 2-opt Algorithm adapted from https://en.wikipedia.org/wiki/2-opt
route = np.arange(cities.shape[0]) # Make an array of row numbers corresponding to cities.
improvement_factor = 1 # Initialize the improvement factor.
best_distance = path_distance(route,cities) # Calculate the distance of the initial path.
while improvement_factor > improvement_threshold: # If the route is still improving, keep going!
distance_to_beat = best_distance # Record the distance at the beginning of the loop.
for swap_first in range(1,len(route)-2): # From each city except the first and last,
for swap_last in range(swap_first+1,len(route)): # to each of the cities following,
new_route = two_opt_swap(route,swap_first,swap_last) # try reversing the order of these cities
new_distance = path_distance(new_route,cities) # and check the total distance with this modification.
if new_distance < best_distance: # If the path distance is an improvement,
route = new_route # make this the accepted best route
best_distance = new_distance # and update the distance corresponding to this route.
improvement_factor = 1 - best_distance/distance_to_beat # Calculate how much the route has improved.
return route # When the route is no longer improving substantially, stop searching and return the route.
以下是正在使用的函数的示例:
# Create a matrix of cities, with each row being a location in 2-space (function works in n-dimensions).
cities = np.random.RandomState(42).rand(70,2)
# Find a good route with 2-opt ("route" gives the order in which to travel to each city by row number.)
route = two_opt(cities,0.001)
这是绘图上显示的近似解决方案路径:
import matplotlib.pyplot as plt
# Reorder the cities matrix by route order in a new matrix for plotting.
new_cities_order = np.concatenate((np.array([cities[route[i]] for i in range(len(route))]),np.array([cities[0]])))
# Plot the cities.
plt.scatter(cities[:,0],cities[:,1])
# Plot the path.
plt.plot(new_cities_order[:,0],new_cities_order[:,1])
plt.show()
# Print the route as row numbers and the total distance travelled by the path.
print("Route: " + str(route) + "\n\nDistance: " + str(path_distance(route,cities)))
如果算法的速度对您很重要,我建议预先计算距离并将它们存储在矩阵中。 这大大减少了收敛时间。
编辑:自定义开始和结束点
对于非圆形路径(一个终止于与其开始的位置不同的位置),编辑路径距离公式
path_distance = lambda r,c: np.sum([np.linalg.norm(c[r[p+1]]-c[r[p]]) for p in range(len(r)-1)])
然后重新排序城市以便使用
new_cities_order = np.array([cities[route[i]] for i in range(len(route))])
使用代码,起始城市被固定为城市中的第一个cities
,而结束城市是可变的。
要使结束城市成为城市中的最后一个cities
,请通过使用代码更改two_opt()
中swap_first
和swap_last
的范围来限制可交换城市的范围
for swap_first in range(1,len(route)-3):
for swap_last in range(swap_first+1,len(route)-1):
要使起始和结束城市变量,而是扩展swap_first
和swap_last
的范围
for swap_first in range(0,len(route)-2):
for swap_last in range(swap_first+1,len(route)):
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.