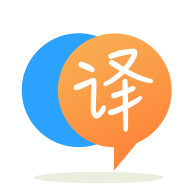
[英]Is there a way to find minimum element in a set in less than O(n) time?
[英]Fastest way to get minimum elements in a list less than a particular element
我最好用一个例子来解释
假设,我有一个列表 [6,3,5,1,4,2]。
从索引 0 开始,查找所有小于(尚未标记)该索引处的值的项目。
Index 0: [6,3,5,1,4,2]
Elements less than 6: 5{3,5,1,4,2}
Visited array: [1 0 0 0 0 0]
Index 1: [6,3,5,1,4,2]
Elements less than 3: 2 {1,2}
Visited array: [1 1 0 0 0 0]
Index 2: [6,3,5,1,4,2]
Elements less than 5: 3 {1,4,2}
Visited array: [1 1 1 0 0 0]
Index 3: [6,3,5,1,4,2]
Elements less than 1: 0 {NULL}
Visited array: [1 1 1 1 0 0]
Index 4: [6,3,5,1,4,2]
Elements less than 4: 1 {2}
Visited array: [1 1 1 1 1 0]
This yields an array as [5 2 3 0 1 0]
目前使用,
def get_diff(lis):
ans=[]
for index,elem in enumerate(lis):
number_of_smaller=len(filter(lambda x:x<elem ,lis[index+1:]))
ans.append(number_of_smaller)
return ans
但是,我有一种感觉,这不会有效率。 我如何使它值得一个巨大的清单? 我闻到前缀和了吗? 谢谢,
您可以简单地在 dict 推导中使用列表推导将项目保留为键,将小于它的项目保留为值(并使用collections.OrderedDict
保留顺序):
>>> from collections import OrderedDict
>>> def get_diff(lis):
... return OrderedDict((item,[i for i in lis if i<item]) for item in lis)
由于您的条件<
没有必要排除项目本身,因为相比之下,删除它的成本大于包含它的成本。
此外,如果您想保留索引,您可以使用enumerate
循环遍历您的列表:
def get_diff(lis):
return OrderedDict((item,index),[i for i in lis if i<item]) for index,item in enumerate(lis))
如果您想计算这些项目的数量,您可以在sum
函数中使用生成器表达式:
>>> from collections import OrderedDict
>>> def get_diff(lis):
... return OrderedDict((item,sum(1 for i in lis if i<item)) for item in lis)
注意:如果您想计算的项目少于此后的任何项目(具有更大的索引),您可以简单地在循环中使用索引:
>>> def get_diff(lis):
... return OrderedDict((item,sum(1 for i in lis[index:] if i<item)) for index,item in enumerate(lis))
...
>>> get_diff(l).values()
[5, 2, 3, 0, 1, 0]
my_list = [6,3,5,1,4,2]
def get_diff(lis):
result = []
for visited, i in enumerate(range(len(lis))):
limit = lis[i]
elem = filter(None, [x if x < limit else None for x in lis][visited + 1:])
result.append(len(elem))
return result
print get_diff(my_list)
#[5, 2, 3, 0, 1, 0]
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.