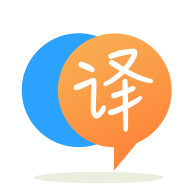
[英]To Find Lowest Common Ancestor in a Tree[Binary] but with multiple Node IN python?
[英]Python find lowest common ancestor of two nodes in a binary tree if not all of these nodes in the tree
我理解如何解决这两个节点必须在二叉树中的问题,但是如果它们不必在树中呢? 如果树中只有一个节点或没有节点,则返回None。
这是我的代码:
# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def lowestCommonAncestor(self, root, p, q):
"""
:type root: TreeNode
:type p: TreeNode
:type q: TreeNode
:rtype: TreeNode
"""
[root,count] = self.findAncestor(root,p,q,0)
if count == 2:
return root
else:
return None
def findAncestor(self,root,p,q,count):
if not root:
return None, count
left,left_count = self.findAncestor(root.left, p, q,count)
right,right_count = self.findAncestor(root.right,p,q,count)
if root == p or root == q:
return root,count+1
if left and right:
return root,count
elif left:
return left,left_count
else:
return right,right_count
但我一直得到错误的答案。 任何人都知道如何根据我的代码修复它? 谢谢!
我们可以计算目标节点数,如果它是2,那么我们知道两个节点都在树中。
class Solution(object):
def lowestCommonAncestor(self, root, p, q):
"""
:type root: TreeNode
:type p: TreeNode
:type q: TreeNode
:rtype: TreeNode
"""
self.count = 0
node = self.find(root, p, q)
return node if self.count == 2 else None
def find(self, node, p, q):
if not node:
return None
if node in (p, q):
self.count += 1
left = self.find(node.left, p, q)
right = self.find(node.right, p, q)
return node if node in (p, q) or left and right else left or right
基于kitt的解决方案,我在lintCode问题578上测试他的解决方案,但它没有通过。 问题发生在计数条件下,应该再检查那些输入的两个节点。 所以我重新设计了一个通过lintcode测试的新解决方案,同时具有更好的读取逻辑。
"""
Definition of TreeNode:
class TreeNode:
def __init__(self, val):
this.val = val
this.left, this.right = None, None
"""
class Solution:
"""
@param: root: The root of the binary tree.
@param: A: A TreeNode
@param: B: A TreeNode
@return: Return the LCA of the two nodes.
"""
count = 0
def lowestCommonAncestor3(self, root, A, B):
result = self.lca(root, A, B)
return result if self.count == 2 else None
def lca(self, root, A, B):
if not root:
return None
for node in [A, B]:
if root == node:
self.count += 1
left = self.lca(root.left, A, B)
right = self.lca(root.right, A, B)
if root in (A, B) or left and right:
return root
if left:
return left
if right:
return right
return None
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.