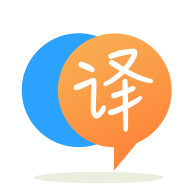
[英]A image converted with canvas fails to be read by python PIL _io.BytesIO
[英]open fp = io.BytesIO(fp.read()) AttributeError: 'str' object has no attribute 'read' in PIL/image.py
我对 Python 有点陌生,尤其是我目前正在使用的 Imaging 库。 我正在使用面部识别代码并在运行 Jessie 的 Raspberry Pi 2 B+ 中运行它。 我正在使用 Opencv 2.4.9 和 Python 2.7。 我目前正在使用的代码一直工作到几分钟前,但现在我不断收到错误消息。 我没有更改代码或更新任何内容。
我尝试过的:我卸载了枕头并安装了不同的版本,但它仍然无法正常工作。
我只是不明白发生了什么变化。 我尝试通过更改变量名称仍然没有效果。
import cv
import cv2
import sys
import os
import datetime
import time
from PIL import Image
import numpy as np
def EuclideanDistance(p, q):
p = np.asarray(p).flatten()
q = np.asarray(q).flatten()
return np.sqrt(np.sum(np.power((p-q),2)))
class EigenfacesModel():
def __init__(self, X=None, y=None, num_components=0):
self.num_components = 0
self.projections = []
self.W = []
self.mu = []
if (X is not None) and (y is not None):
self.compute(X,y)
def compute(self, X, y):
[D, self.W, self.mu] = pca(asRowMatrix(X),y, self.num_components)
# store labels
self.y = y
# store projections
for xi in X:
self.projections.append(project(self.W, xi.reshape(1,-1), self.mu))
def predict(self, X):
minDist = np.finfo('float').max
minClass = -1
Q = project(self.W, X.reshape(1,-1), self.mu)
for i in xrange(len(self.projections)):
dist = EuclideanDistance(self.projections[i], Q)
#print i,dist
if dist < minDist:
minDist = dist
minClass = self.y[i]
print "\nMinimum distance ", minDist
return minClass,minDist
def asRowMatrix(X):
if len(X) == 0:
return np.array([])
mat = np.empty((0, X[0].size), dtype=X[0].dtype)
for row in X:
mat = np.vstack((mat, np.asarray(row).reshape(1,-1)))
return mat
def read_images(filename, sz=None):
c = 0
X,y = [], []
with open(filename) as f:
for line in f:
line = line.rstrip()
im = Image.open(line)
im = im.convert("L")
# resize to given size (if given)
if (sz is None):
im = im.resize((92,112), Image.ANTIALIAS)
X.append(np.asarray(im, dtype=np.uint8))
y.append(c)
c = c+1
print c
return [X,y]
def pca(X, y, num_components=0):
[n,d] = X.shape
print n
if (num_components <= 0) or (num_components>n):
num_components = n
mu = X.mean(axis=0)
X = X - mu
if n>d:
C = np.dot(X.T,X)
[eigenvalues,eigenvectors] = np.linalg.eigh(C)
else:
C = np.dot(X,X.T)
[eigenvalues,eigenvectors] = np.linalg.eigh(C)
eigenvectors = np.dot(X.T,eigenvectors)
for i in xrange(n):
eigenvectors[:,i] = eigenvectors[:,i]/np.linalg.norm(eigenvectors[:,i])
# or simply perform an economy size decomposition
# eigenvectors, eigenvalues, variance = np.linalg.svd(X.T, full_matrices=False)
# sort eigenvectors descending by their eigenvalue
idx = np.argsort(-eigenvalues)
eigenvalues = eigenvalues[idx]
eigenvectors = eigenvectors[:,idx]
# select only num_components
num_components = 25
eigenvalues = eigenvalues[0:num_components].copy()
eigenvectors = eigenvectors[:,0:num_components].copy()
return [eigenvalues, eigenvectors, mu]
def project(W, X, mu=None):
if mu is None:
return np.dot(X,W)
return np.dot(X - mu, W)
def reconstruct(W, Y, mu=None):
if mu is None:
return np.dot(W.T,Y)
return np.dot(W.T,Y) + mu
#if __name__ == "__main__":
def FaceRecognitionWrapper(Database_Address,TestImages_Address):
out_dir = "Output_Directory"
[X,y] = read_images(Database_Address)
y = np.asarray(y, dtype=np.int32)
#print len(X)
model = EigenfacesModel(X[0:], y[0:])
# get a prediction for the first observation
[X1,y1] = read_images(TestImages_Address)
y1 = np.asarray(y1, dtype=np.int32)
OutputFile = open("Output.txt",'a')
for i in xrange(len(X1)):
predicted,difference = model.predict(X1[i])
predicted1 = int(predicted/10) + 1
if difference <= 1000:
print i+1 , "th image was recognized as individual" , predicted+1
OutputFile.write(str(predicted1))
OutputFile.write("\n")
else:
os.chdir(out_dir)
print i+1,"th image could not be recognized. Storing in error folder."
errorImage = Image.fromarray(X1[i])
current_time = datetime.datetime.now().time()
error_img_name=current_time.isoformat()+'.png'
errorImage.save(error_img_name)
os.chdir('..')
OutputFile.close()
#Create Model Here
cascPath = '/home/pi/opencv-2.4.9/data/haarcascades/haarcascade_frontalface_default.xml'
faceCascade = cv2.CascadeClassifier(cascPath)
Test_Files = []
video_capture = cv2.VideoCapture(0)
i = 0
while True:
# Capture frame-by-frame
ret, frame = video_capture.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = faceCascade.detectMultiScale(
gray,
scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30),
flags=cv2.cv.CV_HAAR_SCALE_IMAGE
)
# Draw a rectangle around the faces
for (x, y, w, h) in faces:
dummy_image = frame
cv2.rectangle(dummy_image, (x, y), (x+w, y+h), (0, 255, 0), 2)
dummy_image=dummy_image[y:y+h, x:x+w]
dirname = 'detection_output'
os.chdir(dirname)
current_time = datetime.datetime.now().time()
final_img_name=current_time.isoformat()+'.png'
Test_Files.append(final_img_name)
dummy_image = cv2.cvtColor(dummy_image, cv2.COLOR_BGR2GRAY)
cv2.imwrite(final_img_name,dummy_image)
os.chdir('..')
# Display the resulting frame
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
i = i + 1
if i % 20 == 0:
dirname = 'detection_output'
os.chdir(dirname)
TestFile = open('CameraFeedFaces.txt',"w")
for Files in Test_Files:
TestFile.write(os.getcwd()+"/"+Files+"\n")
TestFile.close()
os.chdir("..")
#Call testing.py
FaceRecognitionWrapper("/home/pi/train_faces/temp.txt",os.getcwd()+"/detection_output/CameraFeedFaces.txt")
#Open Output File and Copy in a separate folder where distance greater than threshold
#Then delete all the files in the folder
break
# When everything is done, release the capture
video_capture.release()
cv2.destroyAllWindows()
这是回溯:
Traceback (most recent call last):
File "hel.py", line 213, in <module>
FaceRecognitionWrapper("/home/pi/train_faces/temp.txt",os.getcwd()+"/detection_output/CameraFeedFaces.txt")
File "hel.py", line 127, in FaceRecognitionWrapper
[X,y] = read_images(Database_Address)
File "hel.py", line 68, in read_images
im = Image.open(line)
File "/usr/local/lib/python2.7/dist-packages/PIL/Image.py", line 2277, in open
fp = io.BytesIO(fp.read())
AttributeError: 'str' object has no attribute 'read'
我在某处读过它,如果我尝试编辑 im = Image.open(open(line,'rb')) 我得到这个错误而不是前一个
Traceback (most recent call last):
File "hel.py", line 208, in <module>
FaceRecognitionWrapper("/home/pi/train_faces/temp.txt",os.getcwd()+"/detection_output/CameraFeedFaces.txt")
File "hel.py", line 122, in FaceRecognitionWrapper
[X,y] = read_images(Database_Address)
File "hel.py", line 63, in read_images
im = Image.open(open(line,'rb'))
IOError: [Errno 2] No such file or directory: ''
您的主题行令人困惑。 你修复了这个问题,然后暴露了一个不同的错误。
IOError: [Errno 2] No such file or directory: ''
该消息显示您正在尝试打开一个没有名称的文件,这意味着您正在阅读的输入文件至少有一个空行。 如果该文件可以有空行,请跳过以下内容:
def read_images(filename, sz=None):
c = 0
X,y = [], []
with open(filename) as f:
for line in f:
line = line.rstrip()
if line:
im = Image.open(open(line,'rb'))
im = im.convert("L"
# resize to given size (if given)
if (sz is None):
im = im.resize((92,112), Image.ANTIALIAS)
X.append(np.asarray(im, dtype=np.uint8))
y.append(c)
c = c+1
否则,您会遇到合法的错误,您应该捕获并处理异常。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.