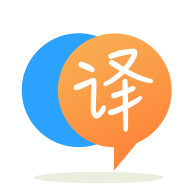
[英]How can I find the longest contiguous subsequence in a rising sequence in Python?
[英]Python: Longest Plateau Problem: to find the length and location of the longest contiguous sequence of equal values
问题是在Sedgewick Wayne的Python书中解决以下问题:
给定一个整数数组,组成一个程序,找到相等值的最长连续序列的长度和位置,其中此序列之前和之后的元素值较小。
我试过这个问题,遇到了一些问题。
这是我的代码:
import sys
import stdio
# Ask the user input for the list of integers
numList = list(sys.argv[1])
maxcount = 0
value = None
location = None
i = 1
while i < len(numList) - 1:
resList = []
count = 0
# If i > i-1, then we start taking i into the resList
if numList[i] > numList[i - 1]:
# start counting
resList += [numList[i]]
# Iterating through the rest of the numbers
j = i + 1
while j < len(numList):
# If the j element equals the i, then append it to resList
if numList[i] == numList[j]:
resList += [numList[j]]
j += 1
elif numList[i] < numList[j]:
# if j element is greater than i, break out the loop
i = j
break
else:
# if j element is smaller than i, count equals length of resList
count = len(resList)
if count > maxcount:
maxcount = count
value = resList[1]
location = i
i = j
else:
# if not greater than the previous one, increment by 1
i += 1
stdio.writeln("The longest continuous plateau is at location: " + str(location))
stdio.writeln("Length is: " + str(maxcount))
stdio.writeln("Number is: " + str(value))
结果显示:
python exercise1_4_21.py 553223334445554
The longest continuous plateau is at location: 11
Length is: 3
Number is: 5
python exercise1_4_21.py 1234567
The longest continuous plateau is at location: None
Length is: 0
Number is: None
但不知何故,如果给出的列表的格式是一组连续整数大于前一个整数,但是这个组结束列表后面没有整数,我的程序根本就没有结束....
exercise1_4_21.py 11112222111444
Traceback (most recent call last):
File "exercise1_4_21.py", line 32, in <module>
if numList[i] == numList[j]:
KeyboardInterrupt
exercise1_4_21.py 111222211112223333
Traceback (most recent call last):
File "exercise1_4_21.py", line 25, in <module>
if numList[i] > numList[i - 1]:
KeyboardInterrupt
不太确定逻辑错误在哪里...非常感谢你的帮助和善意!
似乎你使解决方案过于复杂(正确选择的关键案例)。
它只需要单次运行列表。
def maxplat(l):
if (len(l)==0):
return 0, 0
start, leng = 0, 1
maxlen, maxstart = 0, 1
for i in range(1, len(l) + 1):
if (i == len(l)) or (l[i] < l[i-1]):
if (leng > maxlen):
maxlen, maxstart = leng, start
elif (l[i] == l[i-1]):
leng += 1
else:
start, leng = i, 1
return maxlen, maxstart
#test cases
print(maxplat([])) #empty case
print(maxplat([3])) #single element
print(maxplat([3,2,4,4,2,5,5,5,3])) #simple case
print(maxplat([3,2,4,4,2,5,5,5,6])) #up after long run
print(maxplat([3,2,4,4,2,5,5,5])) #run at the end
print(maxplat([3,3,3,3,2,4,4,2])) #run at the start
>>>
(0, 0)
(1, 0)
(3, 5)
(2, 2)
(3, 5)
(4, 0)
您需要在代码中添加额外的检查才能退出。
if j == len(numList):
maxcount = len(resList)
value = resList[1]
location = i
break
在你的代码中,它看起来像这样:
import sys
import stdio
# Ask the user input for the list of integers
numList = list(sys.argv[1])
maxcount = 0
value = None
location = None
i = 1
while i < len(numList) - 1:
resList = []
count = 0
# If i > i-1, then we start taking i into the resList
if numList[i] > numList[i - 1]:
# start counting
resList += [numList[i]]
# Iterating through the rest of the numbers
j = i + 1
while j < len(numList):
# If the j element equals the i, then append it to resList
if numList[i] == numList[j]:
resList += [numList[j]]
j += 1
elif numList[i] < numList[j]:
# if j element is greater than i, break out the loop
i = j
break
else:
# if j element is smaller than i, count equals length of resList
count = len(resList)
if count > maxcount:
maxcount = count
value = resList[1]
location = i
i = j
#EXTRA CHECK HERE
if j == len(numList):
maxcount = len(resList)
value = resList[1]
location = i
break
else:
# if not greater than the previous one, increment by 1
i += 1
stdio.writeln("The longest continuous plateau is at location: " + str(location))
stdio.writeln("Length is: " + str(maxcount))
stdio.writeln("Number is: " + str(value))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.