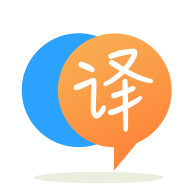
[英]Adding line plot using second axis to bar plot using custom dates as x ticks matplotlib
[英]Plot bar and line using both right and left axis in Matplotlib
给一个dataframe如下:
date gdp tertiary_industry gdp_growth tertiary_industry_growth
0 2015/3/31 3768 2508 10.3 11.3
1 2015/6/30 8285 5483 10.9 12.0
2 2015/9/30 12983 8586 11.5 12.7
3 2015/12/31 18100 12086 10.5 13.2
4 2016/3/31 4118 2813 13.5 14.6
5 2016/6/30 8844 6020 13.3 14.3
6 2016/9/30 14038 9513 14.4 13.9
7 2016/12/31 19547 13557 16.3 13.3
8 2017/3/31 4692 3285 13.3 12.4
9 2017/6/30 9891 6881 12.9 12.5
10 2017/9/30 15509 10689 12.7 12.3
11 2017/12/31 21503 15254 14.8 12.7
12 2018/3/31 4954 3499 12.4 11.3
13 2018/6/30 10653 7520 12.9 12.4
14 2018/9/30 16708 11697 13.5 13.0
15 2018/12/31 22859 16402 14.0 13.2
16 2019/3/31 5508 3983 13.5 13.9
17 2019/6/30 11756 8556 10.2 13.4
18 2019/9/30 17869 12765 10.2 14.8
19 2019/12/31 23629 16923 11.6 15.2
20 2020/3/31 5229 3968 11.9 14.9
我已应用以下代码为gdp
和tertiary_industry
绘制条形 plot 。
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import matplotlib.ticker as ticker
import matplotlib.style as style
style.available
style.use('fivethirtyeight')
from pylab import rcParams
plt.rcParams["figure.figsize"] = (20, 10)
plt.rcParams['font.sans-serif']=['SimHei']
plt.rcParams['axes.unicode_minus']=False
import matplotlib
matplotlib.matplotlib_fname()
plt.rcParams.update({'font.size': 25})
colors = ['#c23531','#2f4554', '#61a0a8', '#d48265', '#91c7ae','#749f83', '#ca8622', '#bda29a', '#6e7074', '#546570', '#c4ccd3']
df = df.sort_values(by = 'date')
df['date'] = pd.to_datetime(df['date']).dt.to_period('M')
df = df.set_index('date')
df.columns
cols = ['gdp', 'tertiary_industry']
df[cols] = df[cols].apply(pd.to_numeric, errors='coerce')
color_dict = dict(zip(cols, colors))
plt.figure(figsize=(20, 10))
df[cols].plot(color=[color_dict.get(x, '#333333') for x in df.columns], kind='bar', width=0.8)
plt.xticks(rotation=45)
plt.xlabel("")
plt.ylabel("million dollar")
fig = plt.gcf()
plt.show()
plt.draw()
fig.savefig("./gdp.png", dpi=100, bbox_inches = 'tight')
plt.clf()
上述代码中的 output:
现在我想使用线型和右轴在同一个 plot 上绘制百分比值gdp_growth
和tertiary_industry_growth
。
请注意,我想使用代码中自定义颜色列表中的 colors 而不是默认颜色。
我怎么能根据上面的代码做到这一点? 非常感谢您的帮助。
这就是我要做的:
#convert to datetime
df['date'] = pd.to_datetime(df['date']).dt.to_period('M')
cols = ['gdp', 'tertiary_industry']
colors = ['#c23531','#2f4554', '#61a0a8', '#d48265', '#91c7ae','#749f83', '#ca8622', '#bda29a', '#6e7074', '#546570', '#c4ccd3']
df[cols] = df[cols].apply(pd.to_numeric, errors='coerce')
# modify color_dict here:
color_dict = dict(zip(cols, colors))
# initialize an axis instance
fig, ax = plt.subplots(figsize=(10,6))
# plot on new instance
df.plot.bar(y=cols,ax=ax,
color=[color_dict.get(x, '#333333') for x in cols])
# create a twinx axis
ax1 = ax.twinx()
# plot the other two columns on this axis
df.plot.line(y=['gdp_growth','tertiary_industry_growth'], ax=ax1,
color=[color_dict.get(x, '#333333') for x in line_cols])
ax.set_xticklabels(df['date'])
# set y-axes labels:
ax.set_ylabel('Million Dollar')
ax1.set_ylabel('%')
# set x-axis label
ax.set_xlabel('Quarter')
plt.show()
Output:
如果您将上述代码中的两个colors=[...]
替换为原始color=[color_dict.get(x, '#333333') for x in df.columns]
,您将得到
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.