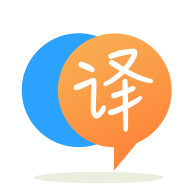
[英]Fastest way to index an element by the first element of a tuple in a list of tuples in python
[英]What is the fastest way to find the average for a list of tuples in Python, each tuple containing a pair of namedtuples?
import numpy as numpy
from collections import namedtuple
from random import random
Smoker = namedtuple("Smoker", ["Female","Male"])
Nonsmoker = namedtuple("Nonsmoker", ["Female","Male"])
LST = [(Smoker(random(),random()),Nonsmoker(random(),random())) for i in range(100)]
所以我有一个很长的列表,其元素是元组。 每个元组包含一对命名元组。 找到此列表的平均值的最快方法是什么? 理想情况下,结果仍然是相同的结构,即(Smoker(Female=w,Male=x),Nonsmoker(Female=y,Male=z))
..
grizzly = Smoker(np.mean([a.Female for a,b in LST]),np.mean([a.Male for a,b in LST]))
panda = Nonmoker(np.mean([b.Female for a,b in LST]),np.mean([b.Male for a,b in LST]))
result = (grizzly, panda)
np.mean
必须将列表转换为数组,这需要时间。 Python sum
节省时间:
In [6]: %%timeit
...: grizzly = Smoker(np.mean([a.Female for a,b in LST]),np.mean([a.Male for
...: a,b in LST]))
...: panda = Nonsmoker(np.mean([b.Female for a,b in LST]),np.mean([b.Male for
...: a,b in LST]))
...: result = (grizzly, panda)
...:
...:
158 µs ± 597 ns per loop (mean ± std. dev. of 7 runs, 10000 loops each)
In [9]: %%timeit
...: n=len(LST)
...: grizzly = Smoker(sum([a.Female for a,b in LST])/n,sum([a.Male for a,b in
...: LST])/n)
...: panda = Nonsmoker(sum([b.Female for a,b in LST])/n,sum([b.Male for a,b i
...: n LST])/n)
...: result = (grizzly, panda)
...:
...:
46.2 µs ± 37.4 ns per loop (mean ± std. dev. of 7 runs, 10000 loops each)
两者都产生相同的result
(在一个小的 epsilon 内):
In [8]: result
Out[8]:
(Smoker(Female=0.5383695316982974, Male=0.5493854404111675),
Nonsmoker(Female=0.4913454565011218, Male=0.47143788469638825))
如果您可以在一个数组中收集值,可能是 (n,4) 形状,那么平均值将很快。 一次计算它可能不值得 -
In [11]: M = np.array([(a.Female, a.Male, b.Female, b.Male) for a,b in LST])
In [12]: np.mean(M, axis=0)
Out[12]: array([0.53836953, 0.54938544, 0.49134546, 0.47143788])
In [13]: timeit M = np.array([(a.Female, a.Male, b.Female, b.Male) for a,b in LST])
128 µs ± 1.22 µs per loop (mean ± std. dev. of 7 runs, 10000 loops each)
In [14]: timeit np.mean(M, axis=0)
21.9 µs ± 371 ns per loop (mean ± std. dev. of 7 runs, 10000 loops each)
由于命名元组可以像常规元组一样访问,我们可以直接从LST
创建一个数组:
In [16]: np.array(LST).shape
Out[16]: (100, 2, 2)
In [17]: np.array(LST).mean(axis=0)
Out[17]:
array([[0.53836953, 0.54938544],
[0.49134546, 0.47143788]])
但时机并不令人鼓舞:
In [18]: timeit np.array(LST).mean(axis=0)
1.26 ms ± 7.92 µs per loop (mean ± std. dev. of 7 runs, 1000 loops each)
我还可以从您的列表中创建一个结构化数组 - 使用嵌套的 dtypes:
In [26]: dt = np.dtype([('Smoker', [('Female','f'),('Male','f')]),('Nonsmoker',[
...: ('Female','f'),('Male','f')])])
In [27]: M=np.array(LST,dt)
In [28]: M['Smoker']['Female'].mean()
Out[28]: 0.53836954
奇怪的是时机相对较好:
In [29]: timeit M=np.array(LST,dt)
40.6 µs ± 243 ns per loop (mean ± std. dev. of 7 runs, 10000 loops each)
但是我必须分别取每个平均值,否则先将其转换为非结构化数组。
我可以使用view
或recfunctions
实用程序从结构化数组中创建一个 (n,4) 浮点数组:
In [53]: M1 = M.view([('f0','f',(4,))])['f0']
In [54]: M1.shape
Out[54]: (100, 4)
In [55]: M2=rf.structured_to_unstructured(M)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.