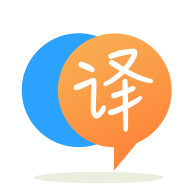
[英]How to pass a python function to a cython function that accepts a function as a parameter?
[英]Assistance with a python function that accepts an array as a parameter
我正在请求 HW 分配的帮助,该分配确定一组名称的 BMI,并输出该名称是否体重不足、正常体重或超重。 到目前为止,这是我的程序,我遇到问题的部分是最后的函数。
#function to calculate bmi
def bmi(weight, height):
return weight * 703 / height ** 2
name_list = ['Spiderman', 'Ironman', 'Black Widow', 'Hulk', 'Thanos', 'Shuri']
#prompting the user for the height of the individuals
heights = []
for name in name_list:
heights.append(int(input(f"Please input the height in inches for {name}: ")))
#prompting the user for the weight of the individuals
weights = []
for name in name_list:
weights.append(int(input(f"Please input the weight in pounds for {name}: ")))
#outputting the BMI for each individual
for i, name in enumerate(name_list):
print(f"The BMI for {name} is {bmi(weights[i], heights[i])}.")
#appending the BMI to a new list
bmi_list = []
for i, name in enumerate(name_list):
bmi_list.append((bmi(weights[i], heights[i])))
#function to calculate if the person is underweight, normal weight, or overweight.
#BMI of <= 18 is underweight, BMI of >= 19 and <=25 is normal and BMI of >26 is overweight
def calc(bmi_list):
for i in bmi_list:
if i <= 18:
print(f"{name} is underweight.")
if i >= 19 and i <= 25:
print(f"{name} is normal weight.")
if i >= 26:
print(f"{name} is overweight.")
calc(bmi_list)
最后一个函数的输出是
Shuri is normal weight.
Shuri is normal weight.
Shuri is normal weight.
Shuri is normal weight.
Shuri is underweight.
Shuri is underweight.
而我需要它像这样输出:
Spiderman is normal weight.
Ironman is normal weight.
Black Widow is normal weight.
Hulk is normal weight.
Thanos is underweight.
Shuri is underweight.
您需要遍历name_list
而不仅仅是使用变量name
(它具有for i, name
循环中剩下的最后一个值)。 您可以使用zip()
将两个列表一起循环。
def calc(name_list, bmi_list):
for i, name in zip(bmi_list, name_list):
if i <= 18:
print(f"{name} is underweight.")
elif i <= 25:
print(f"{name} is normal weight.")
else:
print(f"{name} is overweight.")
calc(name_list, bmi_list)
您还应该将elif and
else` 用于互斥条件。
不为每个属性设置单独的列表通常更容易。 相反,请列出元组或字典,以便将所有相关信息放在一起。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.