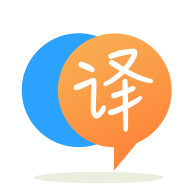
[英]Python - Concatenate or stack more than two arrays with different shape
[英]Concatenate arrays with different shape duplicating values
我有一个形状为: (15,2)
的数组。 我还有另一个带有值的数组: [0, 3, 5]
。
我想在第一个数组中使用第二个数组中的值创建另一列,其中前 5 行的值为 0,第 6-10 行的值为 3,最后 5 行的值为 5。
像这样:
[0,
0,
0,
0,
0,
3,
3,
3,
3,
3,
5,
5,
5,
5,
5]
是否有任何 numpy 方法可以做到这一点?
谢谢!
您可以使用 numpy 的内置repeat
和stack
ing:
a = np.zeros((15,2))
b = np.array([0,3,5])
np.hstack((a, np.repeat(b,5)[:,None]))
output:
[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]
[0. 0. 3.]
[0. 0. 3.]
[0. 0. 3.]
[0. 0. 3.]
[0. 0. 3.]
[0. 0. 5.]
[0. 0. 5.]
[0. 0. 5.]
[0. 0. 5.]
[0. 0. 5.]]
使用np.concatenate
。
a = [0, 0, 0, 0, 0, 3, 3, 3, 3, 3, 5, 5, 5, 5, 5] # your list of [0, 3, 5]
a = np.array([a]).T # [ [0], [0], [0], ..., [5] ] the "vector" array
然后简单地
b = np.array([[i, i +1] for i in range(15)]) # some example 15x2 array
print(np.concatenate((b, a), axis=1))
output 是
array([[ 0, 1, 0],
[ 1, 2, 0],
[ 2, 3, 0],
[ 3, 4, 0],
[ 4, 5, 0],
[ 5, 6, 3],
[ 6, 7, 3],
[ 7, 8, 3],
[ 8, 9, 3],
[ 9, 10, 3],
[10, 11, 5],
[11, 12, 5],
[12, 13, 5],
[13, 14, 5],
[14, 15, 5]])
rand = np.random.random((15,2)) # shape is (15,2)
vals = np.array([0,3,5]) # shape is (3,)
res = np.concatenate(([np.full((rand.shape[0]//vals.shape[0],), val) for val in vals]), axis=0)
res
output:
array([0, 0, 0, 0, 0, 3, 3, 3, 3, 3, 5, 5, 5, 5, 5])
最后,
final = np.hstack((rand, res.reshape(15,1)))
final
output:
array([[0.29759807, 0.60548479, 0. ],
[0.61242249, 0.46456274, 0. ],
[0.86172011, 0.2963868 , 0. ],
[0.91728575, 0.36366023, 0. ],
[0.56488556, 0.82130321, 0. ],
[0.59482141, 0.46148353, 3. ],
[0.7762271 , 0.25415058, 3. ],
[0.09176551, 0.9687253 , 3. ],
[0.06473259, 0.34686598, 3. ],
[0.69542414, 0.15540001, 3. ],
[0.02880707, 0.23169327, 5. ],
[0.90004256, 0.22145591, 5. ],
[0.61596969, 0.46807342, 5. ],
[0.02263769, 0.68522023, 5. ],
[0.81777274, 0.58145853, 5. ]])
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.