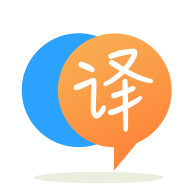
[英]Python Count occurrences of a substring in pandas by row appending distinct string as column
[英]Counting distinct substring occurrences in column for every row in Pyspark?
我的数据集看起来像这样,我在col1
和col2
有一组逗号分隔的字符串值,而col3
是连接在一起的两列。
+===========+========+===========
|col1 |col2 |col3
+===========+========+===========
|a,b,c,d |a,c,d |a,b,c,d,a,c,d
|e,f,g |f,g,h |e,f,g,f,g,h
+===========+========+===========
基本上,我想要做的是获取col3
以逗号分隔的所有值,并在另一列中附加每个值及其计数。
基本上,我试图在col4
获得这种输出:
+===========+========+==============+======================
|col1 |col2 |col3 |col4
+===========+========+==============+======================
|a,b,c,d |a,c,d |a,b,c,d,a,c,d |a: 2, b: 1, c: 2, d: 2
|e,f,g |f,g,h |e,f,g,f,g,h |e: 1, f: 2, g: 2, h: 1
+===========+========+==============+======================
我已经想出了如何将列连接在一起以到达col3
,但是我在到达col4
时遇到了一些麻烦。 这是我离开的地方,我有点不确定从哪里开始:
from pyspark.sql.functions import concat, countDistinct
df = df.select(concat(df.col1, df.col2).alias('col3'), '*')
df.agg(countDistinct('col3')).show()
+---------------------+ |count(DISTINCT col3)| +---------------------+ | 2| +---------------------+
问题:我如何动态计算col3
由逗号分隔的子字符串,并制作最后一列,显示数据集中所有行的每个子字符串的频率?
这是使用 udfs 执行此操作的一种方法。 首先进行数据生成。
from pyspark.sql.types import StringType, StructType, StructField
from pyspark.sql.functions import concat_ws, udf
data = [("a,b,c,d", "a,c,d", "a,b,c,d,a,c,d"),
("e,f,g", "f,g,h", "e,f,g,f,g,h")
]
schema = StructType([
StructField("col1",StringType(),True),
StructField("col2",StringType(),True),
StructField("col3",StringType(),True),
])
df = spark.createDataFrame(data=data,schema=schema)
然后使用一些原生的 python 函数,如 Counter 和 json 来完成任务。
from collections import Counter
import json
@udf(StringType())
def count_occurances(row):
return json.dumps(dict(Counter(row.split(','))))
df.withColumn('concat', concat_ws(',', df.col1, df.col2, df.col3))\
.withColumn('counts', count_occurances('concat')).show(2, False)
结果是
+-------+-----+-------------+---------------------------+--------------------------------+
|col1 |col2 |col3 |concat |counts |
+-------+-----+-------------+---------------------------+--------------------------------+
|a,b,c,d|a,c,d|a,b,c,d,a,c,d|a,b,c,d,a,c,d,a,b,c,d,a,c,d|{"a": 4, "b": 2, "c": 4, "d": 4}|
|e,f,g |f,g,h|e,f,g,f,g,h |e,f,g,f,g,h,e,f,g,f,g,h |{"e": 2, "f": 4, "g": 4, "h": 2}|
+-------+-----+-------------+---------------------------+--------------------------------+
此解决方案比使用 udf 稍微复杂一些,但由于缺少 udf,性能可能更高。 这个想法是连接三个字符串列并分解它们。 为了知道每个分解的行来自哪里,我们添加了一个索引。 双重分组将帮助我们获得所需的结果。 最后,我们将结果连接回原始帧以获得所需的模式。
from pyspark.sql.functions import concat_ws, monotonically_increasing_id, split, explode, collect_list
df = df.withColumn('index', monotonically_increasing_id())
df.join(
df
.withColumn('concat', concat_ws(',', df.col1, df.col2, df.col3))\
.withColumn('arr_col', split('concat', ','))\
.withColumn('explode_col', explode('arr_col'))\
.groupBy('index', 'explode_col').count()\
.withColumn('concat_counts', concat_ws(':', 'explode_col', 'count'))\
.groupBy('index').agg(concat_ws(',', collect_list('concat_counts')).alias('grouped_counts')), on='index').show()
结果是
+-----------+-------+-----+-------------+---------------+
| index| col1| col2| col3| grouped_counts|
+-----------+-------+-----+-------------+---------------+
|42949672960|a,b,c,d|a,c,d|a,b,c,d,a,c,d|a:4,b:2,c:4,d:4|
|94489280512| e,f,g|f,g,h| e,f,g,f,g,h|h:2,g:4,f:4,e:2|
+-----------+-------+-----+-------------+---------------+
请注意,我们在 udf 部分创建的 json 通常比grouped_counts
列中使用原生 pyspark 函数的简单字符串更方便使用。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.