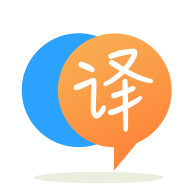
[英]OpenScad - How to find Robotic Arm Nodes Coordinates after Rotations - 5 axis
[英]How can I use multiprocessing in python to monitor the coordinates of a robotic arm?
我使用下面的代码在执行Move_Relative()
的同时打印我的x
和y
坐标。 似乎没有执行相对移动 function 因为在执行结束时我的 output 是( x = 0
和y = 0
)。 我究竟做错了什么?
import multiprocessing
from multiprocessing import Pool
import sys
x = 0
y = 0
def monitor_coordinates():
global x
global y
print("x: " + str(x) + " y: " + str(y))
def Move_Relative():
global x
global y
while x < 100000:
x = x + 1
y = y + 0.5
if __name__=='__main__':
q = multiprocessing.Process(target = Move_Relative)
q.start()
p = multiprocessing.Process(target = monitor_coordinates)
p.start()
q.join()
p.join()
全局变量不在进程之间共享。 一种不错的选择是使用multiprocessing.Value
import multiprocessing
from multiprocessing import Pool, Value
import sys
x = Value('d', 0)
y = Value('d', 0)
def monitor_coordinates(x, y):
print("x: " + str(x.value) + " y: " + str(y.value))
def Move_Relative(x, y):
while x.value < 100000:
x.value = x.value + 1
y.value = y.value + 0.5
if __name__ == '__main__':
q = multiprocessing.Process(target=Move_Relative, args=(x, y))
q.start()
p = multiprocessing.Process(target=monitor_coordinates, args=(x, y))
p.start()
q.join()
p.join()
output:
x: 88826.0 y: 44413.0
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.