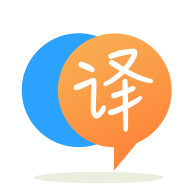
[英]How to convert only integars of an array of strings to an array of floats in numpy?
[英]How to convert an array of strings to an array of floats in numpy?
如何转换
["1.1", "2.2", "3.2"]
到
[1.1, 2.2, 3.2]
在 NumPy?
好吧,如果您正在以列表形式读取数据,只需执行np.array(map(float, list_of_strings))
(或等效地使用列表np.array(map(float, list_of_strings))
)。 (在Python 3中,如果使用map
,则需要在map
返回值上调用list
,因为map
现在返回一个迭代器。)
但是,如果它已经是一个numpy字符串数组,那么有一种更好的方法。 使用astype()
。
import numpy as np
x = np.array(['1.1', '2.2', '3.3'])
y = x.astype(np.float)
你也可以使用它
import numpy as np
x=np.array(['1.1', '2.2', '3.3'])
x=np.asfarray(x,float)
如果您有(或创建)单个字符串,则可以使用np.fromstring :
import numpy as np
x = ["1.1", "2.2", "3.2"]
x = ','.join(x)
x = np.fromstring( x, dtype=np.float, sep=',' )
注意, x = ','.join(x)
将x数组转换为字符串'1.1, 2.2, 3.2'
。 如果从txt文件中读取一行,则每行都将是一个字符串。
另一种选择可能是numpy.asarray :
import numpy as np
a = ["1.1", "2.2", "3.2"]
b = np.asarray(a, dtype=np.float64, order='C')
对于Python 2 *:
print a, type(a), type(a[0])
print b, type(b), type(b[0])
导致:
['1.1', '2.2', '3.2'] <type 'list'> <type 'str'>
[1.1 2.2 3.2] <type 'numpy.ndarray'> <type 'numpy.float64'>
您可以将np.array()
与 dtype dtype = float
一起使用:
import numpy as np
x = ["1.1", "2.2", "3.2"]
y = np.array(x,dtype=float)
Output:
array([1.1, 2.2, 3.2])
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.