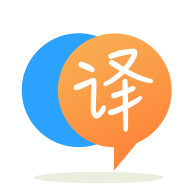
[英]fastest way to reshape 2D numpy array (gray image) into a 3D stacked array
[英]Numpy fastest 3D to 2D projection
我有一個二進制數據的3D數組。 我想把它投射到3個2D圖像 - 側面,正面,鳥眼。
我寫了代碼:
for x in range(data.shape[2]):
for y in range(data.shape[0]):
val = 0
for z in range(data.shape[1]):
if data[y][z][x] > 0:
val = 255
break
side[y][x] = val
但對於~700x300x300矩陣來說,這是非常緩慢的(75秒!)。
實現此任務的最快方法是什么?
編輯:
為了保存圖像,我使用了:
sideImage = Image.fromarray(side)
sideImage.convert('RGB').save("sideImage.png")
當我擁有3D數據時,我傾向於將其視為具有2D圖像的行,列和切片(或面板)的“立方體”。 每個切片或面板是2D圖像是尺寸的(rows, cols)
我通常會這樣想:
與(0,0,0)
在所述前片的左上角之中。 隨着numpy
索引是超級容易選擇3D陣列,你有興趣在無需編寫自己的循環只是部分:
>>> import numpy as np
>>> import matplotlib.pyplot as plt
>>> np.set_printoptions(precision=2)
# Generate a 3D 'cube' of data
>>> data3D = np.random.uniform(0,10, 2*3*5).reshape((2,3,5))
>>> data3D
array([[[ 7.44, 1.14, 2.5 , 3.3 , 6.05],
[ 1.53, 8.91, 1.63, 8.95, 2.46],
[ 3.57, 3.29, 6.43, 8.81, 6.43]],
[[ 4.67, 2.67, 5.29, 7.69, 7.59],
[ 0.26, 2.88, 7.58, 3.27, 4.55],
[ 5.84, 9.04, 7.16, 9.18, 5.68]]])
# Grab some "views" of the data
>>> front = data3D[:,:,0] # all rows and columns, first slice
>>> back = data3D[:,:,-1] # all rows and cols, last slice
>>> top = data3D[0,:,:] # first row, all cols, all slices
>>> bottom = data3D[-1,:,:] # last row, all cols, all slices
>>> r_side = data3D[:,-1,:] # all rows, last column, all slices
>>> l_side = data3D[:,0,:] # all rows, first column, all slices
看看前面的樣子:
>>> plt.imshow(front, interpolation='none')
>>> plt.show()
您可以按如下方式計算它:
>>> data = np.random.random_sample((200, 300, 100)) > 0.5
>>> data.any(axis=-1).shape # show the result has the shape we want
(200, 300)
>>> data.any(axis=-1)
array([[ True, True, True, ..., True, True, True],
[ True, True, True, ..., True, True, True],
[ True, True, True, ..., True, True, True],
...,
[ True, True, True, ..., True, True, True],
[ True, True, True, ..., True, True, True],
[ True, True, True, ..., True, True, True]], dtype=bool)
>>>
您可以根據需要縮放值
>>> data.any(axis=-1) * 255
array([[255, 255, 255, ..., 255, 255, 255],
[255, 255, 255, ..., 255, 255, 255],
[255, 255, 255, ..., 255, 255, 255],
...,
[255, 255, 255, ..., 255, 255, 255],
[255, 255, 255, ..., 255, 255, 255],
[255, 255, 255, ..., 255, 255, 255]])
>>>
一段時間后,我將下面的內容寫成3D陣列的可視化輔助工具。 也是一個很好的學習練習。
# Python 2.7.10
from __future__ import print_function
from numpy import *
def f_Print3dArray(a_Array):
v_Spacing = (len(str(amax(abs(a_Array)))) + 1) if amin(a_Array)\
< 0 else (len(str(amax(a_Array))) + 1)
for i in a_Array[:,:,::-1].transpose(0,2,1):
for index, j in enumerate(i):
print(" " * (len(i) - 1 - index) + "/ ", end="")
for k in j:
print(str(k).ljust( v_Spacing + 1), end="")
print("/")
print()
a_Array = arange(27).reshape(3, 3, 3)
print(a_Array)
print()
f_Print3dArray(a_Array)
轉換這個:
[[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]]
[[ 9 10 11]
[12 13 14]
[15 16 17]]
[[18 19 20]
[21 22 23]
[24 25 26]]]
對此:
/ 2 5 8 /
/ 1 4 7 /
/ 0 3 6 /
/ 11 14 17 /
/ 10 13 16 /
/ 9 12 15 /
/ 20 23 26 /
/ 19 22 25 /
/ 18 21 24 /
希望它可以幫助某人。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.