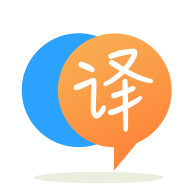
[英]How can I find the co-ordinates where the user wearing a VR Headset is looking at in a 360 video playing insider Unity Skybox?
[英]How can I find the centre co-ordinates of an array of connected lines?
對於每個段,計算(並存儲)段的長度。 將所有長度相加,然后將總數除以2。
按路徑順序遍歷所有段,從此減半的總和中減去每個段的長度,直到當前段的長度大於其余的總和。
然后沿着該線段計算該長度處的點。
這是一個抓住中間點的快速代碼示例:
Vector2 GetMidPoint(Vector2[] path)
{
var totalLength = 0d;
for(var i = 0; i < path.Length - 1; i++)
totalLength += GetDistanceBetween(path[i], path[i + 1]);
var halfLength = totalLength / 2;
var currLength = 0d;
for(var i = 0; i < path.Length - 1; i++)
{
var currentNode = path[i];
var nextNode = path[i+1];
var nextStepLength = GetDistanceBetween(currentNode, nextNode);
if (halfLength < currLength + nextStepLength)
{
var distanceLeft = halfLength - currLength;
var ratio = distanceLeft / nextStepLength;
return new Vector2(currentNode.x + (nextNode.x - currentNode.x) * ratio, currentNode.y + (nextNode.y - currentNode.y) * ratio);
}
else
currLength += nextStepLength;
}
throw new Exception("Couldn't get the mid point");
}
public double GetDistanceBetween(Vector2 a, Vector2 b)
{
var x = Math.Abs(a.x - b.x);
var y = Math.Abs(a.y - b.y);
return (Math.Sqrt(Math.Pow(x,2) + Math.Pow(y, 2)));
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.