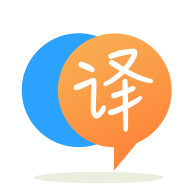
[英]How to product element-wise a 2-d numpy array into 3-d over the second dimension of the latter?
[英]Element-wise product of two 2-D lists
我不能使用Numpy或任何其他庫函數,因為這是我必須要做的一個問題,我必須定義自己的方式。
我正在編寫一個函數,它將兩個列表(2維)作為參數。 該函數應計算兩個列表的元素乘積並將它們存儲在第三個列表中,並從函數返回該結果列表。 輸入列表的示例是:
[[2,3,5,6,7],[5,2,9,3,7]]
[[5,2,9,3,7],[1,3,5,2,2]]
該函數打印以下列表:
[[10, 6, 45, 18, 49], [5, 6, 45, 6, 14]]
那是2*5=10
3*2=6
5*9=45
......依此類推。
這是我的下面的代碼,但它只適用於里面有2個列表(元素)的列表,就像上面的例子一樣,並且完全可以正常工作,但我想要的是編輯我的代碼,這樣無論有多少個列表(元素)存在於二維列表中,它應該在一個新的二維列表中打印出它的元素產品,例如它也應該適用於
[[5,2,9,3,7],[1,3,5,2,2],[1,3,5,2,2]]
要么
[[5,2,9,3,7],[1,3,5,2,2],[1,3,5,2,2],[5,2,9,3,7]]
或整個列表中的任意數量的列表。
def ElementwiseProduct(l,l2):
i=0
newlist=[] #create empty list to put prouct of elements in later
newlist2=[]
newlist3=[] #empty list to put both new lists which will have proudcts in them
while i==0:
a=0
while a<len(l[i]):
prod=l[i][a]*l2[i][a] #corresponding product of lists elements
newlist.append(prod) #adding the products to new list
a+=1
i+=1
while i==1:
a=0
while a<len(l[i]):
prod=l[i][a]*l2[i][a] #corresponding product of lists elements
newlist2.append(prod) #adding the products to new list
a+=1
i+=1
newlist3.append(newlist)
newlist3.append(newlist2)
print newlist3
#2 dimensional list example
list1=[[2,3,5,6,7],[5,2,9,3,7]]
list2=[[5,2,9,3,7],[1,3,5,2,2]]
ElementwiseProduct(list1,list2)
您可以zip
這兩個列表列表中的理解 ,則進一步zip
所產生的子表 ,然后最后乘以項目:
list2 = [[5,2,9,3,7],[1,3,5,2,2]]
list1 = [[2,3,5,6,7],[5,2,9,3,7]]
result = [[a*b for a, b in zip(i, j)] for i, j in zip(list1, list2)]
print(result)
# [[10, 6, 45, 18, 49], [5, 6, 45, 6, 14]]
如果列表 / 子列表沒有相同數量的元素,則itertools.izip_longest
可用於生成填充值,例如較小列表的空子列表,或較短子列表的0:
from itertools import izip_longest
list1 = [[2,3,5,6]]
list2 = [[5,2,9,3,7],[1,3,5,2,2]]
result = [[a*b for a, b in izip_longest(i, j, fillvalue=0)]
for i, j in izip_longest(list1, list2, fillvalue=[])]
print(result)
# [[10, 6, 45, 18, 0], [0, 0, 0, 0, 0]]
您可以將內部fillvalue
從0更改為1,以按fillvalue
返回較長子列表中的元素,而不是同類0。
參考 :
這是一個可以處理任何類型的iterable的函數,嵌套到任何級別(任意數量的維度,而不僅僅是2):
def elementwiseProd(iterA, iterB):
def multiply(a, b):
try:
iter(a)
except TypeError:
# You have a number
return a * b
return elementwiseProd(a, b)
return [multiply(*pair) for pair in zip(iterA, iterB)]
此函數遞歸工作。 對於列表中的每個元素,它檢查元素是否可迭代。 如果是,則輸出元素是包含迭代的元素乘法的列表。 如果不是,則返回數字的乘積。
此解決方案適用於混合嵌套類型。 這里做出的一些假設是,所有嵌套級別都是相同的大小,並且一個迭代中的數字元素(相對於嵌套的可迭代)始終是另一個中的數字。
事實上,這個代碼片段可以擴展為將任何n-ary函數應用於任何n個迭代:
def elementwiseApply(op, *iters):
def apply(op, *items):
try:
iter(items[0])
except TypeError:
return op(*items)
return elementwiseApply(op, *items)
return [apply(op, *items) for items in zip(*iters)]
要進行乘法運算,您可以使用operator.mul
:
from operator import mul
list1=[[2,3,5,6,7], [5,2,9,3,7]]
list2=[[5,2,9,3,7], [1,3,5,2,2]]
elementwiseApply(mul, list1, list2)
產生
[[10, 6, 45, 18, 49], [5, 6, 45, 6, 14]]
在Python中,通常最好直接循環遍歷列表中的項,而不是使用索引間接循環。 它使代碼更容易閱讀,也更有效,因為它避免了繁瑣的索引算法。
以下是使用傳統for
循環解決問題的方法。 我們使用內置的zip
函數同時迭代兩個(或更多)列表。
def elementwise_product(list1,list2):
result = []
for seq1, seq2 in zip(list1,list2):
prods = []
for u, v in zip(seq1, seq2):
prods.append(u * v)
result.append(prods)
return result
list1=[[2,3,5,6,7], [5,2,9,3,7]]
list2=[[5,2,9,3,7], [1,3,5,2,2]]
print(elementwise_product(list1,list2))
產量
[[10, 6, 45, 18, 49], [5, 6, 45, 6, 14]]
我們可以使用列表推導來使代碼更緊湊。 一開始看起來可能看起來更難,但你會習慣用練習列出理解。
def elementwise_product(list1,list2):
return [[u*v for u, v in zip(seq1, seq2)]
for seq1, seq2 in zip(list1,list2)]
你可以使用numpy數組。 它們是您最好的選擇,因為它們在C背景上運行,因此計算速度更快
首先,安裝numpy。 點擊您的終端(如果您在窗口中,則為CMD),鍵入
pip install numpy
或者,如果在Linux中, sudo pip install numpy
然后,繼續編寫代碼
import numpy as np
list1=np.array([[2,3,5,6,7],[5,2,9,3,7]]) #2 dimensional list example
list2=np.array([[5,2,9,3,7],[1,3,5,2,2]])
prod = np.multiply(list1,list2)
# or simply, as suggested by Mad Physicist,
prod = list1*list2
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.