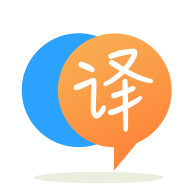
[英]TensorFlow Cannot feed value of shape (100, 784) for Tensor 'Placeholder:0'
[英]MNIST data - Cannot feed value of shape (1000, 784) for Tensor 'Placeholder:0', which has shape '(5500, 784)'
我嘗試使用1000個示例來測試數據,而獲得5500個訓練數據示例。 盡管我從用於訓練部分的(5500,784)更改了測試部分的(1000,784)的占位符參數,但仍然出現錯誤ValueError:無法為Tensor'占位符提供形狀的值(1000,784): 0',形狀為((5500,784)',有人可以幫忙嗎?
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
import random
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
def TRAIN_SIZE(num):
print ('Total Training Images in Dataset = ' + str(mnist.train.images.shape))
print ('--------------------------------------------------')
x_train = mnist.train.images[:num,:]
print ('x_train Examples Loaded = ' + str(x_train.shape))
y_train = mnist.train.labels[:num,:]
print ('y_train Examples Loaded = ' + str(y_train.shape))
print('')
return x_train, y_train
def TEST_SIZE(num):
print ('Total Test Examples in Dataset = ' + str(mnist.test.images.shape))
print ('--------------------------------------------------')
x_test = mnist.test.images[:num,:]
print ('x_test Examples Loaded = ' + str(x_test.shape))
y_test = mnist.test.labels[:num,:]
print ('y_test Examples Loaded = ' + str(y_test.shape))
return x_test, y_test
def display_digit(num,x_train,y_train):
print(y_train[num])
label = y_train[num].argmax(axis=0)
image = x_train[num].reshape([28,28])
plt.title('Example: %d Label: %d' % (num, label))
plt.imshow(image, cmap=plt.get_cmap('gray_r'))
# plt.show()
X_train, Y_train = TRAIN_SIZE(5500)
X_test, Y_test = TEST_SIZE(1000)
learning_rate = 0.01
train_steps = 2500
# Display 30 random digits
for i in range(30):
selected = random.randint(0,5500-1)
display_digit(selected, X_train, Y_train)
# n features, k classes
n = 784
k = 10
batch_size = 5500
X = tf.placeholder(tf.float32, [batch_size, 784])
Y = tf.placeholder(tf.float32, [batch_size, 10])
W = tf.Variable(tf.random_normal(shape=[784,10],stddev=0.01),name="weights")
b = tf.Variable(tf.zeros([1,10]),name="bias")
logits = tf.matmul(X, W) + b
entropy = tf.nn.softmax_cross_entropy_with_logits(logits= logits, labels=Y)
loss = tf.reduce_mean(entropy) #computes the mean over examples in the batch
optimizer = tf.train.GradientDescentOptimizer(learning_rate=learning_rate).minimize(loss)
X_ = tf.placeholder(tf.float32, [1000, 784])
preds = tf.nn.softmax(tf.matmul(X_, W) + b)
Y_ = tf.placeholder(tf.float32, [1000, 10])
correct_prediction = tf.equal(tf.argmax(preds, 1), tf.argmax(Y_, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
# Train model
for i in range(train_steps + 1):
sess.run([optimizer, loss], feed_dict={X: X_train, Y: Y_train})
if i % 100 == 0:
print('Training Step: ', i)
print('Accuracy:', sess.run(accuracy, feed_dict={X: X_test, Y: Y_test}))
# Obtain weights
W_values = sess.run(W)
# Display the weights
for i in range(10):
plt.subplot(2, 5, i+1)
weight = W_values[:,i]
plt.title(i)
plt.imshow(weight.reshape([28,28]), cmap=plt.get_cmap('seismic'))
frame1 = plt.gca()
frame1.axes.get_xaxis().set_visible(False)
frame1.axes.get_yaxis().set_visible(False)
plt.show()
我發現了你的錯誤。 您已將測試占位符聲明為X_和Y_,並且要提供X,Y,它們是應該提供測試占位符的地方的火車占位符。 您在2個地方提供X,Y火車占位符(火車,同時測試這兩個地方)。在代碼中更改以下行即可正常工作。
sess.run(accuracy,feed_dict={X_:X_test,Y_:Y_test})
我運行了您的代碼,並得到以下答案。 該程序仍在運行。
Extracting MNIST_data/train-images-idx3-ubyte.gz
Extracting MNIST_data/train-labels-idx1-ubyte.gz
Extracting MNIST_data/t10k-images-idx3-ubyte.gz
Extracting MNIST_data/t10k-labels-idx1-ubyte.gz
Total Training Images in Dataset = (55000, 784)
--------------------------------------------------
x_train Examples Loaded = (5500, 784)
y_train Examples Loaded = (5500, 10)
Total Test Examples in Dataset = (10000, 784)
--------------------------------------------------
x_test Examples Loaded = (1000, 784)
y_test Examples Loaded = (1000, 10)
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 1. 0. 0. 0. 0. 0. 0.]
[0. 1. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 1. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 1. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 1. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 0. 1.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 0. 1.]
[0. 0. 0. 0. 0. 0. 1. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 1. 0. 0.]
[0. 0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 1. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 1. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 1. 0. 0.]
[0. 0. 0. 0. 0. 0. 1. 0. 0. 0.]
[0. 0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 1. 0. 0. 0. 0. 0. 0.]
[0. 0. 1. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 1. 0. 0. 0. 0. 0.]
[1. 0. 0. 0. 0. 0. 0. 0. 0. 0.]
('Training Step: ', 0)
('Accuracy:', 0.098)
('Training Step: ', 100)
('Accuracy:', 0.716)
('Training Step: ', 200)
('Accuracy:', 0.763)
('Training Step: ', 300)
('Accuracy:', 0.778)
('Training Step: ', 400)
('Accuracy:', 0.79)
('Training Step: ', 500)
('Accuracy:', 0.802)
('Training Step: ', 600)
('Accuracy:', 0.81)
('Training Step: ', 700)
('Accuracy:', 0.821)
('Training Step: ', 800)
('Accuracy:', 0.829)
('Training Step: ', 900)
('Accuracy:', 0.833)
('Training Step: ', 1000)
('Accuracy:', 0.836)
('Training Step: ', 1100)
('Accuracy:', 0.84)
('Training Step: ', 1200)
('Accuracy:', 0.846)
('Training Step: ', 1300)
('Accuracy:', 0.85)
('Training Step: ', 1400)
('Accuracy:', 0.853)
('Training Step: ', 1500)
('Accuracy:', 0.853)
占位符的形狀應為(None,784)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.