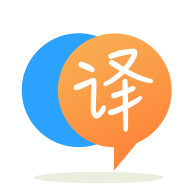
[英]Python: Extract the indices of repeated rows corresponding to the non-zero unique rows in a matrix
[英]Python: Store indices of non-zero unique rows after comparing each rows with every other row in a matrix
對於這個矩陣K =
[[-1. 1. 0.]
[ 0. 0. 0.]
[ 0. -1. 1.]
[ 0. 0. 0.]
[ 0. -1. 1.]
[ 0. 0. 0.]]
任務是在數組中存儲非零唯一行的索引(這里的答案是{0,2}),所以
K([0,2],:)
可用於線性代數運算。 我的嘗試是:
myList = []
for i in range(len(K)): #generate pairs
for j in range(i+1,len(K)): #travel down each other rows
if np.array_equal(K[i],K[j]) and np.any(K[i] != 0, axis=1) and np.any(K[j] != 0, axis=1):
myList.append(K[i])
print ('indices of similar-non-zeros rows are\n',(i, j)),
elif not np.array_equal(K[i],K[j]) and np.any(K[i] != 0,axis=1) and np.any(K[j] != 0, axis=1):
myList.append(K[i])
print ('indices of non-similar-non-zeros rows are\n',(i, j)),
else:
continue
new_K = np.asmatrix(np.asarray(myList))
new_new_K = np.unique(new_K,axis=0)
print('Now K is \n',new_new_K)
答案是:
new_new_K = [[-1. 1. 0.]
[ 0. -1. 1.]]
問題1:如何以pythonic方式進行。 以上是具有矩陣存儲限制的替代解決方案,但更優選地將索引存儲在陣列中。
您可以使用帶enumerate
的簡單for
循環。
import numpy as np
A = np.array([[-1, 1, 0],
[ 0, 0, 0],
[ 0, -1, 1],
[ 0, 0, 0],
[ 0, -1, 1],
[ 0, 0, 0]])
seen = {(0, 0, 0)}
res = []
for idx, row in enumerate(map(tuple, A)):
if row not in seen:
res.append(idx)
seen.add(row)
結果
print(A[res])
[[-1 1 0]
[ 0 -1 1]]
例#2
import numpy as np
A=np.array([[0, 1, 0, 0, 0, 1],
[0, 0, 0, 1, 0, 1],
[0, 1, 0, 0, 0, 1],
[1, 0, 1, 0, 1, 1],
[1, 1, 1, 0, 0, 0],
[0, 1, 0, 1, 0, 1],
[0, 0, 0, 0, 0, 0]])
seen={(0, )*6}
res = []
for idx, row in enumerate(map(tuple, A)):
if row not in seen:
res.append(idx)
seen.add(row)
print(A[res])
# [[0 1 0 0 0 1]
# [0 0 0 1 0 1]
# [1 0 1 0 1 1]
# [1 1 1 0 0 0]
# [0 1 0 1 0 1]]
你可以使用np.unique
及其axis
參數來獲取起始的唯一行索引,然后過濾掉唯一一行的索引,其對應的行是全零,如下所示 -
def unq_row_indices_wozeros(a):
# Get unique rows and their first occuring indices
unq, idx = np.unique(a, axis=0, return_index=1)
# Filter out the index, the corresponding row of which is ALL 0s
return idx[(unq!=0).any(1)]
樣品運行 -
In [53]: # Setup input array with few all zero rows and duplicates
...: np.random.seed(0)
...: a = np.random.randint(0,9,(10,3))
...: a[[2,5,7]] = 0
...: a[4] = a[1]
...: a[8] = a[3]
In [54]: a
Out[54]:
array([[5, 0, 3],
[3, 7, 3],
[0, 0, 0],
[7, 6, 8],
[3, 7, 3],
[0, 0, 0],
[1, 5, 8],
[0, 0, 0],
[7, 6, 8],
[2, 3, 8]])
In [55]: unq_row_indices_wozeros(a)
Out[55]: array([6, 9, 1, 0, 3])
# Sort those indices if needed
In [56]: np.sort(unq_row_indices_wozeros(a))
Out[56]: array([0, 1, 3, 6, 9])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.