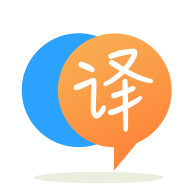
[英]Cannot cast array data from dtype('float64') to dtype('int32') according to 'safe'
[英]Cannot cast array data from dtype('float64') to dtype('int32') according to the rule 'safe'
我有一個像numpy數組
result = np.array([[[289, 354, 331],
[291, 206, 66],
[242, 70, 256]],
[[210, 389, 342],
[273, 454, 218],
[255, 87, 256]],
[[127, 342, 173],
[450, 395, 147],
[223, 228, 401]]])
我試圖掩蓋數組,如果一個元素大於255.即我假設它是0-1024范圍並將我的值除以4
result = np.putmask(result, result > 255, result/4)
注意:結果是以前的3D數組。我收到此錯誤
TypeError: Cannot cast array data from dtype('float64') to dtype('int32') according to the rule 'safe'
我究竟做錯了什么? 提前致謝
錯誤說明:
這說明了numpy數組的一個有趣屬性:numpy數組的所有元素必須是相同的類型
例如,如果您有以下數組:
>>> array1 = np.array([[23, 632, 634],[23.5, 67, 123.6]])
>>> array1
array([[ 23. , 632. , 634. ],
[ 23.5, 67. , 123.6]])
>>> type(array1[0][0])
<class 'numpy.float64'>
我們注意到,即使列表[ 23,632,634 ]中的所有元素都是int類型(特別是'numpy.int64' ), array1中的所有元素都被轉換為浮點數,因為元素123.6在第二行(注意數組中的小數點打印出來)。
類似地,如果我們在數組中的任何位置包含一個字符串,則數組的所有元素都將轉換為字符串:
>>> array2 = np.array([[23, 632, 'foo'],[23.5, 67, 123.6]])
>>> type(array2[0][0])
<class 'numpy.str_'>
結論:
原始結果數組包含'numpy.int64'類型的元素,但result/4
操作返回類型為'numpy.float64'的元素數組(因為82/4 = 20.5等)。 因此,當您嘗試並替換結果中的值時,它不是“安全”,因為您無意中嘗試將浮動放入一個int數組中。
問題是當你除以4時,你正在創建浮點值,它們不想進入int
數組。
如果你想使用putmask
,並避免嘗試轉換為float的問題,那么你可以使用floor division( //
)來將你的值更改為int
:
np.putmask(result, result>255, result//4)
>>> result
array([[[ 72, 88, 82],
[ 72, 206, 66],
[242, 70, 64]],
[[210, 97, 85],
[ 68, 113, 218],
[255, 87, 64]],
[[127, 85, 173],
[112, 98, 147],
[223, 228, 100]]])
將result
數組轉換為float dtype
,並使用原始putmask
:
result = result.astype(float)
np.putmask(result, result > 255, result/4)
>>> result
array([[[ 72.25, 88.5 , 82.75],
[ 72.75, 206. , 66. ],
[242. , 70. , 64. ]],
[[210. , 97.25, 85.5 ],
[ 68.25, 113.5 , 218. ],
[255. , 87. , 64. ]],
[[127. , 85.5 , 173. ],
[112.5 , 98.75, 147. ],
[223. , 228. , 100.25]]])
如果需要,您甚至可以轉換回int:
result = result.astype(int)
array([[[ 72, 88, 82],
[ 72, 206, 66],
[242, 70, 64]],
[[210, 97, 85],
[ 68, 113, 218],
[255, 87, 64]],
[[127, 85, 173],
[112, 98, 147],
[223, 228, 100]]])
完全putmask
,你可以得到你想要的結果:
result[result > 255] = result[result > 255] / 4
>>> result
array([[[ 72, 88, 82],
[ 72, 206, 66],
[242, 70, 64]],
[[210, 97, 85],
[ 68, 113, 218],
[255, 87, 64]],
[[127, 85, 173],
[112, 98, 147],
[223, 228, 100]]])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.