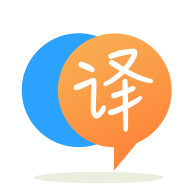
[英]How to make a control snap to a Grid.Row/Grid.Column in WPF at runtime?
[英]Make a placeable object snap to grid
我在我的游戲中有一個構建機制,我想知道我是如何做到這一點的,所以每當我放下對象時它都會對齊網格。 每當我按0時,它會將對象移動到我的鼠標上,每當我點擊它時,都會將對象放下。 我想讓它在放置時對齊網格,這樣物體就不會碰撞。
using UnityEngine; using UnityEngine.AI; public class GroundPlacementController : MonoBehaviour { [SerializeField] private GameObject placeableObjectPrefab; public NavMeshObstacle nav; [SerializeField] private KeyCode newObjectHotkey = KeyCode.A; private GameObject currentPlaceableObject; private float mouseWheelRotation; private void Update() { HandleNewObjectHotkey(); nav = GetComponent<NavMeshObstacle>(); if (currentPlaceableObject != null) { MoveCurrentObjectToMouse(); RotateFromMouseWheel(); ReleaseIfClicked(); } } private void HandleNewObjectHotkey() { if (Input.GetKeyDown(newObjectHotkey)) { if (currentPlaceableObject != null) { Destroy(currentPlaceableObject); } else { currentPlaceableObject = Instantiate(placeableObjectPrefab); } } } private void MoveCurrentObjectToMouse() { Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition); RaycastHit hitInfo; if (Physics.Raycast(ray, out hitInfo)) { currentPlaceableObject.transform.position = hitInfo.point; currentPlaceableObject.transform.rotation = Quaternion.FromToRotation(Vector3.up, hitInfo.normal); currentPlaceableObject.GetComponent<NavMeshObstacle>().enabled = false; } } private void RotateFromMouseWheel() { Debug.Log(Input.mouseScrollDelta); mouseWheelRotation += Input.mouseScrollDelta.y; currentPlaceableObject.transform.Rotate(Vector3.up, mouseWheelRotation * 90f); } private void ReleaseIfClicked() { if (Input.GetMouseButtonDown(0)) { currentPlaceableObject.GetComponent<NavMeshObstacle>().enabled = true; print("disabled"); currentPlaceableObject = null; print("removed prefab"); } } }
您可以通過簡單地舍入transform.position
每個軸來捕捉代碼中的網格:
var currentPosition = transform.position;
transform.position = new Vector3(Mathf.Round(currentPosition.x),
Mathf.Round(currentPosition.y),
Mathf.Round(currentPosition.z));
一個更加數學的方法,你可以輕松設置網格大小(除了Lews Therin的答案)將是:
position
mod
yourGridSize
(比如,你的網格大小是64,位置是144. 那么 :144 mod
64 = 16) mod
並從位置減去:144 - 16 = 128 yourGridSize
: yourGridSize
= 2 現在你知道你的位置是進入網格的2
個街區。 對三軸應用此操作:
var yourGridSize = 64;
var currentPosition = currentPlaceableObject.transform.position;
currentPlaceableObject.transform.position = new Vector3(((currentPosition.x - (currentPosition.x % yourGridSize)) / yourGridSize) * yourGridSize,
((currentPosition.y - (currentPosition.y % yourGridSize)) / yourGridSize) * yourGridSize,
((currentPosition.z - (currentPosition.z % yourGridSize)) / yourGridSize) * yourGridSize);
令人費解? 也許。 有效? 哎呀!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.