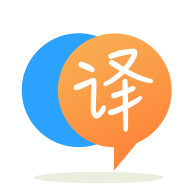
[英]Questions that in case of fluctuating the validation accuracy and loss curve for image binary classification, ask the way of analysis and solution
[英]Validation Loss and Validation Accuracy Curve Fluctuating with the Pretrained Model
我目前正在研究神經網絡,在嘗試學習 CNN 時遇到問題,我正在嘗試訓練包含有關音樂流派的頻譜圖的數據。 我的數據由 27000 個頻譜圖組成,分為 3 個 class(流派)。 我的數據以 9:1 的比例拆分用於訓練和驗證
誰能幫助我,為什么我的驗證損失/准確性的結果會波動? 我正在使用 Keras 的 MobileNetV2 並將其與 3 個密集層連接。 這是我的代碼片段:
train_datagen = ImageDataGenerator(
preprocessing_function=preprocess_input,
validation_split=0.1)
train_generator = train_datagen.flow_from_dataframe(
dataframe=traindf,
directory="...",
color_mode='rgb',
x_col="ID",
y_col="Class",
subset="training",
batch_size=32,
seed=42,
shuffle=True,
class_mode="categorical",
target_size=(64, 64))
valid_generator = train_datagen.flow_from_dataframe(
dataframe=traindf,
directory="...",
color_mode='rgb',
x_col="ID",
y_col="Class",
subset="validation",
batch_size=32,
seed=42,
shuffle=True,
class_mode="categorical",
target_size=(64, 64))
base_model = MobileNetV2(weights='imagenet', include_top=False)
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(1025, activation='relu')(x)
x = Dense(1025, activation='relu')(x)
x = Dense(512, activation='relu')(x)
preds = Dense(3, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=preds)
model.compile(optimizer='adam', loss='categorical_crossentropy',
metrics=['accuracy'])
step_size_train = train_generator.n//train_generator.batch_size
step_size_valid = valid_generator.n//valid_generator.batch_size
history = model.fit_generator(
generator=train_generator,
steps_per_epoch=step_size_train,
validation_data=valid_generator,
validation_steps=step_size_valid,
epochs=75)
這些是我的驗證損失和驗證准確度曲線波動太大的圖片
有沒有辦法減少波動或讓它變得更好? 我在這里有過度擬合或擬合不足的問題嗎? 我曾嘗試使用 Dropout() ,但它只會讓情況變得更糟。 我需要做什么來解決這個問題?
感謝之前,Aquilla Setiawan Kanadi。
首先,缺少驗證損失和驗證准確性的圖片。
要回答您的問題,以下可能是您的驗證丟失和驗證准確性波動的原因 -
(model Trainable Parameters 5115398 - base_model Trainable Parameters 2223872 = 2891526)
節目統計:
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import GlobalAveragePooling2D, Dense
from keras.utils.layer_utils import count_params
class color:
PURPLE = '\033[95m'
CYAN = '\033[96m'
DARKCYAN = '\033[36m'
BLUE = '\033[94m'
GREEN = '\033[92m'
YELLOW = '\033[93m'
RED = '\033[91m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
END = '\033[0m'
base_model = tf.keras.applications.MobileNetV2(weights='imagenet', include_top=False)
#base_model.summary()
trainable_count = count_params(base_model.trainable_weights)
non_trainable_count = count_params(base_model.non_trainable_weights)
print("\n",color.BOLD + ' base_model Statistics !' + color.END)
print("Trainable Parameters :", color.BOLD + str(trainable_count) + color.END)
print("Non Trainable Parameters :", non_trainable_count,"\n")
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(1025, activation='relu')(x)
x = Dense(1025, activation='relu')(x)
x = Dense(512, activation='relu')(x)
preds = Dense(3, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=preds)
#model.summary()
trainable_count = count_params(model.trainable_weights)
non_trainable_count = count_params(model.non_trainable_weights)
print(color.BOLD + ' model Statistics !' + color.END)
print("Trainable Parameters :", color.BOLD + str(trainable_count) + color.END)
print("Non Trainable Parameters :", non_trainable_count,"\n")
new_weights_added = count_params(model.trainable_weights) - count_params(base_model.trainable_weights)
print("Additional trainable weights added to the model excluding basel model trainable weights :", color.BOLD + str(new_weights_added) + color.END)
Output -
WARNING:tensorflow:`input_shape` is undefined or non-square, or `rows` is not in [96, 128, 160, 192, 224]. Weights for input shape (224, 224) will be loaded as the default.
base_model Statistics !
Trainable Parameters : 2223872
Non Trainable Parameters : 34112
model Statistics !
Trainable Parameters : 5115398
Non Trainable Parameters : 34112
Additional trainable weights added to the model excluding basel model trainable weights : 2891526
您的問題的解決方案是 -
以與 base_model 可訓練參數相比新的可訓練參數最小的方式自定義附加層。 可以添加最大池化層和更少的密集層。
通過base_model.trainable = False
凍結基礎 model 並僅訓練您在 MobileNetV2 層之上添加的新層。
或者
解凍基礎模型的頂層(MobileNetV2 層)並將底層設置為不可訓練。 您可以按如下方式進行,我們將 model 凍結到第 100 層,其余層將是可訓練的 -
# Let's take a look to see how many layers are in the base model
print("Number of layers in the base model: ", len(base_model.layers))
# Fine-tune from this layer onwards
fine_tune_at = 100
# Freeze all the layers before the `fine_tune_at` layer
for layer in base_model.layers[:fine_tune_at]:
layer.trainable = False
Output -
Number of layers in the base model: 155
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.