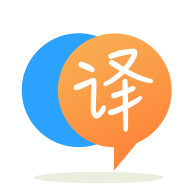
[英]How to create column with values from column of duplicated rows separated by commas in DataFrame in Python Pandas?
[英]How to extract the rows of a dataframe where a combination of specified column values are duplicated?
假設我有以下數據框:
import pandas as pd
data = {'Year':[2018, 2018, 2018, 2018, 2018, 2018, 2018, 2018],
'Month':[1,1,1,2,2,3,3,3],
'ID':['A', 'A', 'B', 'A', 'B', 'A', 'B', 'B'],
'Fruit':['Apple', 'Banana', 'Apple', 'Pear', 'Mango', 'Banana', 'Apple', 'Mango']}
df = pd.DataFrame(data, columns=['Year', 'Month', 'ID', 'Fruit'])
df = df.astype(str)
df
我想提取重復的“年”、“月”和“ID”的組合。 因此,使用上述數據框,預期結果是此數據框:
我這樣做的方法是先做一個groupby
來計算Year
, Month
和ID
的組合出現的次數:
df2 = df.groupby(['Year', 'Month'])['ID'].value_counts().to_frame(name = 'Count').reset_index()
df2 = df2[df2.Count>1]
df2
然后,我的想法是遍歷 groupby 數據框中的Year
、 Month
和ID
組合,並將與原始數據框中的組合匹配的那些行提取到一個新的數據框中:
df_new = pd.DataFrame(columns=df.columns, index=range(sum(df2.Count)))
count = 0
for i in df2.index:
temp = df[(df.ID==df2.ID[i]) & (df.Year==df2.Year[i]) & (df.Month==df2.Month[i])]
temp.reset_index(drop=True, inplace=True)
for j in range(len(temp)):
df_new.iloc[count] = temp.iloc[j]
count+=1
df_new
但這會產生以下錯誤:
---------------------------------------------------------------------------
IndexError Traceback (most recent call last)
<ipython-input-38-7f2d95d71270> in <module>()
6 temp.reset_index(drop=True, inplace=True)
7 for j in range(len(temp)):
----> 8 df_new.iloc[count] = temp.iloc[j]
9 count+=1
10 df_new
c:\users\h473\appdata\local\programs\python\python35\lib\site-packages\pandas\core\indexing.py in __setitem__(self, key, value)
187 else:
188 key = com.apply_if_callable(key, self.obj)
--> 189 indexer = self._get_setitem_indexer(key)
190 self._setitem_with_indexer(indexer, value)
191
c:\users\h473\appdata\local\programs\python\python35\lib\site-packages\pandas\core\indexing.py in _get_setitem_indexer(self, key)
173
174 try:
--> 175 return self._convert_to_indexer(key, is_setter=True)
176 except TypeError as e:
177
c:\users\h473\appdata\local\programs\python\python35\lib\site-packages\pandas\core\indexing.py in _convert_to_indexer(self, obj, axis, is_setter)
2245
2246 try:
-> 2247 self._validate_key(obj, axis)
2248 return obj
2249 except ValueError:
c:\users\h473\appdata\local\programs\python\python35\lib\site-packages\pandas\core\indexing.py in _validate_key(self, key, axis)
2068 return
2069 elif is_integer(key):
-> 2070 self._validate_integer(key, axis)
2071 elif isinstance(key, tuple):
2072 # a tuple should already have been caught by this point
c:\users\h473\appdata\local\programs\python\python35\lib\site-packages\pandas\core\indexing.py in _validate_integer(self, key, axis)
2137 len_axis = len(self.obj._get_axis(axis))
2138 if key >= len_axis or key < -len_axis:
-> 2139 raise IndexError("single positional indexer is out-of-bounds")
2140
2141 def _getitem_tuple(self, tup):
IndexError: single positional indexer is out-of-bounds
有什么錯誤? 我無法弄清楚。
當我將for
循環的內容更改為以下內容時,錯誤消失了,這會產生所需的結果:
for j in range(len(temp)):
df_new.ID[count] = temp.ID[j]
df_new.Year[count] = temp.Year[j]
df_new.Month[count] = temp.Month[j]
df_new.Fruit[count] = temp.Fruit[j]
count+=1
但這是一種繁瑣的解決方法,涉及為原始數據幀中的n
列中的每一列編寫n
行。
使用GroupBy.transform
與任何列和計算由GroupBy.size
的系列與原來一樣大小相同,通過這樣可以過濾boolean indexing
:
df1 = df[df.groupby(['Year','Month','ID'])['ID'].transform('size') > 1]
或者,如果小DataFrame
或性能不重要,請使用DataFrameGroupBy.filter
:
df1 = df.groupby(['Year','Month','ID']).filter(lambda x: len(x) > 1)
print (df1)
Year Month ID Fruit
0 2018 1 A Apple
1 2018 1 A Banana
6 2018 3 B Apple
7 2018 3 B Mango
您可以使用參數keep=False
duplicated
的方法來選擇所有重復項:
df[df.duplicated(subset=['Year', 'Month', 'ID'], keep=False)]
輸出:
Year Month ID Fruit
0 2018 1 A Apple
1 2018 1 A Banana
6 2018 3 B Apple
7 2018 3 B Mango
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.