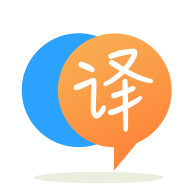
[英]Find the largest drop between two numbers in an array. What is the time complexity of this code?
[英]What will be the run time complexity for recursive algorithm for finding the largest number in an array. Compare it vs iterative loop code
我已經編寫了迭代循環代碼中最大數字的代碼。 而且我發現代碼的運行時間復雜度是 O(n)。 最大數量的遞歸代碼的運行時間復雜度是多少,它與迭代循環有何不同。 哪個會更好。 我的迭代循環代碼是
package com.bharat;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the numbers you want to add in the array: ");
int number = scanner.nextInt();
int[] myIntgersArray = getIntegers(number);
System.out.println(Arrays.toString(myIntgersArray));
System.out.println(findBiggestNumber(myIntgersArray));
}
public static int[] getIntegers(int number){
Scanner scanner = new Scanner(System.in);
int[] values= new int[number];
for (int i =0;i<values.length;i++){
values[i]=scanner.nextInt();
}
return values;
}
public static int findBiggestNumber(int[] array){
int i=0;
int biggestNumber = array[i];
for ( i = 0;i<array.length;i++){
if (array[i]>biggestNumber){
biggestNumber=array[i];
}
}
return biggestNumber;
}
}
在評論中發布的遞歸代碼 -
public static int findBiggestNumber(int[] array, int number) {
int highestNumber = array[0];
if (number==1) {
return highestNumber;
}
else {
if (highestNumber < array[number]) {
array[number] = highestNumber;
return highestNumber;
}
}
return findBiggestNumber(array, number-1);
}
正確的遞歸代碼(假設數組至少有 1 個元素) -
public static int findBiggestNumber(int[] array, int number)
{
if(number == 1) //only one element is there in array
{
return array[number - 1];
}
return Math.max( array[number - 1] , findBiggestNumber(array,number-1) );
}
遞歸代碼在 O(n) 時間內運行,但由於遞歸 function 調用堆棧,它具有 O(n) 的額外空間開銷。
Why the recursive code has O(n) time?
它解決n-1
較小的問題。 從第n-1
個問題的結果中,第n
個問題在 O(1) 時間內得到解決。
How the recursive code works?
如果您有一個具有n
大小數組的數組,則該數組的最大元素是
1. 最后一個元素array[n-1]
,或
2. n-1
大小數組的最大元素。
要計算n-1
大小數組的結果,請類似地重復。
基本情況是,當數組中只有一個元素時,這是最大值。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.