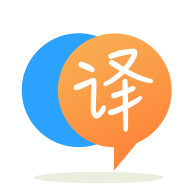
[英]How can I change the value of 'Draw Halo' inside Point Light - Unity3D
[英]How can I control a point light's color in Unity?
我已經設法將一種材料應用於網格,隨着時間的推移不斷地改變顏色(從一組顏色中挑選出來),我想對點光源做同樣的事情。 我怎樣才能做到這一點?
這是控制材質顏色的腳本,我想對其進行調整,因此它也可以控制燈光的顏色。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ChangeColors : MonoBehaviour
{
public Color[] colors;
public int currentIndex = 0;
private int nextIndex;
public float changeColourTime = 2.0f;
private float lastChange = 0.0f;
private float timer = 0.0f;
void Start()
{
if (colors == null || colors.Length < 2)
Debug.Log("Need to setup colors array in inspector");
nextIndex = (currentIndex + 1) % colors.Length;
}
void Update()
{
timer += Time.deltaTime;
if (timer > changeColourTime)
{
currentIndex = (currentIndex + 1) % colors.Length;
nextIndex = (currentIndex + 1) % colors.Length;
timer = 0.0f;
}
GetComponent<Renderer>().material.color = Color.Lerp(colors[currentIndex], colors[nextIndex], timer / changeColourTime);
}
}
您只需要在腳本中獲取對點光源的引用並更改其顏色,就像您為渲染器的材質所做的那樣。
您的腳本可以改進很多,但只需進行少量更改,如下所示:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ChangeColors : MonoBehaviour
{
public Color[] colors;
public Light pLight;
public int currentIndex = 0;
private int nextIndex;
public float changeColourTime = 2.0f;
private float lastChange = 0.0f;
private float timer = 0.0f;
void Start()
{
if (colors == null || colors.Length < 2)
Debug.Log("Need to setup colors array in inspector");
nextIndex = (currentIndex + 1) % colors.Length;
}
void Update()
{
timer += Time.deltaTime;
if (timer > changeColourTime)
{
currentIndex = (currentIndex + 1) % colors.Length;
nextIndex = (currentIndex + 1) % colors.Length;
timer = 0.0f;
}
Color c = Color.Lerp(colors[currentIndex], colors[nextIndex], timer / changeColourTime);
GetComponent<Renderer>().material.color = c; // MIND! you should store the Renderer reference in a variable rather than getting it once per frame!!!
pLight.color = c;
}
}
這是舊的,但您只需要訪問燈光組件
GetComponent Light.color
而不是Renderer.material.color
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.