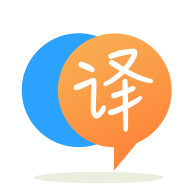
[英]Import multiple csv files into pandas and concatenate into one DataFrame where 1st column same in all csv and no headers of data just file name
[英]How do I concatenate files that have multiple sheets with same column headers but randomly ordered in Python/Pandas?
我有 3 個 xls 文件,每個文件有 3 張。 所有工作表都有相同的列標題,但如下所示的順序不同
1.xls
Name Address Date City State Zip
2.xls
Address Date City Zip Name State
3.xls
City Zip Name Address Date State
我希望我的最終 xls 文件連接所有 3 個文件和工作表
Output.xls
Name Address Date City State Zip RowNumber SheetName
rownumber 應該是來自每個文件的特定行號,並且在連接之前數據來自工作表。Sheetname 應該是它來自 xls 文件中的工作表。
我的嘗試-
import os
import pandas as pd
#set src directory
os.chdir('C:/Users/hhh/Desktop/python/Concat')
def read_sheets(filename):
result = []
sheets = pd.read_excel(filename, sheet_name=None)
for name, sheet in sheets.items():
sheet['Sheetname'] = name
sheet['Row'] = sheet.index
result.append(sheet)
return pd.concat(result, ignore_index=True)
files = [file for file in os.listdir(folder_path) if file.endswith(".xls")]
dfoo = read_sheets(files)
但什么也沒發生,我只是收到一個斷言錯誤,說 assert content_or_path is not None。 這是因為列順序不匹配嗎? 有解決方法嗎? 所有文件和工作表中的列數都相同。 在每個文件表中都有相同的順序。 但是,如果您將 1.xls 工作表與 2.xls 進行比較,則列順序會有所不同,正如您在上面的 reprex 中看到的那樣
我相信您的問題是要求獲取 9 個不同的工作表(3 個不同的 .xls 文件中的每個 3 個)並將它們合並到新電子表格 Output.xls 中的一個工作表中。
一些評論開始:
FutureWarning:
As the xlwt package is no longer maintained, the xlwt engine will be removed in a future version of pandas.
This is the only engine in pandas that supports writing in the xls format.
Install openpyxl and write to an xlsx file instead.
You can set the option io.excel.xls.writer to 'xlwt' to silence this warning.
While this option is deprecated and will also raise a warning, it can be globally set and the warning suppressed.
writer = pd.ExcelWriter('Output.xls')
這是對您的代碼的修改,它可以滿足您的要求(對 os.chdir() 使用不同的參數以匹配我的測試環境):
import os
import pandas as pd
#set src directory
#os.chdir('C:/Users/hhh/Desktop/python/Concat')
os.chdir('./Concat')
def read_sheets(files):
result = []
for filename in files:
sheets = pd.read_excel(filename, sheet_name=None)
for name, sheet in sheets.items():
sheet['Sheetname'] = name
sheet['Row'] = sheet.index
result.append(sheet)
return pd.concat(result, ignore_index=True)
folder_path = '.'
files = [file for file in os.listdir(folder_path) if file.endswith(".xls")]
dfCombined = read_sheets(files)
writer = pd.ExcelWriter('Output.xls')
dfCombined.to_excel(writer, index=None, sheet_name='Combined')
writer.save()
writer.close()
樣本 output 如下所示:
Name Address Date City State Zip Sheetname Row
Alice 1 Main St 11 Nome Alaska 11111 Sheet1 0
Bob 1 Main St 12 Providence Rhode Island 22222 Sheet1 1
Candace 1 Main St 13 Denver Colorado 33333 Sheet1 2
Dirk 1 Main St 14 Wilmington Delaware 44444 Sheet1 3
Edward 1 Marvin Gardens 11 Nome Alaska 11111 Sheet2 0
Fran 1 Marvin Gardens 12 Providence Rhode Island 22222 Sheet2 1
George 1 Marvin Gardens 13 Denver Colorado 33333 Sheet2 2
Hannah 1 Marvin Gardens 14 Wilmington Delaware 44444 Sheet2 3
Irvin 1 St Marks Place 11 Nome Alaska 11111 Sheet3 0
Jasmine 1 St Marks Place 12 Providence Rhode Island 22222 Sheet3 1
Kirk 1 St Marks Place 13 Denver Colorado 33333 Sheet3 2
Lana 1 St Marks Place 14 Wilmington Delaware 44444 Sheet3 3
Alice 2 Main St 11 Nome Alaska 11111 Sheet1 0
Bob 2 Main St 12 Providence Rhode Island 22222 Sheet1 1
Candace 2 Main St 13 Denver Colorado 33333 Sheet1 2
Dirk 2 Main St 14 Wilmington Delaware 44444 Sheet1 3
Edward 2 Marvin Gardens 11 Nome Alaska 11111 Sheet2 0
Fran 2 Marvin Gardens 12 Providence Rhode Island 22222 Sheet2 1
George 2 Marvin Gardens 13 Denver Colorado 33333 Sheet2 2
Hannah 2 Marvin Gardens 14 Wilmington Delaware 44444 Sheet2 3
Irvin 2 St Marks Place 11 Nome Alaska 11111 Sheet3 0
Jasmine 2 St Marks Place 12 Providence Rhode Island 22222 Sheet3 1
Kirk 2 St Marks Place 13 Denver Colorado 33333 Sheet3 2
Lana 2 St Marks Place 14 Wilmington Delaware 44444 Sheet3 3
Alice 3 Main St 11 Nome Alaska 11111 Sheet1 0
Bob 3 Main St 12 Providence Rhode Island 22222 Sheet1 1
Candace 3 Main St 13 Denver Colorado 33333 Sheet1 2
Dirk 3 Main St 14 Wilmington Delaware 44444 Sheet1 3
Edward 3 Marvin Gardens 11 Nome Alaska 11111 Sheet2 0
Fran 3 Marvin Gardens 12 Providence Rhode Island 22222 Sheet2 1
George 3 Marvin Gardens 13 Denver Colorado 33333 Sheet2 2
Hannah 3 Marvin Gardens 14 Wilmington Delaware 44444 Sheet2 3
Irvin 3 St Marks Place 11 Nome Alaska 11111 Sheet3 0
Jasmine 3 St Marks Place 12 Providence Rhode Island 22222 Sheet3 1
Kirk 3 St Marks Place 13 Denver Colorado 33333 Sheet3 2
Lana 3 St Marks Place 14 Wilmington Delaware 44444 Sheet3 3
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.