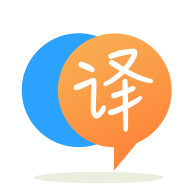
[英]I have a list in one class and I'm having trouble accessing it from another class C#
[英]I'm having some issues referencing a class to another class and the error I'm getting is I don't have an argument for a formal perimeter
我有一個 Player 類,我試圖將該類引用到我的 Main 類。 但是我在那里放了一些代碼,當我嘗試將它引用到我的主類時,這給了我一個錯誤。 沒有與所需的正式周長相對應的給定參數,'Player.PLayer(string,int)'的'statName'有人可以幫助我嗎? 我很確定這與將所有代碼混合在一起有關,但我只是不知道並且想要一點幫助。 這是我的課。
這是我的程序課
class Program
{
PLayer Playerstat = new PLayer(); // I'm trying to reference the class Player here but it's not letting me.
static void Main()
{
Console.WriteLine("please pick a mode");
PLayGame();
PickMode();
Console.ReadKey();
}
public static void PLayGame()
{
List<string> Options = new List<string> { "Easy mode: ", "med mode: ", "Hard mode", "super hard mode: " };
foreach(string ModePick in Options)
{
Thread.Sleep(570);
Console.WriteLine(ModePick);
}
}
private static void PickMode()
{
string pick = Console.ReadLine();
if (pick == "easy")
{
Console.WriteLine("you have picked easy.");
}
}
}
這是我的 Player 課程
public class PLayer
{
public string Name;
private int _statValue;
private string _statName;
public bool Dead;
public PLayer(string statName, int statValue)
{
_statName = statName;
_statValue = statValue;
}
public override string ToString() => _statName + ": " + _statValue;
List<PLayer> stats = new List<PLayer>
{ new("Health", 100),
new("Accuracy", 89),
new("Blade Amount", 2),
new("Blade Damage", 20),
new("Blade Lanth", 40),
new("Blade durablity", 50),
new("Col", 0)
};
//all the NPCs you know.
List<string> Npc = new List<string> { "levi: ", "Armin: ", "Mikasa: ", "Hange: ", "Erwin: " };
//skills for killing titans
List<string> Skills = new List<string>();
public void PrintStats()
{
Console.WriteLine("your stats are: ");
foreach (var stat in stats)
{
Thread.Sleep(575);
Console.WriteLine(stat.ToString());
}
}
}
像“PLayer”這樣的名字(“P”和“L”都是大寫的)只是自找麻煩。
最佳實踐:使用PascalCase ,例如class Player
使用命名空間。 默認情況下,MSVS 會為您執行此操作(如果您使用的是 Visual Studio IDE); 您沒有向我們展示您是否自己使用它們。
在引用不同模塊中的對象時,使用命名空間可以很容易地限定類名。
主要問題:
如果您想要Player Playerstat = new Player()
... 那么您需要為“Player”聲明一個無參數(“無參數”)構造函數!
您的代碼有幾個問題,一個巨大的問題是Stack Overflow Error 。
如果您一開始就正確創建了PLayer
類,那么它的設計方式會導致無限循環。
首先,要修復您看到的錯誤,您需要將參數傳遞到PLayer
類的構造函數中。
在您的PLayer
類中,您有一個構造函數:
public PLayer(string statName, int statValue)
{
_statName = statName;
_statValue = statValue;
}
這要求您在實例化PLayer
時提供string
statName
和int
statValue
。
因此,在您的Program
中,您編寫的行如下:
PLayer Playerstat = new PLayer(); // I'm trying to reference the class Player here but it's not letting me.
需要改為:
PLayer Playerstat = new PLayer("ExampleString", 1); // I'm trying to reference the class Player here but it's not letting me.
但是,這不是這里的主要問題。 如果你最終解決了這個問題,你將去運行你的程序並遇到 Stack Overflow 錯誤。 原因是,您的PLayer
類在實例化時會嘗試使用您的代碼在自身內部創建一個List<PLayer>
:
List<PLayer> stats = new List<PLayer>
{ new("Health", 100),
new("Accuracy", 89),
new("Blade Amount", 2),
new("Blade Damage", 20),
new("Blade Lanth", 40),
new("Blade durablity", 50),
new("Col", 0)
};
您嘗試在此處創建的每個PLayer
也將嘗試創建自己的PLayers
列表,該列表無限重復,直到您的計算機崩潰。
如果您希望能夠創建一個帶有可以打印出來的自定義統計信息的PLayer
,也許可以嘗試使用包含統計信息的PLayer
類中的Dictionary
來接近它。 這樣,您可以向PLayer
構造函數提供Dictionary
以在實例化時填充它。
也許看起來像下面這樣的PLayer
類會更適合您的需求:
public class PLayer
{
public string Name;
public bool Dead;
public PLayer(string name = "", Dictionary<string, int> stats = null)
{
Name = name;
if (stats != null)
this.Stats = stats;
}
Dictionary<string, int> Stats = new Dictionary<string, int>
{
{ "Health", 100 },
{ "Accuracy", 89 },
{ "Blade Amount", 2},
{ "Blade Damage", 20 },
{ "Blade Lanth", 40 },
{ "Blade durablity", 50 },
{ "Col", 0}
};
//all the NPCs you know.
List<string> Npc = new List<string> { "levi: ", "Armin: ", "Mikasa: ", "Hange: ", "Erwin: " };
//skills for killing titans
List<string> Skills = new List<string>();
public void PrintStats()
{
Console.WriteLine("your stats are: ");
foreach (var stat in stats)
{
Thread.Sleep(575);
Console.WriteLine(stat.ToString());
}
}
}
然后你可以使用它:
class Program
{
static PLayer Playerstat;
static void Main()
{
Dictionary<string, int> CustomStats = new Dictionary<string, int>
{
{ "Health", 1000 },
{ "Accuracy", 5 },
{ "Blade Amount", 15},
{ "Blade Damage", 5 },
{ "Blade Lanth", 20 },
{ "Blade durablity", 100 },
{ "Col", 0}
};
// Here we actually provide parameters for the constructor
Playerstat = new PLayer("Example Player Name", CustomStats);
Console.WriteLine("please pick a mode");
PLayGame();
PickMode();
Console.ReadKey();
}
public static void PLayGame()
{
List<string> Options = new List<string> { "Easy mode: ", "med mode: ", "Hard mode", "super hard mode: " };
foreach(string ModePick in Options)
{
Thread.Sleep(570);
Console.WriteLine(ModePick);
}
}
private static void PickMode()
{
string pick = Console.ReadLine();
if (pick == "easy")
{
Console.WriteLine("you have picked easy.");
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.