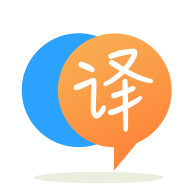
[英]Python pandas dataframe: Count number of elements a in column greater or smaller than a threshold
[英]Count number of elements greater than 1 in pandas dataframe
嗨,我希望你能幫我解決這個問題。 我有一個 dataframe df_test
import pandas as pd
import numpy as np
df_test = pd.DataFrame(data=[[np.nan,2,3,"male"],
[4,5,6,"female"],
[1,2,np.nan,"female"]],
columns=['a','b','c','sex'])
df_test
a b c sex
0 NaN 2 3.0 male
1 4.0 5 6.0 female
2 1.0 2 NaN female
對於性別列中的每個類別,我想計算 dataframe (a,b,c)
的每一列中大於 1 的值的數量。
df_results
a_count b_count c_count sex
0 NaN 1 1 male
1 2 2 1 female
您可以使用groupby
和 aggregate 來計算值:
df_test = pd.DataFrame(data=[[np.nan,2,3,"male"],
[4,5,6,"female"],
[1,2,np.nan,"female"]],
columns=['a','b','c','sex'])
df_test.groupby('sex', sort=False).agg(lambda x : len(x.dropna()>1))
這給了我們預期的 output:
a b c
sex
male 0 1 1
female 2 2 1
如果您完全希望這些值是 Nan,那么您可以這樣做
df_test.groupby('sex', sort=False).agg(lambda x : np.nan if len(x.dropna()) == 0 else len(x.dropna()))
a b c
sex
male NaN 1 1
female 2.0 2 1
由於該列包含 NaN 值,因此 pandas 進行了一些內部優化以在內部將int
轉換為float
。 因此,您可能必須將列顯式轉換為 int。
請糾正
columns=[['a','b','c','sex']]
並替換為
columns=['a','b','c','sex']
然后
pd.concat([df_test.sex, df_test.drop(columns=["sex"]) >= 1], axis=1).groupby("sex").sum().replace(0, np.nan).rename(columns=lambda x: x + "_count").reset_index()
檢查下面的代碼(根據示例數據,我假設所有值都大於或等於 1 或 nan)
pd.DataFrame(np.where(df_test.values == 1, np.nan, df_test.values), columns = df_test.columns).groupby(“sex”).count().reset_index()
讓我們試試:
(df_test.drop('sex', axis=1).ge(1) # compare the data with `1`
.groupby(df_test['sex'],sort=False).sum() # count the number of `True` with sum
.add_suffix('_count') # add the suffix
.reset_index() # make `sex` a column
)
Output:
sex a_count b_count c_count
0 male 0 1 1
1 female 2 2 1
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.