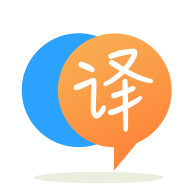
[英]Extract named group regex pattern from a compiled regex in Python
[英]Extract values from Regex Group
我有一個問題,我需要能夠從列表中的字符串中提取值。
我在 for 循環中使用 re 來匹配列表中每個字符串的組模式,並將其分配給一個變量,並使用 format 方法將其打印在一行上。
import re
inventory = ["shoes, 12, 29.99", "shirts, 20, 9.99", "sweatpants, 25, 15.00", "scarves, 13, 7.75"]
for items in inventory:
pattern = "(?P<product>[a-z]+),\s(?P<quantity>[0-9]+),\s(?P<price>[0-9]*\.[0-9]{2})"
details = re.match(pattern, items)
product = details.group('product')
quantity = details.group('quantity')
price = details.group('price')
print("The store has {} {}, each for {} USD".format(quantity, product, price))
這產生了我的結果
The store has 12 shoes, each for 29.99 USD
The store has 20 shirts, each for 9.99 USD
The store has 25 sweatpants, each for 15.00 USD
The store has 13 scarves, each for 7.75 USD
我想問是否可以使用details.groups
從元組中提取值並打印所需的結果或任何建議來改進/簡化它?
實際上,借助re.split
和f
字符串,我們可以使您的解決方案更加緊湊:
import re
inventory = ["shoes, 12, 29.99", "shirts, 20, 9.99", "sweatpants, 25, 15.00", "scarves, 13, 7.75"]
for inv in inventory:
product, quantity, price = re.split(r',\s*', inv)
print(f"The store has {quantity} {product}, each for {price} USD"
這打印:
The store has 12 shoes, each for 29.99 USD
The store has 20 shirts, each for 9.99 USD
The store has 25 sweatpants, each for 15.00 USD
The store has 13 scarves, each for 7.75 USD
您可以使用
print("The store has {quantity} {product}, each for {price} USD".format(**details.groupdict()))
請注意,占位符的命名方式與正則表達式命名的捕獲組相同。
以下代碼:
import re
inventory = ["shoes, 12, 29.99", "shirts, 20, 9.99", "sweatpants, 25, 15.00", "scarves, 13, 7.75"]
pattern = "(?P<product>[a-z]+),\s(?P<quantity>[0-9]+),\s(?P<price>[0-9]*\.[0-9]{2})"
for items in inventory:
details = re.match(pattern, items)
print("The store has {quantity} {product}, each for {price} USD".format(**details.groupdict()))
也打印
The store has 12 shoes, each for 29.99 USD
The store has 20 shirts, each for 9.99 USD
The store has 25 sweatpants, each for 15.00 USD
The store has 13 scarves, each for 7.75 USD
另請注意,該pattern
是在for
循環之外定義的,以獲得更好的性能。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.