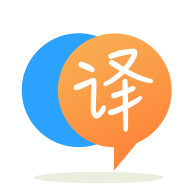
[英]ASP.NET CORE, How read all files in a folder that is used from another process
[英]How to save files to another folder in ASP.NET Core
我正在嘗試制定一種節省圖像的策略。 很簡單,用戶上傳一些圖像並將它們發送到服務器計算機上的文件夾中。
我使用代碼一次只保存用戶的一張圖片,並嘗試讓它與多張圖片一起使用。 結果如下:
這是代碼:
上傳模型.cs:
using Microsoft.AspNetCore.Http;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace SaveImagesTest.Models
{
public class UploadModel
{
public List<string> Name { get; set; }
public List<IFormFile> File { get; set; }
}
}
文件上傳控制器:
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using SaveImagesTest.Models;
using Microsoft.AspNetCore.Hosting;
using System.IO;
namespace SaveImagesTest.Controllers
{
public class FileUploadController : Controller
{
[Obsolete]
private IHostingEnvironment hostingEnv;
[Obsolete]
public FileUploadController(IHostingEnvironment env)
{
this.hostingEnv = env;
}
[HttpGet]
public IActionResult Upload()
{
return View();
}
[HttpPost]
[Obsolete]
public IActionResult Upload(UploadModel upload)
{
var FileDic = "Files";
string FilePath = Path.Combine(hostingEnv.WebRootPath, FileDic);
if (!Directory.Exists(FilePath))
Directory.CreateDirectory(FilePath);
int count = 0;
foreach (var file in upload.File)
{
var fileName = ++count;
var filePath = Path.Combine(FilePath, fileName.ToString());
using (FileStream fs = System.IO.File.Create(filePath))
{
file.CopyTo(fs);
continue;
}
}
return View("Index");
}
}
}
索引.cshtml:
@model SaveImagesTest.Models.UploadModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>ASP.NET Core save image to folder </title>
</head>
<body>
@using (Html.BeginForm("Upload", "FileUpload", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<table>
<tr>
<td>File Upload:</td>
<td>
<input type="file" id="File_Upload" name="File" onchange="readURL(this);" accept="image/*" multiple />
<br />
</td>
</tr>
<tr>
<td>File Name:</td>
<td>
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</td>
</tr>
<tr>
<td></td>
<td>
<input type="submit" value="Upload" class="btn-default" />
</td>
</tr>
</table>
}
</body>
</html>
以下是所發生情況的簡短摘要:我上傳了多個隨機文件,我看到所有圖像都在圖像列表中。 在 Upload function 中,它正在運行,但僅將第一個保存為 HEX。
嘗試使用IFormFile
提供的文件名:
[HttpPost]
[Obsolete]
public IActionResult Upload(UploadModel upload)
{
var FileDic = "Files";
string FilePath = Path.Combine(hostingEnv.WebRootPath, FileDic);
if (!Directory.Exists(FilePath))
Directory.CreateDirectory(FilePath);
foreach (var file in upload.File)
{
var filePath = Path.Combine(FilePath, file.FileName);
using (FileStream fs = System.IO.File.Create(filePath))
{
file.CopyTo(fs);
continue;
}
}
return View("Index");
}
由於IFormFile
包含帶有文件擴展名的文件名,因此打開這些文件不會有問題。
如果要使用序列號作為文件名,則需要從IFormFile
接口提供的文件名中提取文件擴展名:
[HttpPost]
public IActionResult Upload(UploadModel upload)
{
var FileDic = "Files";
string FilePath = Path.Combine(hostingEnv.WebRootPath, FileDic);
if (!Directory.Exists(FilePath))
Directory.CreateDirectory(FilePath);
int count = 0;
foreach (var file in upload.File)
{
var extension = Path.GetExtension(file.FileName);
var filePath = Path.Combine(FilePath, (++count).ToString()+extension);
using (FileStream fs = System.IO.File.Create(filePath))
{
file.CopyTo(fs);
continue;
}
}
return View("Index");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.