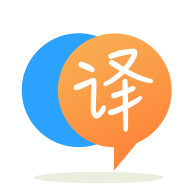
[英]OpenGL appears to be using (far too aggressive) frustum culling which I can't see how to modify
[英]OpenGL - Frustum not culling polygons beyond far plane
我已經實現了視錐剔除,並正在檢查邊界框與視錐平面的交集。 我添加了暫停視錐更新的功能,該功能使我可以查看視錐剔除是否已正常進行。 當我暫停后轉身時,在我身后和左右兩側都沒有任何東西呈現,它們逐漸變細,就像您期望的那樣。 超出剪輯距離(遠平面),它們仍會渲染,並且我不確定我的視錐更新或邊界框檢查代碼是否有問題,或者我使用的矩陣錯誤還是什么。 當我在投影矩陣中將距離設置為3000.0f時,它仍然說邊界框已經過了截錐體,但情況並非如此。
這是我創建模型視圖矩陣的地方:
projectionMatrix = glm::perspective(newFOV, 4.0f / 3.0f, 0.1f, 3000.0f);
viewMatrix = glm::mat4(1.0);
viewMatrix = glm::scale(viewMatrix, glm::vec3(1.0, 1.0, -1.0));
viewMatrix = glm::rotate(viewMatrix, anglePitch, glm::vec3(1.0, 0.0, 0.0));
viewMatrix = glm::rotate(viewMatrix, angleYaw, glm::vec3(0.0, 1.0, 0.0));
viewMatrix = glm::translate(viewMatrix, glm::vec3(-x, -y, -z));
modelViewProjectiomMatrix = projectionMatrix * viewMatrix;
之所以在Z方向上按-1縮放比例,是因為級別被設計為使用DirectX渲染,所以我將Z方向反轉。
這是我更新視錐的地方:
void CFrustum::calculateFrustum()
{
glm::mat4 mat = camera.getModelViewProjectionMatrix();
// Calculate the LEFT side
m_Frustum[LEFT][A] = (mat[0][3]) + (mat[0][0]);
m_Frustum[LEFT][B] = (mat[1][3]) + (mat[1][0]);
m_Frustum[LEFT][C] = (mat[2][3]) + (mat[2][0]);
m_Frustum[LEFT][D] = (mat[3][3]) + (mat[3][0]);
// Calculate the RIGHT side
m_Frustum[RIGHT][A] = (mat[0][3]) - (mat[0][0]);
m_Frustum[RIGHT][B] = (mat[1][3]) - (mat[1][0]);
m_Frustum[RIGHT][C] = (mat[2][3]) - (mat[2][0]);
m_Frustum[RIGHT][D] = (mat[3][3]) - (mat[3][0]);
// Calculate the TOP side
m_Frustum[TOP][A] = (mat[0][3]) - (mat[0][1]);
m_Frustum[TOP][B] = (mat[1][3]) - (mat[1][1]);
m_Frustum[TOP][C] = (mat[2][3]) - (mat[2][1]);
m_Frustum[TOP][D] = (mat[3][3]) - (mat[3][1]);
// Calculate the BOTTOM side
m_Frustum[BOTTOM][A] = (mat[0][3]) + (mat[0][1]);
m_Frustum[BOTTOM][B] = (mat[1][3]) + (mat[1][1]);
m_Frustum[BOTTOM][C] = (mat[2][3]) + (mat[2][1]);
m_Frustum[BOTTOM][D] = (mat[3][3]) + (mat[3][1]);
// Calculate the FRONT side
m_Frustum[FRONT][A] = (mat[0][3]) + (mat[0][2]);
m_Frustum[FRONT][B] = (mat[1][3]) + (mat[1][2]);
m_Frustum[FRONT][C] = (mat[2][3]) + (mat[2][2]);
m_Frustum[FRONT][D] = (mat[3][3]) + (mat[3][2]);
// Calculate the BACK side
m_Frustum[BACK][A] = (mat[0][3]) - (mat[0][2]);
m_Frustum[BACK][B] = (mat[1][3]) - (mat[1][2]);
m_Frustum[BACK][C] = (mat[2][3]) - (mat[2][2]);
m_Frustum[BACK][D] = (mat[3][3]) - (mat[3][2]);
// Normalize all the sides
NormalizePlane(m_Frustum, LEFT);
NormalizePlane(m_Frustum, RIGHT);
NormalizePlane(m_Frustum, TOP);
NormalizePlane(m_Frustum, BOTTOM);
NormalizePlane(m_Frustum, FRONT);
NormalizePlane(m_Frustum, BACK);
}
最后,在檢查邊框的地方:
bool CFrustum::BoxInFrustum( float x, float y, float z, float x2, float y2, float z2)
{
// Go through all of the corners of the box and check then again each plane
// in the frustum. If all of them are behind one of the planes, then it most
// like is not in the frustum.
for(int i = 0; i < 6; i++ )
{
if(m_Frustum[i][A] * x + m_Frustum[i][B] * y + m_Frustum[i][C] * z + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x2 + m_Frustum[i][B] * y + m_Frustum[i][C] * z + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x + m_Frustum[i][B] * y2 + m_Frustum[i][C] * z + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x2 + m_Frustum[i][B] * y2 + m_Frustum[i][C] * z + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x + m_Frustum[i][B] * y + m_Frustum[i][C] * z2 + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x2 + m_Frustum[i][B] * y + m_Frustum[i][C] * z2 + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x + m_Frustum[i][B] * y2 + m_Frustum[i][C] * z2 + m_Frustum[i][D] > 0) continue;
if(m_Frustum[i][A] * x2 + m_Frustum[i][B] * y2 + m_Frustum[i][C] * z2 + m_Frustum[i][D] > 0) continue;
// If we get here, it isn't in the frustum
return false;
}
// Return a true for the box being inside of the frustum
return true;
}
我注意到了幾件事,尤其是關於如何設置投影矩陣的事情。 對於初學者,除非您使用某種包裝器或怪異的api,否則gluProject不會返回值。 gluLookAt經常使用。
接下來,假設縮放,旋轉和平移功能旨在更改模型視圖矩陣,則需要顛倒它們的順序。 OpenGL實際上並沒有移動對象。 而是有效地移動原點,並使用新定義的<0,0,0>渲染每個對象。 因此,您可以“移動”到要渲染的位置,然后根據需要旋轉軸,然后拉伸網格。
至於裁剪問題,您可能希望給glClipPlane()一個好的外觀。 如果一切工作大多 ,但似乎有些舍入誤差,嘗試在你的觀點改變了近裁剪平面(,,,)函數從0.1到1.0(較小的值往往會亂用Z緩沖)。
我看到了許多不熟悉的語法,所以我認為您正在使用某種包裝器。 但是這是我使用的GL項目中的一些(Qt)代碼片段。 可能會幫助,鄧諾:
//This gets called during resize, as well as once during initialization
void GLWidget::resizeGL(int width, int height) {
int side = qMin(width, height);
padX = (width-side)/2.0;
padY = (height-side)/2.0;
glViewport(padX, padY, side, side);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(60.0, 1.0, 1.0, 2400.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
//This fragment gets called at the top of every paint event:
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glLightfv(GL_LIGHT0, GL_POSITION, FV0001);
camMain.stepVars();
gluLookAt(camMain.Pos[0],camMain.Pos[1],camMain.Pos[2],
camMain.Aim[0],camMain.Aim[1],camMain.Aim[2],
0.0,1.0,0.0);
glPolygonMode(GL_FRONT_AND_BACK, drawMode);
//And this fragment represents a typical draw event
void GLWidget::drawFleet(tFleet* tIn) {
if (tIn->firstShip != 0){
glPushMatrix();
glTranslatef(tIn->Pos[0], tIn->Pos[1], tIn->Pos[2]);
glRotatef(tIn->Yaw, 0.0, 1.0, 0.0);
glRotatef(tIn->Pitch,0,0,1);
drawShip(tIn->firstShip);
glPopMatrix();
}
}
我正在假設您是GL的新手,所以如果我有點腐,我深表歉意。
我有同樣的問題。
給定Vinny Rose的答案,我檢查了創建歸一化平面的函數,並發現了錯誤。
這是更正的版本,其中不正確的計算已被注釋掉:
plane plane_normalized(float A, float B, float C, float D) {
// Wrong, this is not a 4D vector
// float nf = 1.0f / sqrtf(A * A + B * B + C * C + D * D);
// Correct
float nf = 1.0f / sqrtf(A * A + B * B + C * C);
return (plane) {{
nf * A,
nf * B,
nf * C,
nf * D
}};
}
我的猜測是您的NormalizePlane
函數會執行類似的操作。
規范化的目的是使飛機具有Hessian范式,以便我們可以輕松進行半空間測試。 如果像對待四維矢量一樣對平面進行歸一化,則法線方向[A,B,C]仍然正確,但偏移D卻不正確。
我認為在針對頂部,底部,左側和右側的平面測試點時,您會獲得正確的結果,因為它們通過了原點,並且近平面可能足夠靠近而不會引起注意。 (邊界球測試將失敗。)
當我恢復正確的歸一化時,平截頭體剔除對我來說工作正常。
我想這是正在發生的事情:遠端平面的定義正確,但是在我的測試中,該平面的D值太小了。 因此,物體被接受為位於遠平面的正確一側,因為數學運算迫使遠平面實際上比您想要的遠得多。
嘗試不同的方法:(http://www.lighthouse3d.com/tutorials/view-frustum-culling/geometric-approach-extracting-the-planes/)
float tang = tanf(fov * PI / 360.0f);
float nh = near * tang; // near height
float nw = nh * aspect; // near width
float fh = far * tang; // far height
float fw = fh * aspect; // far width
glm::vec3 p,nc,fc,X,Y,Z,Xnw,Ynh;
//camera position
p = glm::vec3(viewMatrix[3][0],viewMatrix[3][1],viewMatrix[3][2]);
// the left vector
glm::vec3 X = glm::vec3(viewMatrix[0][0], viewMatrix[1][0], viewMatrix[2][0]);
// the up vector
glm::vec3 Y = glm::vec3(viewMatrix[0][1], viewMatrix[1][1], viewMatrix[2][1]);
// the look vector
glm::vec3 Z = glm::vec3(viewMatrix[0][2], viewMatrix[1][2], viewMatrix[2][2]);
nc = p - Z * near; // center of the near plane
fc = p - Z * far; // center of the far plane
// the distance to get to the left or right edge of the near plane from nc
Xnw = X * nw;
// the distance to get to top or bottom of the near plane from nc
Ynh = Y * nh;
// the distance to get to the left or right edge of the far plane from fc
Xfw = X * fw;
// the distance to get to top or bottom of the far plane from fc
Yfh = Y * fh;
ntl = nc + Ynh - Xnw; // "near top left"
ntr = nc + Ynh + Xnw; // "near top right" and so on
nbl = nc - Ynh - Xnw;
nbr = nc - Ynh + Xnw;
ftl = fc + Yfh - Xfw;
ftr = fc + Yfh + Xfw;
fbl = fc - Yfh - Xfw;
fbr = fc - Yfh + Xfw;
m_Frustum[TOP] = planeWithPoints(ntr,ntl,ftl);
m_Frustum[BOTTOM] = planeWithPoints(nbl,nbr,fbr);
m_Frustum[LEFT] = planeWithPoints(ntl,nbl,fbl);
m_Frustum[RIGHT] = planeWithPoints(nbr,ntr,fbr);
m_Frustum[FRONT] = planeWithPoints(ntl,ntr,nbr);
m_Frustum[BACK] = planeWithPoints(ftr,ftl,fbl);
// Normalize all the sides
NormalizePlane(m_Frustum, LEFT);
NormalizePlane(m_Frustum, RIGHT);
NormalizePlane(m_Frustum, TOP);
NormalizePlane(m_Frustum, BOTTOM);
NormalizePlane(m_Frustum, FRONT);
NormalizePlane(m_Frustum, BACK);
然后,planeWithPoints將類似於:
planeWithPoints(glm::vec3 a, glm::vec3 b, glm::vec3 c){
double A = a.y * (b.z - c.z) + b.y * (c.z - a.z) + c.y * (a.z - b.z);
double B = a.z * (b.x - c.x) + b.z * (c.x - a.x) + c.z * (a.x - b.x);
double C = a.x * (b.y - c.y) + b.x * (c.y - a.y) + c.x * (a.y - b.y);
double D = -(a.x * (b.y * c.z - c.y * b.z) + b.x * (c.y * a.z - a.y * c.z) + c.x * (a.y * b.z - b.y * a.z));
return glm::vec4(A,B,C,D);
}
我沒有測試任何上述內容。 但是如果需要,可以使用原始參考。
上一個答案: 當矩陣由2D數組表示時,OpenGL和GLSL矩陣以列優先順序存儲和訪問。 對於GLM,這也是正確的,因為它們遵循GLSL標准。
您需要將您的視錐創建更改為以下內容。
// Calculate the LEFT side (column1 + column4) m_Frustum[LEFT][A] = (mat[3][0]) + (mat[0][0]); m_Frustum[LEFT][B] = (mat[3][1]) + (mat[0][1]); m_Frustum[LEFT][C] = (mat[3][2]) + (mat[0][2]); m_Frustum[LEFT][D] = (mat[3][3]) + (mat[0][3]); // Calculate the RIGHT side (-column1 + column4) m_Frustum[RIGHT][A] = (mat[3][0]) - (mat[0][0]); m_Frustum[RIGHT][B] = (mat[3][1]) - (mat[0][1]); m_Frustum[RIGHT][C] = (mat[3][2]) - (mat[0][2]); m_Frustum[RIGHT][D] = (mat[3][3]) - (mat[0][3]); // Calculate the TOP side (-column2 + column4) m_Frustum[TOP][A] = (mat[3][0]) - (mat[1][0]); m_Frustum[TOP][B] = (mat[3][1]) - (mat[1][1]); m_Frustum[TOP][C] = (mat[3][2]) - (mat[1][2]); m_Frustum[TOP][D] = (mat[3][3]) - (mat[1][3]); // Calculate the BOTTOM side (column2 + column4) m_Frustum[BOTTOM][A] = (mat[3][0]) + (mat[1][0]); m_Frustum[BOTTOM][B] = (mat[3][1]) + (mat[1][1]); m_Frustum[BOTTOM][C] = (mat[3][2]) + (mat[1][2]); m_Frustum[BOTTOM][D] = (mat[3][3]) + (mat[1][3]); // Calculate the FRONT side (column3 + column4) m_Frustum[FRONT][A] = (mat[3][0]) + (mat[2][0]); m_Frustum[FRONT][B] = (mat[3][1]) + (mat[2][1]); m_Frustum[FRONT][C] = (mat[3][2]) + (mat[2][2]); m_Frustum[FRONT][D] = (mat[3][3]) + (mat[2][3]); // Calculate the BACK side (-column3 + column4) m_Frustum[BACK][A] = (mat[3][0]) - (mat[2][0]); m_Frustum[BACK][B] = (mat[3][1]) - (mat[2][1]); m_Frustum[BACK][C] = (mat[3][2]) - (mat[2][2]); m_Frustum[BACK][D] = (mat[3][3]) - (mat[2][3]);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.