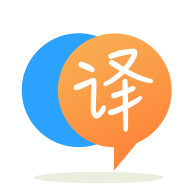
[英]How to add value to ComboBox's items, so I can choose it like this comboBox.SelectedIndex = myInt?
[英]How can I change all the form's text when I choose a language in combobox?
就像facebook改變它的語言一樣,它改變了所有..需要幫助來重建我的代碼。 我有3個表格和我的組合框,我以前選擇的語言是第一種形式。 當我在第一種形式中選擇組合框中的語言時,我希望我的其他兩種形式也會改變它的語言。
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
if (comboBox1.SelectedItem.ToString() == "English")
{
ChangeLanguage("en");
}
else if (comboBox1.SelectedItem.ToString() == "Spanish")
{
ChangeLanguage("es-ES");
}
else if (comboBox1.SelectedItem.ToString() == "French")
{
ChangeLanguage("fr-FR");
}
}
private void ChangeLanguage(string lang)
{
foreach (Control c in this.Controls)
{
ComponentResourceManager crm = new ComponentResourceManager(typeof(Form1));
crm.ApplyResources(c, c.Name, new CultureInfo(lang));
}
}
您可以使用包含“LanguageChanged”靜態事件的基類以及觸發它的函數。 如果您的所有表單都是該基類的派生類,則可以在其中處理該事件。 就像是:
public partial class Form1 : BaseLanguageForm
{
public Form1()
{
InitializeComponent();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
if (comboBox1.SelectedItem.ToString() == "English")
{
this.TriggerLanguageChange("en");
}
else if (comboBox1.SelectedItem.ToString() == "Spanish")
{
this.TriggerLanguageChange("es-ES");
}
else if (comboBox1.SelectedItem.ToString() == "French")
{
this.TriggerLanguageChange("fr-FR");
}
}
}
/// <summary>
/// The event that should be passed whenever language needs to be changed
/// </summary>
public class LanguageArgs:EventArgs
{
string _languageSymbol;
/// <summary>
/// Gets the language symble.
/// </summary>
public string LanguageSymbol
{
get { return _languageSymbol; }
}
/// <summary>
/// Initializes a new instance of the <see cref="LanguageArgs"/> class.
/// </summary>
/// <param name="symbol">The symbol.</param>
public LanguageArgs(string symbol)
{
this._languageSymbol = symbol;
}
}
/// <summary>
/// A base class that your class should derivative from
/// </summary>
public class BaseLanguageForm:Form
{
/// <summary>
/// Triggers the language change event.
/// </summary>
/// <param name="languageSymbol">The language symbol.</param>
protected void TriggerLanguageChange(string languageSymbol)
{
if (Form1.onLanguageChanged != null)
{
LanguageArgs args = new LanguageArgs(languageSymbol);
Form1.onLanguageChanged(this, args);
}
}
/// <summary>
/// This should be triggered whenever the combo box value chages
/// It is protected, just incase you want to do any thing else specific to form instacne type
/// </summary>
protected static event EventHandler<LanguageArgs> onLanguageChanged;
/// <summary>
/// This will be called from your form's constuctor
/// (you don't need to do anything, the base class constuctor is called automatically)
/// </summary>
public BaseLanguageForm()
{
//registering to the event
BaseLanguageForm.onLanguageChanged += new EventHandler<LanguageArgs>(BaseLanguageForm_onLanguageChanged);
}
/// <summary>
/// The function that was regidtered to the event
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="e">The e.</param>
void BaseLanguageForm_onLanguageChanged(object sender, LanguageArgs e)
{
string lang = e.LanguageSymbol;
foreach (Control c in this.Controls)
{
ComponentResourceManager crm = new ComponentResourceManager(typeof(Form1));
crm.ApplyResources(c, c.Name, new CultureInfo(lang));
}
}
}
基於我從你的問題中理解的內容,我認為如果你試圖從其他形式調用ChangeLanguage(string lang)
,這是可能的。
您需要在每個Form
添加一個Timer
,以便使用void和兩個變量。 第一個是bool
,它將用於檢測是否需要在其他TWO表單中調用ChangeLanguage
,第二個是用於指示語言的string
。 這兩個變量必須是public
和static
以便可以通過其他形式控制它們。
Form1.cs的
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Globalization;
namespace NameSpaceGoesHere
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
public static bool _ChangeLanguage = false; //This will indicate if the void needs to be called
public static string _Language = ""; //This will indicate our new language
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
ChangeLanguage("fr-FR");
}
private void ChangeLanguage(string lang)
{
foreach (Control c in this.Controls)
{
ComponentResourceManager crm = new ComponentResourceManager(typeof(Form1));
crm.ApplyResources(c, c.Name, new CultureInfo(lang));
_Language = lang; //Sets the language to lang
_ChangeLanguage = true; //Indicates that the void needs to be called through the TWO other forms as well
}
}
}
}
Form2.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Globalization;
namespace NameSpaceGoesHere
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
public static bool _ChangeLanguage = false; //Required for the third form
private void Form2_Load(object sender, EventArgs e)
{
Timer Timer1 = new Timer(); //Initialize a new Timer object
Timer1.Tick +=new EventHandler(Timer1_Tick); //Link its Tick event with Timer1_Tick
Timer1.Start(); //Start the timer
}
private void ChangeLanguage(string lang)
{
foreach (Control c in this.Controls)
{
ComponentResourceManager crm = new ComponentResourceManager(typeof(Form1));
crm.ApplyResources(c, c.Name, new CultureInfo(lang));
_ChangeLanguage = true;
}
}
private void Timer1_Tick(object sender, EventArgs e)
{
if (Form1._ChangeLanguage) //Indicates if ChangeLanguage() requires to be called
{
Form1._ChangeLanguage = false; //Indicates that we'll call the method
ChangeLanguage(Form1._Language); //Call the method
}
}
}
}
Form3.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Globalization;
namespace NameSpaceGoesHere
{
public partial class Form3 : Form
{
public Form3()
{
InitializeComponent();
}
private void Form3_Load(object sender, EventArgs e)
{
Timer Timer1 = new Timer(); //Initialize a new Timer object
Timer1.Tick += new EventHandler(Timer1_Tick); //Link its Tick event to Timer1_Tick
Timer1.Start(); //Start the timer
}
private void ChangeLanguage(string lang)
{
foreach (Control c in this.Controls)
{
ComponentResourceManager crm = new ComponentResourceManager(typeof(Form1));
crm.ApplyResources(c, c.Name, new CultureInfo(lang));
}
}
private void Timer1_Tick(object sender, EventArgs e)
{
if (Form2._ChangeLanguage) //Indicates if ChangeLanguage() requires to be called
{
Form2._ChangeLanguage = false; //Indicates that we'll call the method
ChangeLanguage(Form1._Language); //Call the method
}
}
}
}
謝謝,
我希望這個對你有用 :)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.