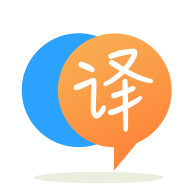
[英]How can I Transfer my Score Game Object from my game scene to game over scene in Unity
[英]How can i convert a game with multiple scene into a single scene game?
我制作了一个统一游戏,其中有多个不同级别的场景,其中用户必须 select 一个彩色块到下一个级别 go 但我希望我的代码在一个场景中有没有办法可以转换我的多场景游戏成一个单一的场景。 一级网格基础的代码是
public IEnumerator Start() {
grid = new GameObject[ySize, xSize];
float x = xStart;
float y = yStart;
ScreenRandom = Random.Range(0, 3);
if (ScreenRandom == 0)
{
img.color = UnityEngine.Color.red;
text.text = "Select all the Red Objects";
yield return new WaitForSeconds(time);
Destroy(img);
Destroy(text);
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 1)
{
GameObject rblock = Instantiate(RedPrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountRed++;
}
else {
GameObject block = Instantiate(NonRedPrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
if (ScreenRandom == 1)
{
img.color = UnityEngine.Color.blue;
text.text = "Select all the Blue Objects";
yield return new WaitForSeconds(time);
Destroy(img);
Destroy(text);
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 1)
{
GameObject rblock = Instantiate(BluePrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountBlue++;
}
else {
GameObject block = Instantiate(NonBluePrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
if (ScreenRandom == 2)
{
img.color = UnityEngine.Color.yellow;
text.text = "Select all the Yellow Objects";
yield return new WaitForSeconds(time);
Destroy(img);
Destroy(text);
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 1)
{
GameObject rblock = Instantiate(YellowPrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountYellow++;
}
else {
GameObject block = Instantiate(NonYellowPrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
}
检查和加载级别 2 的代码是:
void Update () {
screen = GridControl.ScreenRandom;
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (screen == 0)
{
if (Physics.Raycast(ray, out hit))
{
if (hit.collider.tag == "BlueBlock")
{
Destroy(hit.transform.gameObject);
print("GameOver");
Application.LoadLevel(0);
}
if (hit.collider.tag == "RedBlock")
{
RedCount++;
Destroy(hit.transform.gameObject);
Correct++;
Score.text = " " + Correct;
if (RedCount == GridControl.CountRed)
{
print("Next Level");
Application.LoadLevel(3);
}
}
if (hit.collider.tag == "YellowBlock")
{
Destroy(hit.transform.gameObject);
print("GameOver");
Application.LoadLevel(0);
}
}
}
if (screen == 1)
{
if (Physics.Raycast(ray, out hit))
{
if (hit.collider.tag == "BlueBlock")
{
BlueCount++;
Destroy(hit.transform.gameObject);
Correct++;
Score.text = " " + Correct;
if (BlueCount == GridControl.CountBlue)
{
print("Next Level");
Application.LoadLevel(3);
}
}
if (hit.collider.tag == "RedBlock")
{
Destroy(hit.transform.gameObject);
print("GameOver");
Application.LoadLevel(0);
}
if (hit.collider.tag == "YellowBlock")
{
Destroy(hit.transform.gameObject);
print("GameOver");
Application.LoadLevel(0);
}
}
}
if (screen == 2)
{
if (Physics.Raycast(ray, out hit))
{
if (hit.collider.tag == "BlueBlock")
{
Destroy(hit.transform.gameObject);
print("GameOver");
Application.LoadLevel(0);
}
if (hit.collider.tag == "RedBlock")
{
Destroy(hit.transform.gameObject);
print("Game Over");
Application.LoadLevel(0);
}
if (hit.collider.tag == "YellowBlock")
{
YellowCount++;
Destroy(hit.transform.gameObject);
Correct++;
Score.text = " " + Correct;
if (YellowCount == GridControl.CountYellow)
{
print("Next Level");
Application.LoadLevel(3);
}
}
}
2 级 GridBase 的代码是;
public void Start()
{
grid = new GameObject[ySize, xSize];
ScreenRandom = GridControl.ScreenRandom;
float x = xStart;
float y = yStart;
if (ScreenRandom == 0)
{
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 2)
{
GameObject rblock = Instantiate(RedPrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountRed++;
}
else {
GameObject block = Instantiate(NonRedPrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
if (ScreenRandom == 1)
{
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 2)
{
GameObject rblock = Instantiate(BluePrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountBlue++;
}
else {
GameObject block = Instantiate(NonBluePrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
if (ScreenRandom == 2)
{
int check = 1;
for (int i = 0; i < ySize; i++)
{
for (int j = 0; j < xSize; j++)
{
if (check <= 2)
{
GameObject rblock = Instantiate(YellowPrefabs[Random.Range(0, 1)]) as GameObject;
rblock.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
rblock.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
rblock.transform.SetParent(canvas.transform);
grid[i, j] = rblock;
CountYellow++;
}
else {
GameObject block = Instantiate(NonYellowPrefab[Random.Range(0, 2)]) as GameObject;
block.GetComponent<RectTransform>().anchoredPosition = new Vector2(x, y);
block.transform.localScale = new Vector3(1.0f, 1.0f, 1.0f) * scale;
block.transform.SetParent(canvas.transform);
grid[i, j] = block;
}
check++;
x += xWidth * space;
}
y -= yHeight * space;
x = xStart;
}
}
}
首先我不知道你为什么要那样做。 从技术上讲,这就是拥有场景的全部意义所在。 但是您仍然可以做的是将所有内容(1 级和 2 级)放在一个场景中,然后在摄像机外定位和禁用属于 2 级的任何内容。 然后,我将调用一个函数,而不是调用Application.LoadLevel
该函数会将属于 Level 1 的所有内容移出屏幕,同时将 Level 2 移入屏幕。 如果我将一个级别的所有对象分组为另一个 GameObject(可能称为 LevelX)的子级,这样可以更轻松地一次移动所有对象。 这甚至可以让您在级别之间的过渡中获得漂亮的幻灯片。 如果您不想要过渡,只需立即更改位置。
请小心,因为根据级别的数量,您可能会遇到严重的性能问题。
您可以为彩色块使用预制件并将其拖到不同级别的场景中。 如果您现在将行为脚本(管理光线投射到它时发生的情况)附加到您的预制件,则所有级别中的所有实例都将具有相同的逻辑。 这样您就可以为不同级别重用相同的代码库。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.