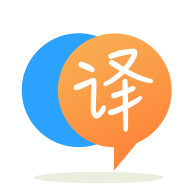
[英]Plot and return points of Intersection between two curves plotted from dataframes
[英]How to find the intersection points between two plotted curves in matplotlib?
这是我的方法。 我首先创建了两条测试曲线,仅使用 12 个样本点,只是为了说明这个概念。 创建具有采样点的阵列后,曲线的精确方程将丢失。
然后,搜索两条曲线之间的交点。 通过逐点遍历数组,并检查一条曲线何时从另一条曲线从另一条曲线向下移动到另一条曲线上方(或反向),可以通过求解线性方程来计算交点。
然后绘制交点以直观地检查结果。
import numpy as np
from matplotlib import pyplot as plt
N = 12
t = np.linspace(0, 50, N)
curve1 = np.sin(t*.08+1.4)*np.random.uniform(0.5, 0.9) + 1
curve2 = -np.cos(t*.07+.1)*np.random.uniform(0.7, 1.0) + 1
# note that from now on, we don't have the exact formula of the curves, as we didn't save the random numbers
# we only have the points correspondent to the given t values
fig, ax = plt.subplots()
ax.plot(t, curve1,'b-')
ax.plot(t, curve1,'bo')
ax.plot(t, curve2,'r-')
ax.plot(t, curve2,'ro')
intersections = []
prev_dif = 0
t0, prev_c1, prev_c2 = None, None, None
for t1, c1, c2 in zip(t, curve1, curve2):
new_dif = c2 - c1
if np.abs(new_dif) < 1e-12: # found an exact zero, this is very unprobable
intersections.append((t1, c1))
elif new_dif * prev_dif < 0: # the function changed signs between this point and the previous
# do a linear interpolation to find the t between t0 and t1 where the curves would be equal
# this is the intersection between the line [(t0, prev_c1), (t1, c1)] and the line [(t0, prev_c2), (t1, c2)]
# because of the sign change, we know that there is an intersection between t0 and t1
denom = prev_dif - new_dif
intersections.append(((-new_dif*t0 + prev_dif*t1) / denom, (c1*prev_c2 - c2*prev_c1) / denom))
t0, prev_c1, prev_c2, prev_dif = t1, c1, c2, new_dif
print(intersections)
ax.plot(*zip(*intersections), 'go', alpha=0.7, ms=10)
plt.show()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.