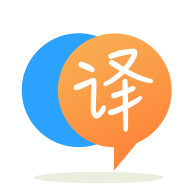
[英]For a given integer a, find all unique combinations of positive integers that sum up to a
[英]Efficient algorithm to compute combinations with repetitions of an array adding up to given sum
因此,在个人 C++ 项目中,我遇到了一个问题。 我将其改写如下:
给定一个包含n 个元素的数组(例如 [1, 3, 5],其中 n = 3 个元素),其中第i个 position 处的数字表示第i个索引处的数字可以取多少个可能值(例如,这里的第一个元素可以取 1 个值,即 0;第二个元素可以取 0,1,2 中的 3 个值;第三个元素可以取 0,1,2,3,4 中的 5 个值)。
我需要列出所有可能的 arrays 长度n总和小于或等于给定数字k 。 这是一个例子:
输入 1 :
输入数组 = [2,2]; k = 2
Output 1 :
[0,0], [0,1], [1,0], [1,1]
另外,例如:
输入 2 :
输入数组 = [2,2]; k = 1
Output 2 :
[0,0], [0,1], [1,0]
问题:
我编写了一个简单的递归和一个简单的迭代解决方案,它枚举了所有 arrays 并且只保留总和小于 k 的那些。 这些问题在于,对于n 很大且 k = 1的情况,我的代码需要很长时间才能运行,因为它枚举了所有情况并保留了一些情况。
我看不到任何重叠的子问题,所以我觉得 DP 和 memoization 不适用。 我怎样才能为此工作编写所需的 C++ 代码?
这是我的迭代版本代码:
// enumerates all arrays which sum up to k
vector<vector<int> > count_all_arrays(vector<int> input_array, int k){
vector<vector<int> > arr;
int n = (int)input_array.size();
// make auxilliary array with elements
for(int i = 0; i < n; i++){
vector<int> temp(input_array[i]);
std::iota(temp.begin(), temp.end(), 0);
arr.push_back(temp);
}
// computes combinations
vector<int> temp(n);
vector<vector<int> > answers;
vector<int> indices(n, 0);
int next;
while(1){
temp.clear();
for (int i = 0; i < n; i++)
temp.push_back(arr[i][indices[i]]);
long long int total = accumulate(temp.begin(), temp.end(), 0);
if(total <= k)
answers.push_back(temp);
next = n - 1;
while (next >= 0 &&
(indices[next] + 1 >= (int)arr[next].size()))
next--;
if (next < 0)
break;
indices[next]++;
for (int i = next + 1; i < n; i++)
indices[i] = 0;
}
return answers;
}
这是一个非常简单的递归任务:
#include <bits/stdc++.h>
using namespace std;
int arr[] = {2, 2};
int n = 2;
int k = 2;
void gen(int pos, int sum, string s){
if(pos == n){
cout<<"["<<s<<" ]"<<endl;
return;
}
for(int i = 0; i < arr[pos]; i++){
if(sum + i > k) return;
gen(pos + 1, sum + i, s + " " + to_string(i));
}
}
int main(){
gen(0, 0, "");
return 0;
}
只需为数组的每个插槽生成所有可能性,对于每个选择,将总和用于评估下一个插槽。
当n
很大且k = 1
时,很自然需要 O(n),因为您将拥有:
[0, 0, 0, ..., 0, 0, 1]
[0, 0, 0, ..., 0, 1, 0]
[0, 0, 0, ..., 1, 0, 0]
...
[0, 0, 1, ..., 0, 0, 0]
[0, 1, 0, ..., 0, 0, 0]
[1, 0, 0, ..., 0, 0, 0]
您应该使用 dp 使其在任何情况下都快速运行。 使用 dp[i][j] 表示您使用第一个 j 元素创建小于或等于 i 的总和的次数。
dp[i][j] = dp[
for (int l = 0; l <= i; l++)
dp[i][j] += dp[l][j] + min(i-l+1, input[j])
结果是 dp[k,n]
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.