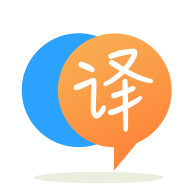
[英]Find the largest palindrome made from the product of two 3-digit numbers, just a small edit needed
[英]Find the largest palindrome made from the product of two 3-digit numbers using numpy
当我尝试使用 numpy package 解决这个问题时,我陷入了这个问题。我的想法是,我将乘以并保留我对 100 到 999 之间的 3 位数字所做的所有计算的列表,然后检查列表以查看哪些是回文并保存它们。 最后,我将对列表进行排序并获得最大的回文。 下面的代码显示了我试图做的事情。
import numpy as np
def void():
list1 = np.array(range(100,999))
list2 = np.array(range(100,999))
k = []
for i,j in zip(list1,list2):
k.append(np.multiply(list1,list2))
b = []
for x in range(0,len(k)):
if(reverseNum(k[x])==k[x]):
b.append(k[x])
print(b)
print(b[-1])
def reverseNum(num):
rev = 0
while(num>0):
rem = num % 10
rev = (rev*10) +rem
num = num // 10
return rev
void()
但是,当我尝试检查列表中的数字是否为回文时,出现以下错误:
Traceback (most recent call last):
File "main.py", line 40, in <module>
void()
File "main.py", line 22, in void
if(reverseNum(k[x]),k[x]):
File "main.py", line 31, in reverseNum
while(num>0):
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
这是否意味着无法使用 numpy 作为解决此问题的方法? 如果是,我哪里错了?
编辑:到目前为止我已经尝试过的(因为它被问到):根据错误消息,我尝试使用np.equal
和np.greater
而不是检查if(reverseNum(k[x])==k[x])
和num>0
但它给出了相同的错误。
假设结果有六位数字的 NumPy 方式(它不能有更多,因为 999 2是 998001):
import numpy as np
v = np.arange(100, 1000) # the range of three-digit numbers
a = np.outer(v, v) # all the products
print(a[(a // 100000 == a % 10) & # first digit == sixth digit
(a // 10000 % 10 == a // 10 % 10) &
(a // 1000 % 10 == a // 100 % 10)].max())
打印906609
。
使用纯 Python 进行双重检查:
>>> max(x*y
for x in range(100, 1000)
for y in range(100, 1000)
if str(x*y) == str(x*y)[::-1])
906609
您的问题源于您的线路,包括zip
。 我下面的代码并不漂亮,但试图松散地遵循您的方法。
import numpy as np
def void():
list1 = np.array(range(100,1000)) # you want to include '999'
list2 = np.array(range(100,1000))
k = []
for i,j in zip(list1,list2):
k.append(np.multiply(list1,j))
b = []
for r, row in enumerate(k):
for c, cell in enumerate(row):
if reverseNum(cell)==cell:
b.append(cell)
print(b)
print(max(b))
def reverseNum(num):
rev = 0
while(num>0):
rem = num % 10
rev = (rev*10) +rem
num = num // 10
return rev
void()
为什么它必须使用 numpy?
# test if palindrome based on str
def is_palindrome(number: int):
converted_to_string = str(number)
return converted_to_string == converted_to_string[::-1]
# product of two three-digit numbers
you_right = []
values = []
for x in range(999, 99, -1):
for y in range(999, 99, -1):
product = x*y
if is_palindrome(product):
values.append((x, y))
you_right.append(product)
winner = you_right.index(max(you_right))
print(values[winner])
# output
(993, 913)
另一个真正的 NumPy 解决方案,使用您的方式反转数字(主要通过使用错误消息中建议的.any()
修复它,您固执地拒绝尝试)。
v = np.arange(100, 1000)
a = np.outer(v, v)
num = a.copy()
rev = num * 0
while (m := num > 0).any():
rev[m] = rev[m] * 10 + num[m] % 10
num[m] //= 10
print(a[rev == a].max())
没有掩码m
你会得到相同的结果 (906609),但它更安全。 否则五位数的乘积不能正确反转,比如 101*102=10302 变成 203010 而不是 20301。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.